はじめに
前回はFlutter Widget of the Weekの「#52 ListView」、「#53 ListTile」、「#54 Container」を紹介しました。
今回はその続きで「#55 SelectableText」、「#56 DataTable」、「#57 Slider,RangeSlider,CupertinoSlider」の3つです。
前回の記事はこちら
Flutter Widget of the Week
環境
- Flutter 3.0.5
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#55 SelectableText
SelectableTextとは
その名の通り、選択できるテキストの際に利用するウィジェットです。
通常のTextウィジェットではコピー等するための選択ができませんがSelectableTextではそれができるようになります。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage055 extends StatelessWidget {
const SamplePage055({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('SelectableText'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: const [
Text('選択できないテキスト'),
SizedBox(height: 100),
SelectableText('選択できるテキスト'),
],
),
),
),
);
}
}
結果
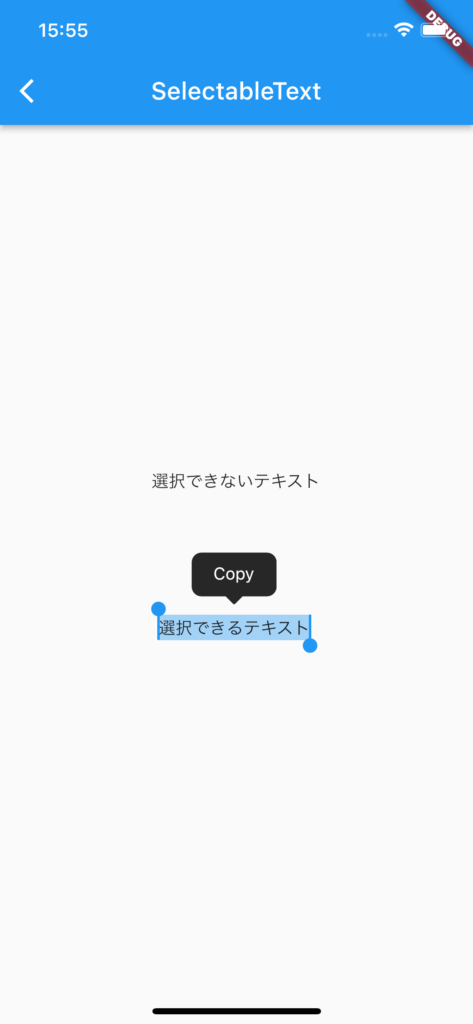
動画
公式リファレンス
SelectableText class - material library - Dart API
API docs for the SelectableText class from the material library, for the Dart programming language.
#56 DataTable
DataTableとは
エクセルのようなテーブル表示をさせたい場合に使うウィジェットです。
セルの内容に応じて列のサイズを自動調整してくれたりします。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage056 extends StatelessWidget {
const SamplePage056({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('DataTable'),
centerTitle: true,
),
body: SafeArea(
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: DataTable(
sortColumnIndex: 1,
columns: const [
DataColumn(
label: Text('年'),
numeric: true,
),
DataColumn(
label: Text('試合'),
),
DataColumn(
label: Text('打席'),
),
DataColumn(
label: Text('打率'),
),
DataColumn(
label: Text('本塁打'),
),
],
rows: const [
DataRow(
cells: [
DataCell(
Text('2021'),
),
DataCell(
Text('155'),
),
DataCell(
Text('639'),
),
DataCell(
Text('0.257'),
),
DataCell(
Text('46'),
),
],
),
DataRow(
cells: [
DataCell(
Text('2020'),
),
DataCell(
Text('44'),
),
DataCell(
Text('175'),
),
DataCell(
Text('0.190'),
),
DataCell(
Text('7'),
),
],
),
],
),
),
),
);
}
}
結果
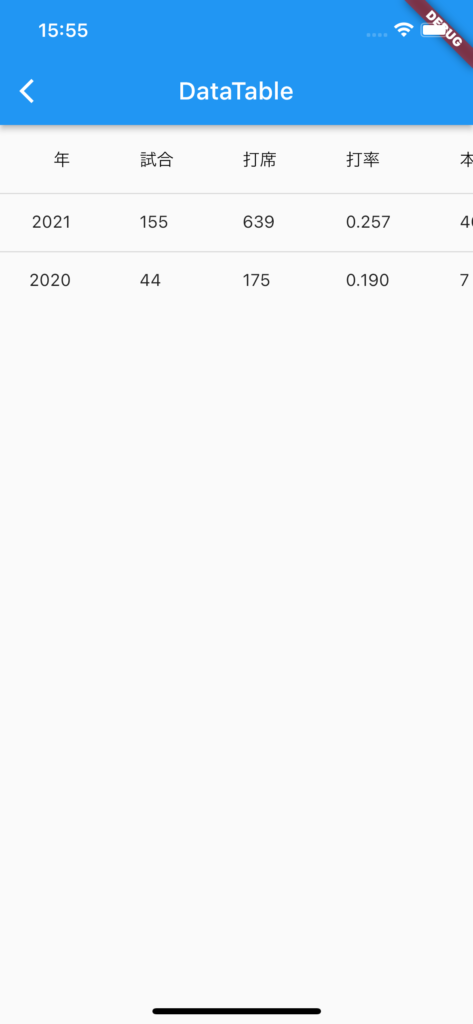
動画
公式リファレンス
DataTable class - material library - Dart API
API docs for the DataTable class from the material library, for the Dart programming language.
