はじめに
前回はFlutter Widget of the Weekの「#97 ExpansionPanel」、「#98 Scrollbar」、「#99 connectivity_plus」を紹介しました。
今回はその続きで「#100 FlutterLogo」、「#101 animated_text_kit」、「#102 MouseRegion」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.3.7
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#100 FlutterLogo
FlutterLogoとは
名前の通り、Flutterのロゴが簡単に表示できるウィジェットです。
正直、使い所はあんまりないですがとりあえずサンプルとして表示する際に便利です。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage100 extends StatefulWidget {
const SamplePage100({
super.key,
});
@override
State<SamplePage100> createState() => _SamplePage100State();
}
class _SamplePage100State extends State<SamplePage100> {
bool isStacked = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('FlutterLogo'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const FlutterLogo(
size: 128,
),
const FlutterLogo(
size: 128,
style: FlutterLogoStyle.horizontal,
),
const FlutterLogo(
size: 128,
style: FlutterLogoStyle.stacked,
),
FlutterLogo(
size: 128,
style: isStacked
? FlutterLogoStyle.stacked
: FlutterLogoStyle.horizontal,
),
],
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
isStacked = !isStacked;
});
},
child: const Icon(Icons.replay_outlined),
),
);
}
}
結果
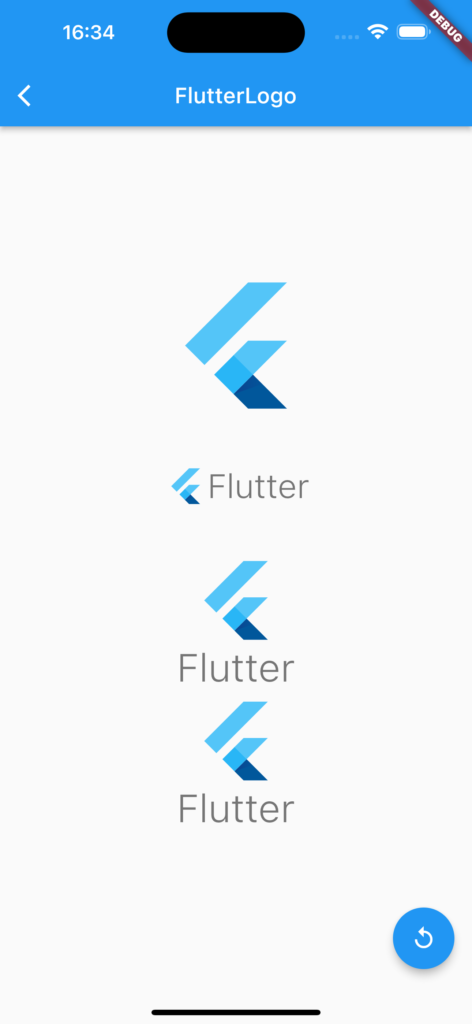
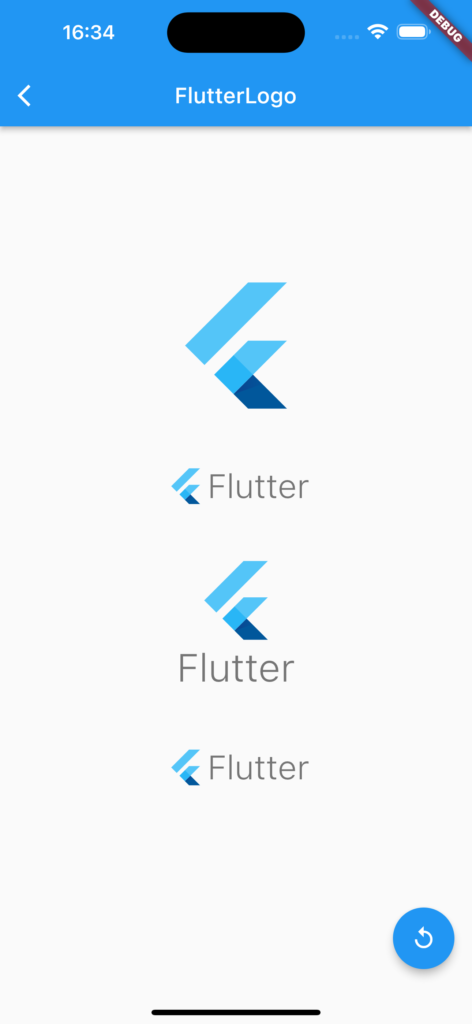
動画
公式リファレンス
FlutterLogo class - material library - Dart API
API docs for the FlutterLogo class from the material library, for the Dart programming language.
#101 animated_text_kit
animated_text_kitとは
簡単にテキストにアニメーションを追加できるパッケージです。
サンプルコード
import 'package:animated_text_kit/animated_text_kit.dart';
import 'package:flutter/material.dart';
class SamplePage101 extends StatelessWidget {
const SamplePage101({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('animated_text_kit'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
AnimatedTextKit(
animatedTexts: [
TyperAnimatedText('Android'),
TyperAnimatedText('iOS'),
TyperAnimatedText('Web'),
RotateAnimatedText('Linux'),
RotateAnimatedText('Windows'),
ScaleAnimatedText('Mac'),
ColorizeAnimatedText(
'LARRY PAGE',
textStyle: const TextStyle(
fontSize: 50,
),
colors: [
Colors.purple,
Colors.blue,
Colors.yellow,
Colors.red,
],
)
],
),
],
),
),
),
);
}
}
結果
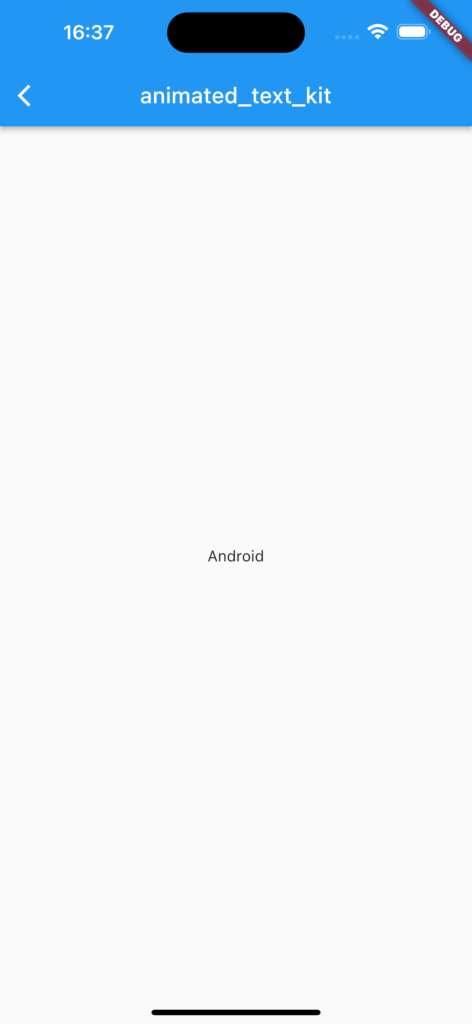
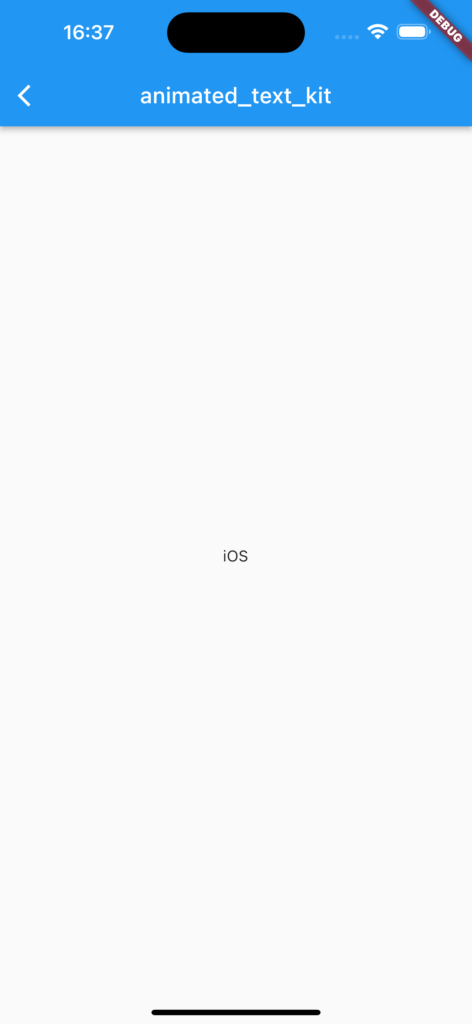
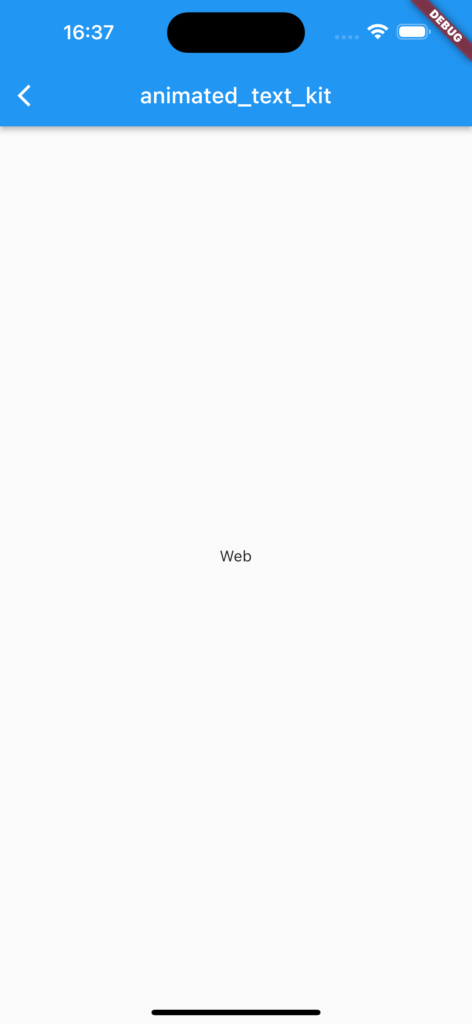
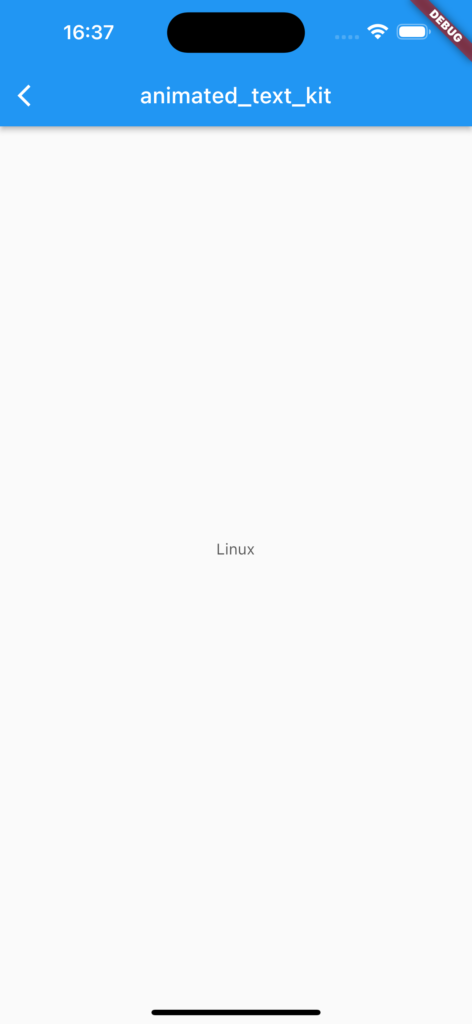
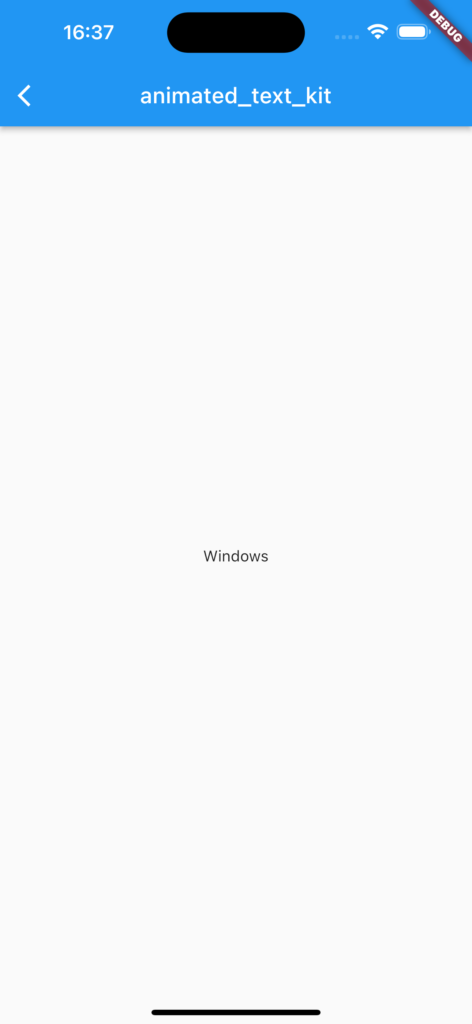
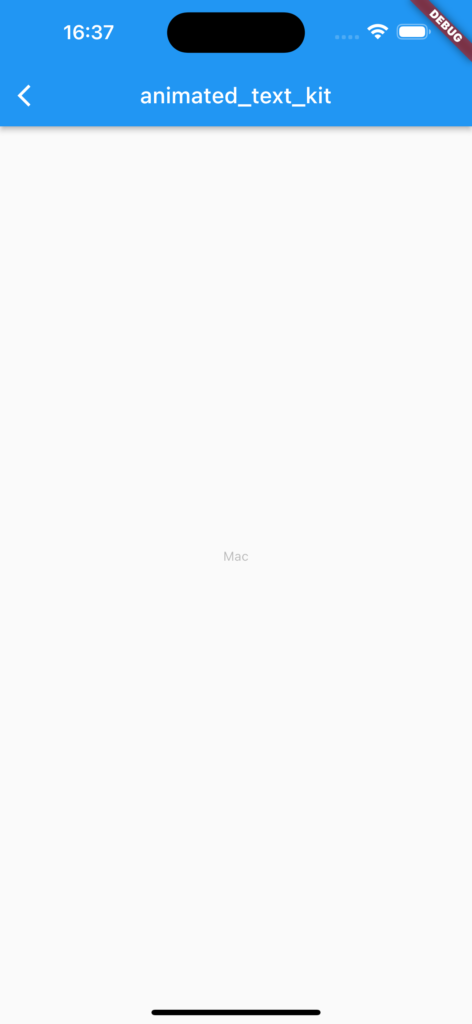
動画
公式リファレンス
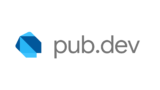
animated_text_kit | Flutter Package
A flutter package project which contains a collection of cool and beautiful text animations.
#102 MouseRegion
MouseRegionとは
マウスカーソルが特定のエリアにいることを検知できるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage102 extends StatelessWidget {
const SamplePage102({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('MouseRegion'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: MouseRegion(
cursor: SystemMouseCursors.text,
child: Container(
width: 128,
height: 128,
color: Colors.blue,
),
onEnter: (event) {
debugPrint('onEnter ${event.toString()}');
},
onHover: (event) {
debugPrint('onHover ${event.toString()}');
},
onExit: (event) {
debugPrint('onExit ${event.toString()}');
},
),
),
),
);
}
}
結果
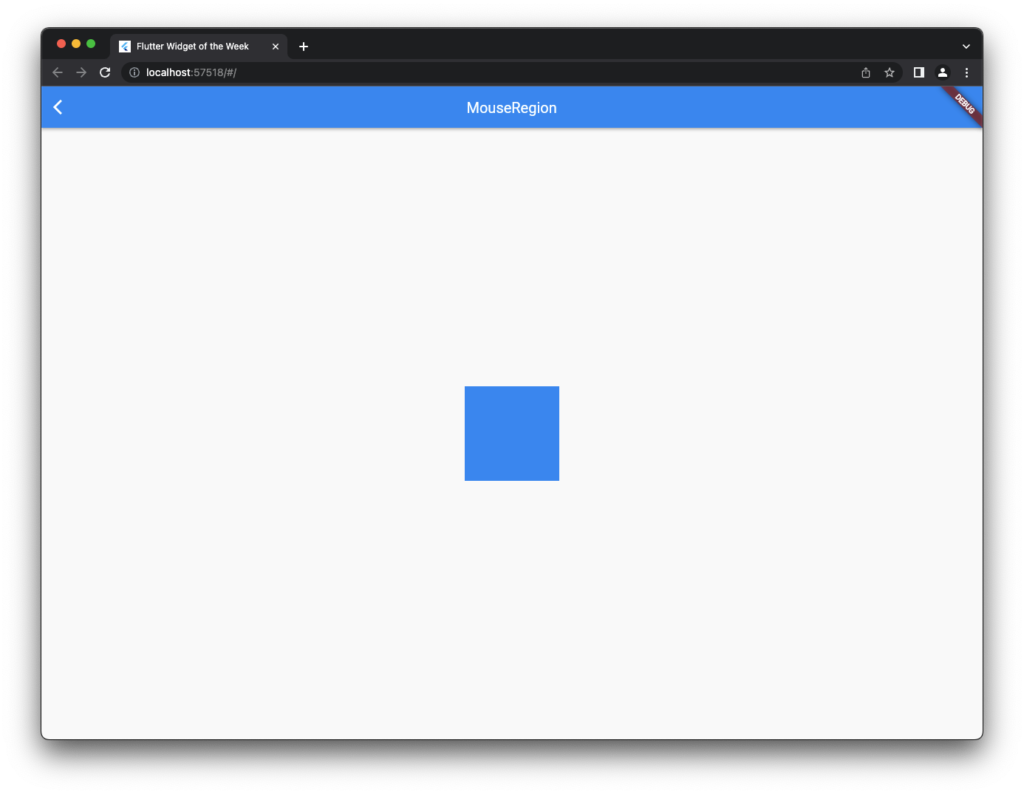
青い四角内にマウスカーソルを入れて、出したときのログ
onEnter _TransformedPointerEnterEvent#71090(position: Offset(614.2, 533.8))
onHover _TransformedPointerHoverEvent#3b457(position: Offset(614.2, 533.8))
onHover _TransformedPointerHoverEvent#4fb04(position: Offset(615.3, 528.0))
onHover _TransformedPointerHoverEvent#c1a2a(position: Offset(617.9, 520.2))
onHover _TransformedPointerHoverEvent#9d80c(position: Offset(619.1, 514.4))
onHover _TransformedPointerHoverEvent#c93f0(position: Offset(621.4, 508.6))
onHover _TransformedPointerHoverEvent#82150(position: Offset(622.6, 502.8))
onHover _TransformedPointerHoverEvent#17608(position: Offset(623.2, 501.0))
onHover _TransformedPointerHoverEvent#cc408(position: Offset(625.1, 497.2))
onHover _TransformedPointerHoverEvent#7c75d(position: Offset(625.7, 495.4))
onHover _TransformedPointerHoverEvent#58b17(position: Offset(626.3, 493.6))
onHover _TransformedPointerHoverEvent#9eae9(position: Offset(626.3, 493.2))
onHover _TransformedPointerHoverEvent#653d0(position: Offset(626.3, 492.8))
onHover _TransformedPointerHoverEvent#2abb4(position: Offset(626.7, 494.4))
onHover _TransformedPointerHoverEvent#300db(position: Offset(626.7, 508.2))
onHover _TransformedPointerHoverEvent#30edc(position: Offset(625.0, 528.0))
onExit _TransformedPointerExitEvent#cbb59(position: Offset(621.5, 555.8))
動画
公式リファレンス
MouseRegion class - widgets library - Dart API
API docs for the MouseRegion class from the widgets library, for the Dart programming language.
さいごに
このシリーズ、終りが見えない。。。
そして追いつく前にYouTubeのFlutter Widget of the Weekが終わってしまい、悲しみ。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント