はじめに
前回はFlutter Widget of the Weekの「#121 StatefulBuilder」、「#122 RepaintBoundary」、「#123 google_fonts」を紹介しました。
今回はその続きで「#124 shared_preferences」、「#125 FocusableActionDetector」、「#126 mason」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
Flutter Widget of the Week
環境
- Flutter 3.3.10
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#124 shared_preferences
shared_preferencesとは
プラットフォーム固有の永続ストレージにデータを保存することができるパッケージです。
iOSだとUserDefaults、AndroidだとSharedPreferencesのことです。
サンプルコード
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
class SamplePage124 extends StatefulWidget {
const SamplePage124({
super.key,
});
@override
State<SamplePage124> createState() => _SamplePage124State();
}
class _SamplePage124State extends State<SamplePage124> {
late final SharedPreferences sharedPreferences;
int count = 0;
@override
void initState() {
super.initState();
Future(() async {
sharedPreferences = await SharedPreferences.getInstance();
if (mounted) {
setState(() {
count = sharedPreferences.getInt('counter') ?? 0;
});
}
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('shared_preferences'),
centerTitle: true,
actions: [
IconButton(
onPressed: () async {
await sharedPreferences.remove('counter');
count = 0;
setState(() {});
},
icon: const Icon(Icons.delete),
),
],
),
body: SafeArea(
child: Center(
child: Text(
count.toString(),
style: Theme.of(context).textTheme.headline4,
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
count++;
await sharedPreferences.setInt('counter', count);
setState(() {});
},
child: const Icon(Icons.add),
),
);
}
}
結果
わかりにくいですが、3が画面から一度離れても記憶され続けています。
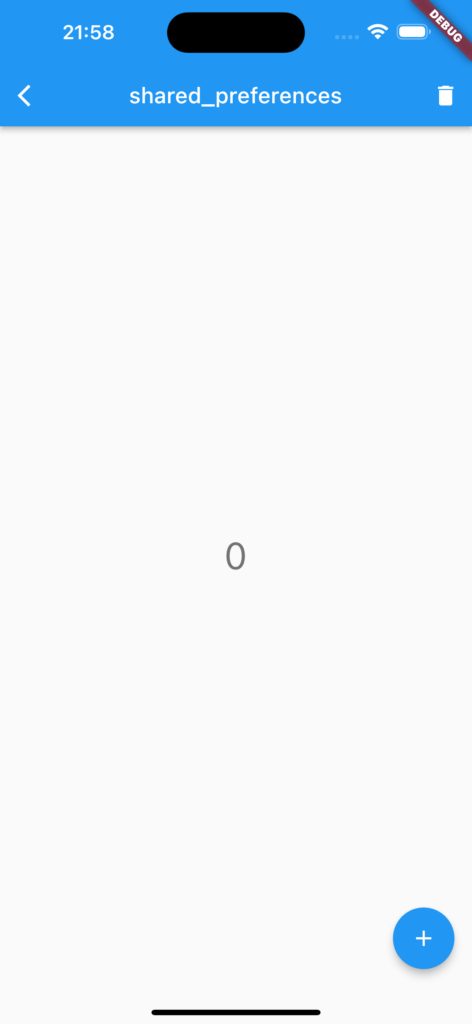
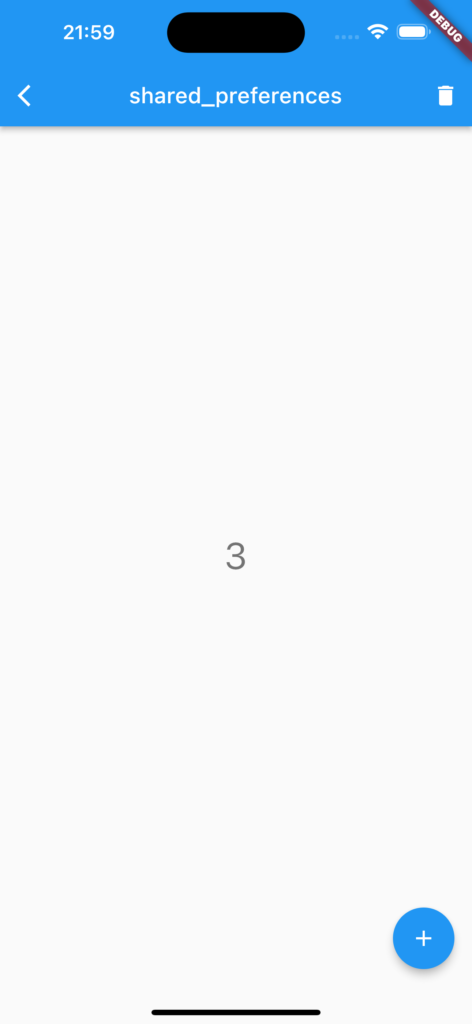
動画
公式リファレンス
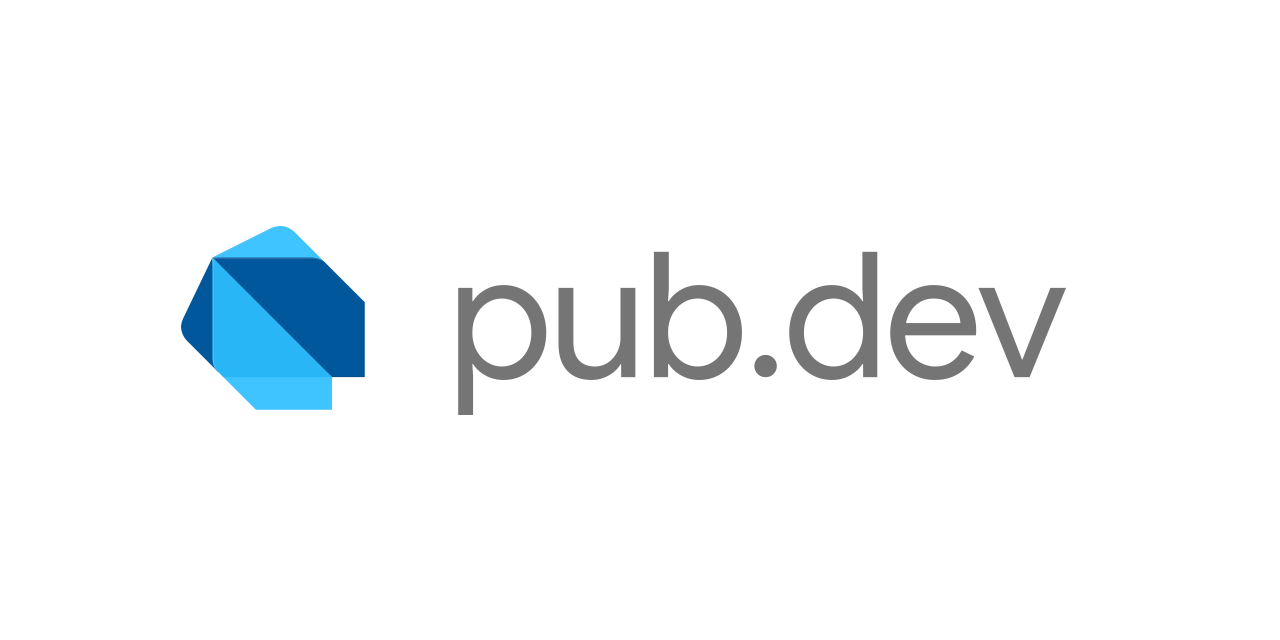
#125 FocusableActionDetector
FocusableActionDetectorとは
デスクトップアプリやWebアプリでユーザーが求めるホバー時のハイライトやフォーカス表示、キーボードのショートカットをウィジェットで表現するには階層が深くなります。
さらに1つでも順番を間違えると機能しません。
そんな時に便利なのがこのFocusableActionDetectorです。このウィジェットだけで上記の内容が網羅できます。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage125 extends StatefulWidget {
const SamplePage125({
super.key,
});
@override
State<SamplePage125> createState() => _SamplePage125State();
}
class _SamplePage125State extends State<SamplePage125> {
String message = '';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('FocusableActionDetector'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
Text(
message,
style: Theme.of(context).textTheme.headline4,
),
const SizedBox(height: 20),
GestureDetector(
onTap: () {
setState(() {
message = 'onTap';
});
},
child: FocusableActionDetector(
child: const Icon(
Icons.flutter_dash,
color: Colors.blue,
size: 128,
),
onFocusChange: (value) {
setState(() {
message = 'onFocusChange($value)';
});
},
onShowFocusHighlight: (value) {
setState(() {
message = 'onShowFocusHighlight($value)';
});
},
onShowHoverHighlight: (value) {
setState(() {
message = 'onShowHoverHighlight($value)';
});
},
),
),
],
),
),
),
);
}
}
結果
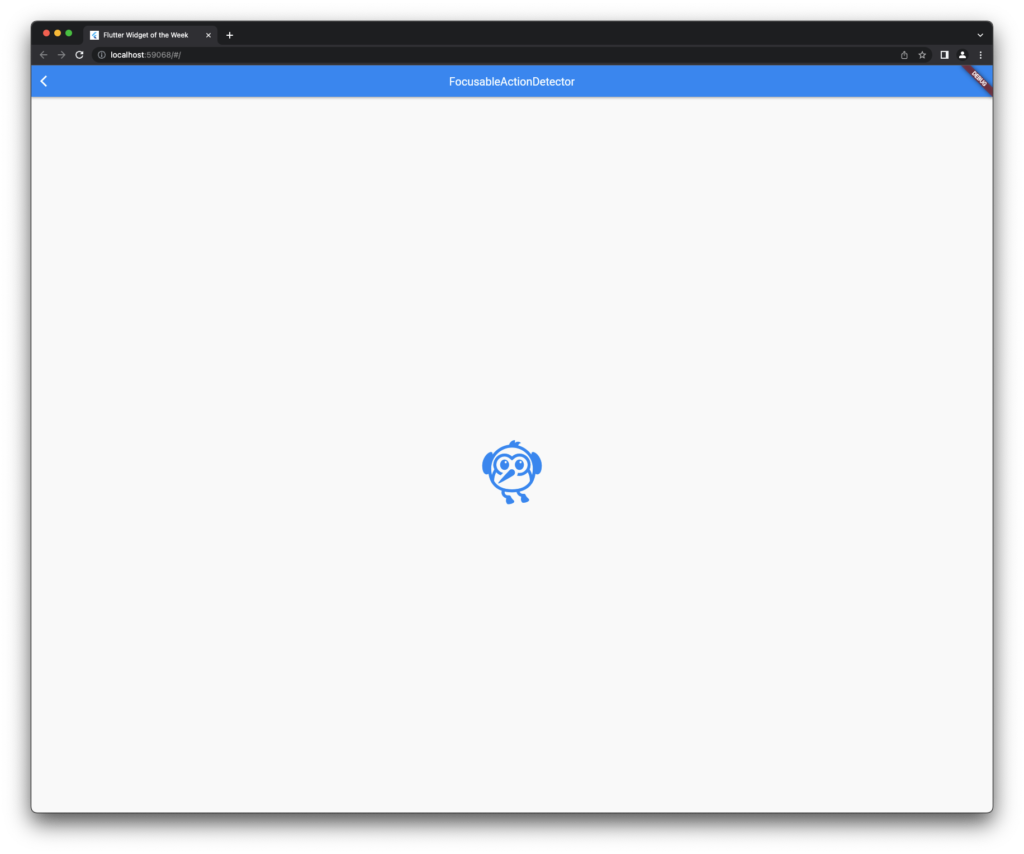
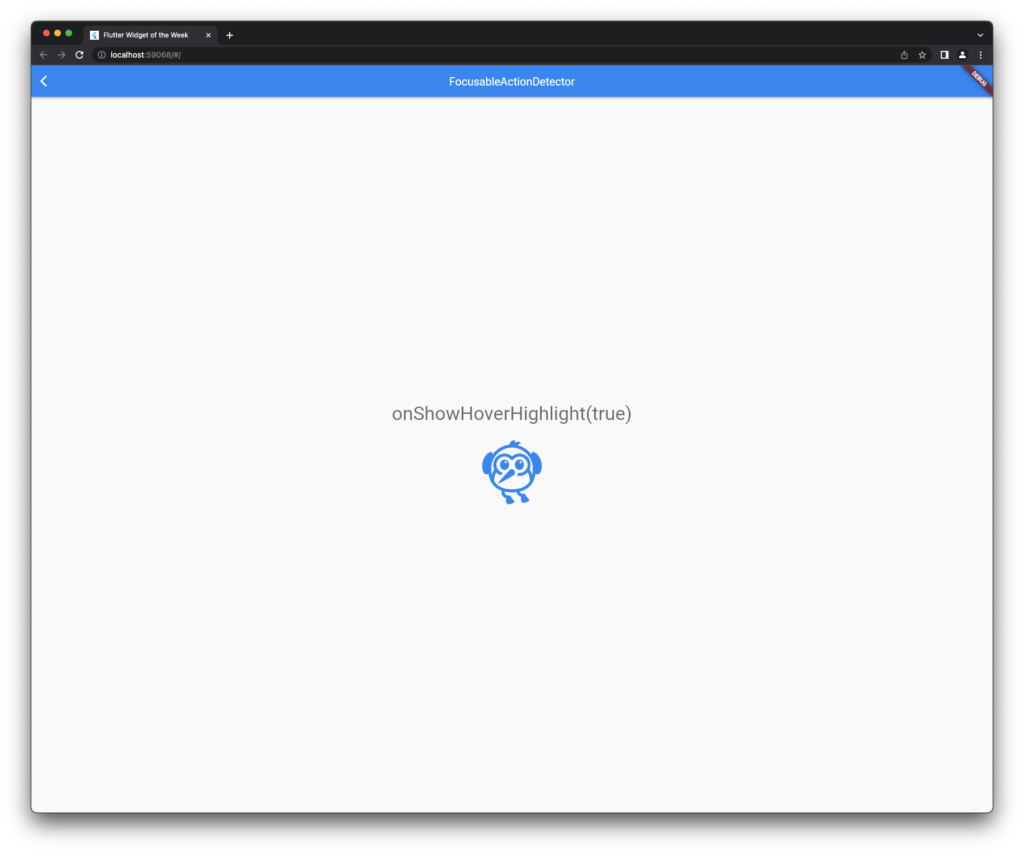
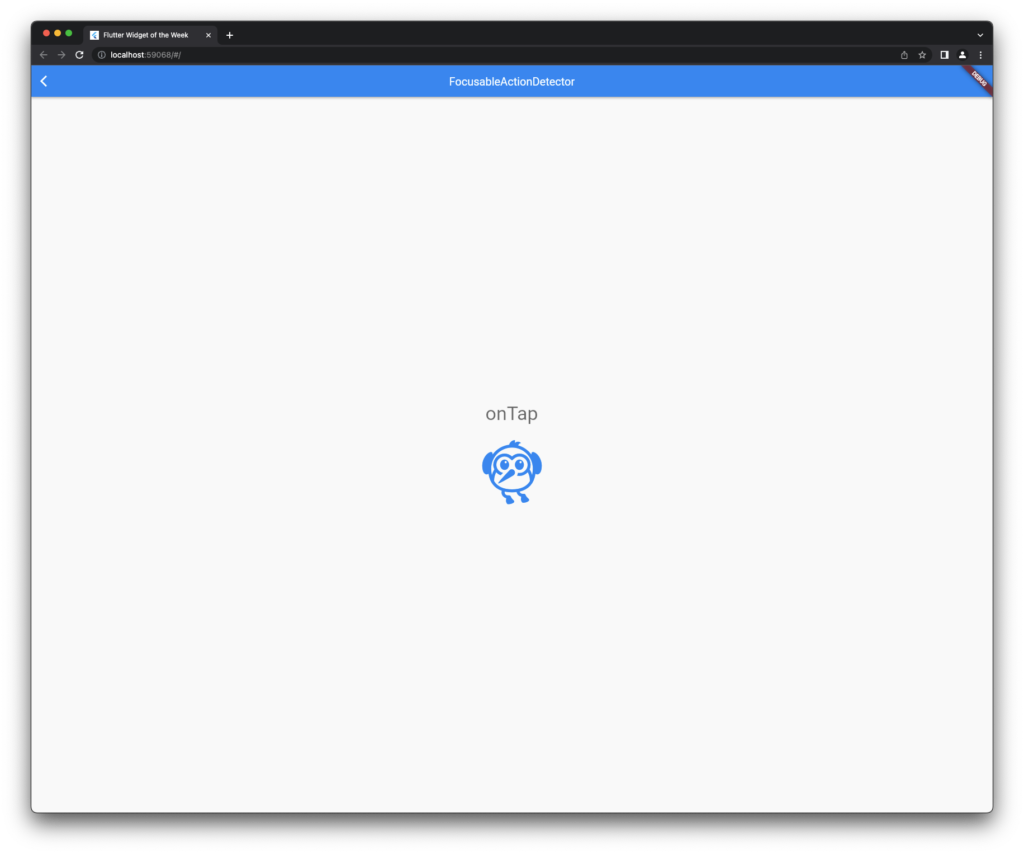
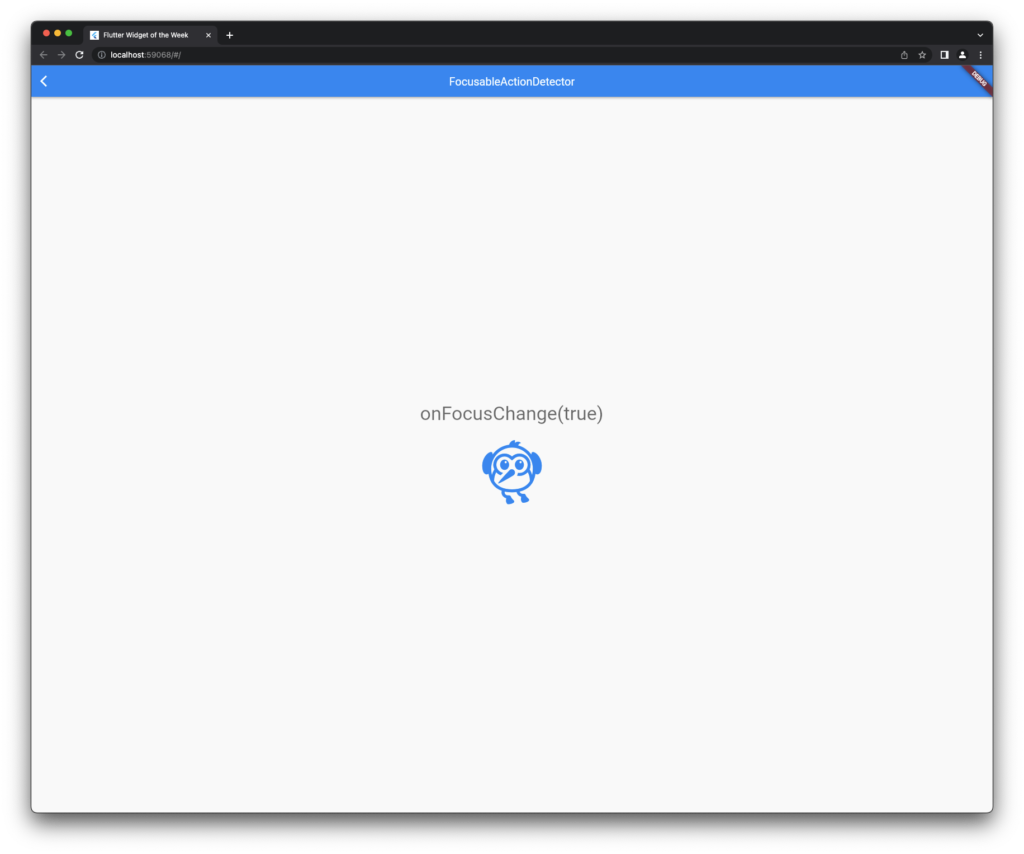
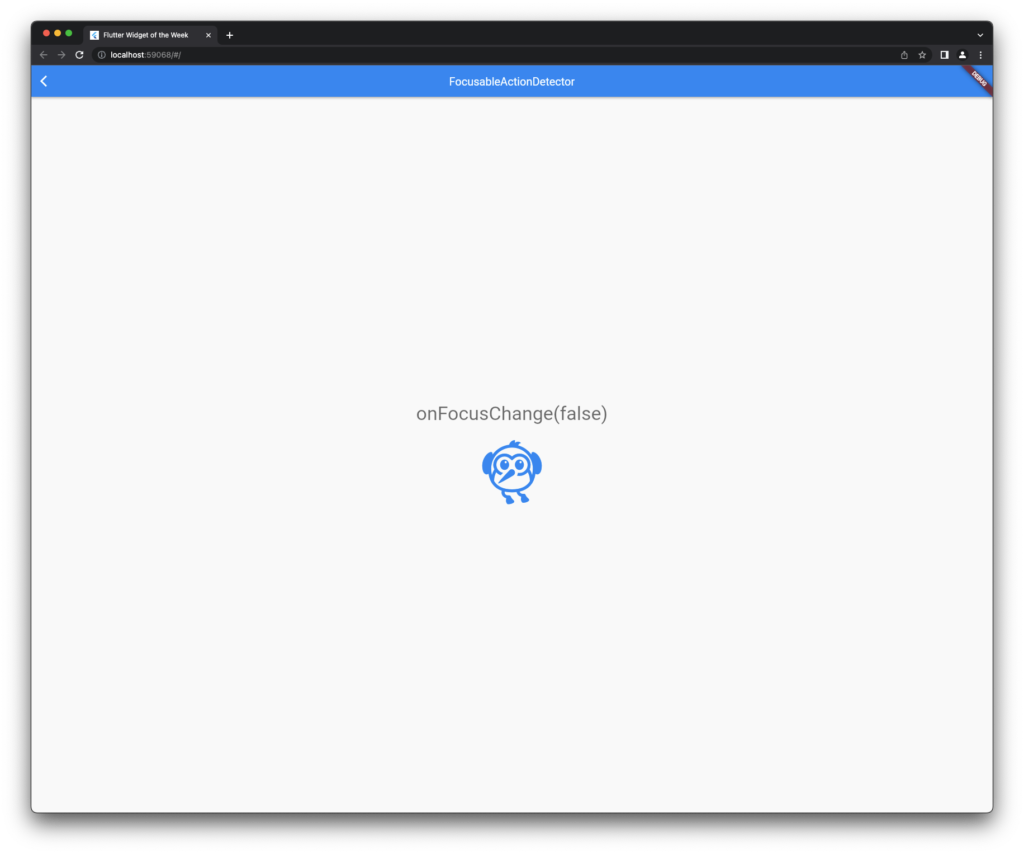
動画
公式リファレンス
#126 mason
masonとは
このパッケージを使うとbricksという事前定義されたブロックで新しいプロジェクトや機能をさっと構築できるそうです。
ただ、自分には使い方がよくわかりませんでした。。。
サンプルコード
うまく動かないので注意
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:mason/mason.dart';
class SamplePage126 extends StatefulWidget {
const SamplePage126({
super.key,
});
@override
State<SamplePage126> createState() => _SamplePage126State();
}
class _SamplePage126State extends State<SamplePage126> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('mason'),
centerTitle: true,
),
body: const SafeArea(
child: Center(
child: Text('mason'),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
// 動かないので注意.
final brick = Brick.git(
const GitPath(
'https://github.com/felangel/mason',
path: 'bricks/greeting',
),
);
final generator = await MasonGenerator.fromBrick(brick);
final target = DirectoryGeneratorTarget(Directory.current);
await generator
.generate(target, vars: <String, dynamic>{'name': 'Dash'});
},
child: const Icon(Icons.download),
),
);
}
}
結果
動作できなかったのでなし
動画
公式リファレンス
さいごに
このシリーズ、終りが見えない。。。
そして追いつく前にYouTubeのFlutter Widget of the Weekが終わってしまい、悲しみ。。。と思ったら再開された?
コメント