#57 Slider,RangeSlider,CupertinoSlider
Slider,RangeSlider,CupertinoSliderとは
ある範囲から値を選択できるスライダーが実装できるのがSliderウィジェットです。
RangeSliderは最初と最後の範囲が決められるスライダーで、CupertinoSliderはiOSデザインのスライダーが実装できます。
サンプルコード
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class SamplePage057 extends StatefulWidget {
const SamplePage057({
super.key,
});
@override
State<SamplePage057> createState() => _SamplePage057State();
}
class _SamplePage057State extends State<SamplePage057> {
double _sliderValue = 50;
RangeValues _rangeValues = const RangeValues(25, 75);
double _cupertinoSliderValue = 50;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Slider & RangeSlider & CupertinoSlider'),
centerTitle: true,
),
body: SafeArea(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text('Slider'),
Slider(
value: _sliderValue,
max: 100,
onChanged: (value) {
setState(() {
_sliderValue = value;
});
},
divisions: 100,
label: '${_sliderValue.toInt()}',
),
const SizedBox(height: 20),
const Text('RangeSlider'),
RangeSlider(
values: _rangeValues,
max: 100,
onChanged: (values) {
setState(() {
_rangeValues = values;
});
},
divisions: 100,
labels: RangeLabels(
'${_rangeValues.start.toInt()}',
'${_rangeValues.end.toInt()}',
),
),
const SizedBox(height: 20),
const Text('CupertinoSlider'),
CupertinoSlider(
value: _cupertinoSliderValue,
max: 100,
onChanged: (value) {
setState(() {
_cupertinoSliderValue = value;
});
},
divisions: 100,
),
],
),
),
);
}
}
結果
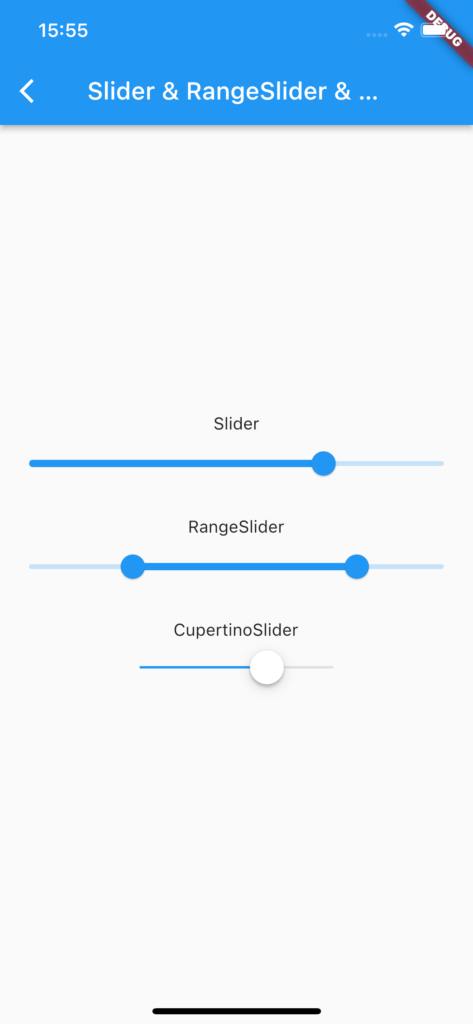
動画
公式リファレンス
Slider class - material library - Dart API
API docs for the Slider class from the material library, for the Dart programming language.
RangeSlider class - material library - Dart API
API docs for the RangeSlider class from the material library, for the Dart programming language.
CupertinoSlider class - cupertino library - Dart API
API docs for the CupertinoSlider class from the cupertino library, for the Dart programming language.
さいごに
SelectableTextは今まで使う機会がなかったので覚えておきたいです。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント