はじめに
前回はFlutter Widget of the Weekの「#94 animations」、「#95 flutter_slidable」、「#96 RotatedBox」を紹介しました。
今回はその続きで「#97 ExpansionPanel」、「#98 Scrollbar」、「#99 connectivity_plus」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.3.5
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#97 ExpansionPanel
ExpansionPanelとは
タップした時に詳細が表示されるような表現ができるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage097 extends StatefulWidget {
const SamplePage097({
super.key,
});
@override
State<SamplePage097> createState() => _SamplePage097State();
}
class _SamplePage097State extends State<SamplePage097> {
List<bool> isExpandedList = [
false,
false,
false,
false,
false,
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ExpansionPanel'),
centerTitle: true,
),
body: SafeArea(
child: SingleChildScrollView(
child: ExpansionPanelList(
children: [
for (int i = 0; i < isExpandedList.length; i++)
ExpansionPanel(
isExpanded: isExpandedList[i],
headerBuilder: (context, isExpanded) {
return Text('Hello index : $i');
},
body: Text('Now Open! index : $i'),
),
],
expansionCallback: (panelIndex, isExpanded) {
setState(() {
isExpandedList[panelIndex] = !isExpandedList[panelIndex];
});
},
),
),
),
);
}
}
結果
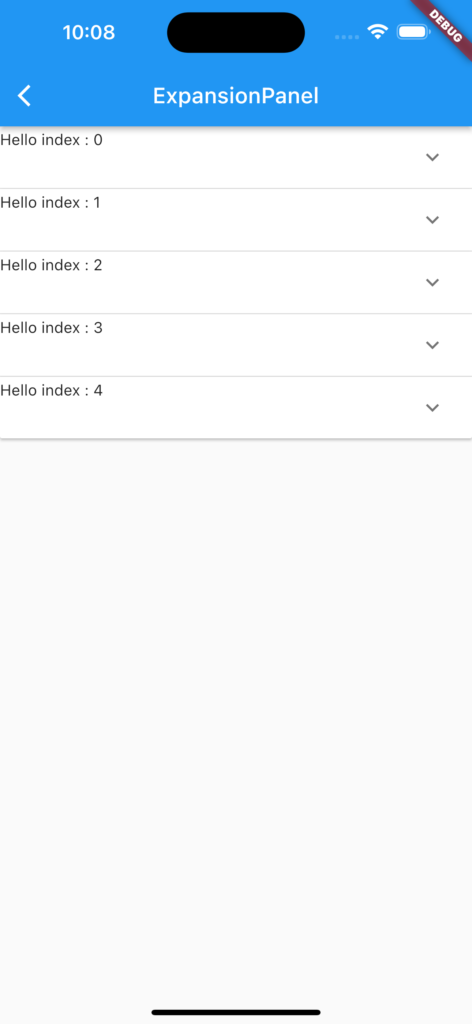
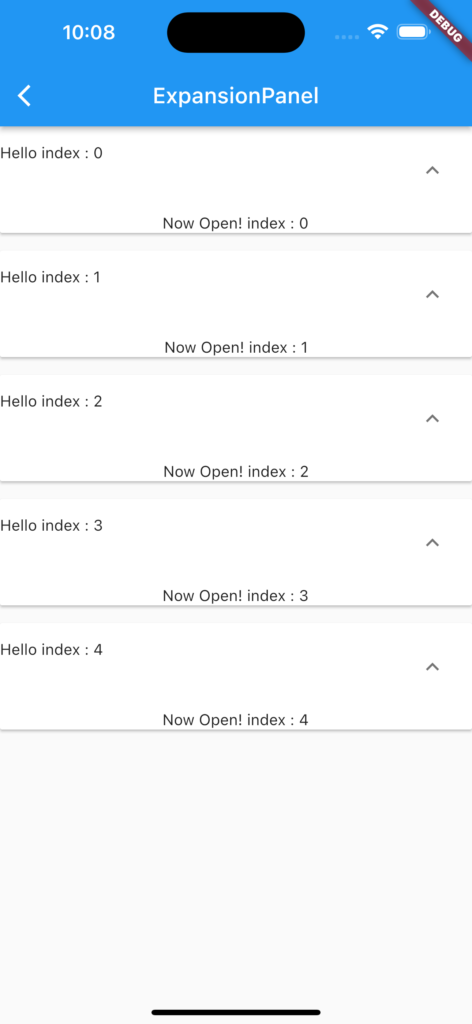
動画
公式リファレンス
ExpansionPanel class - material library - Dart API
API docs for the ExpansionPanel class from the material library, for the Dart programming language.
#98 Scrollbar
Scrollbarとは
その名の通りスクロールバーのウィジェットです。
Flutterは通常スクロール可能な場合でもスクロールバーが表示されません。表示したい場合はこのウィジェットを利用します。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage098 extends StatelessWidget {
const SamplePage098({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Scrollbar'),
centerTitle: true,
),
body: SafeArea(
child: Scrollbar(
thumbVisibility: true,
trackVisibility: true,
child: ListView.builder(
itemCount: 50,
itemExtent: 50,
itemBuilder: (context, index) {
return Container(
alignment: Alignment.center,
color: Colors.blue[100 * (index % 9)],
child: Text('List Item $index'),
);
},
),
),
),
);
}
}
結果
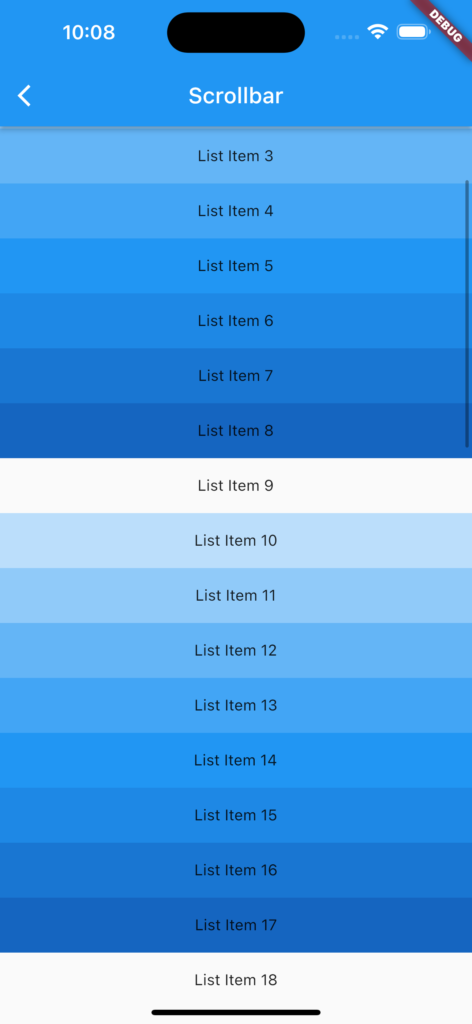
動画
公式リファレンス
Scrollbar class - material library - Dart API
API docs for the Scrollbar class from the material library, for the Dart programming language.
#99 connectivity_plus
connectivity_plusとは
名前でわかる通り、ネットワークの接続状態をしれるパッケージです。
サンプルコード
import 'dart:async';
import 'package:connectivity_plus/connectivity_plus.dart';
import 'package:flutter/material.dart';
class SamplePage099 extends StatefulWidget {
const SamplePage099({
super.key,
});
@override
State<SamplePage099> createState() => _SamplePage099State();
}
class _SamplePage099State extends State<SamplePage099> {
late final StreamSubscription<ConnectivityResult> subscription;
final streamController = StreamController<ConnectivityResult>();
Stream<ConnectivityResult> get stream => streamController.stream;
@override
void initState() {
super.initState();
subscription = Connectivity().onConnectivityChanged.listen((result) {
streamController.sink.add(result);
debugPrint(result.toString());
});
}
@override
void dispose() {
streamController.close();
subscription.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('connectivity_plus'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: StreamBuilder(
stream: stream,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return const Center(
child: CircularProgressIndicator(),
);
}
if (snapshot.hasError || !snapshot.hasData) {
return const Center(
child: Icon(
Icons.error,
color: Colors.red,
),
);
}
return Text(snapshot.data!.toString());
},
),
),
),
);
}
}
結果
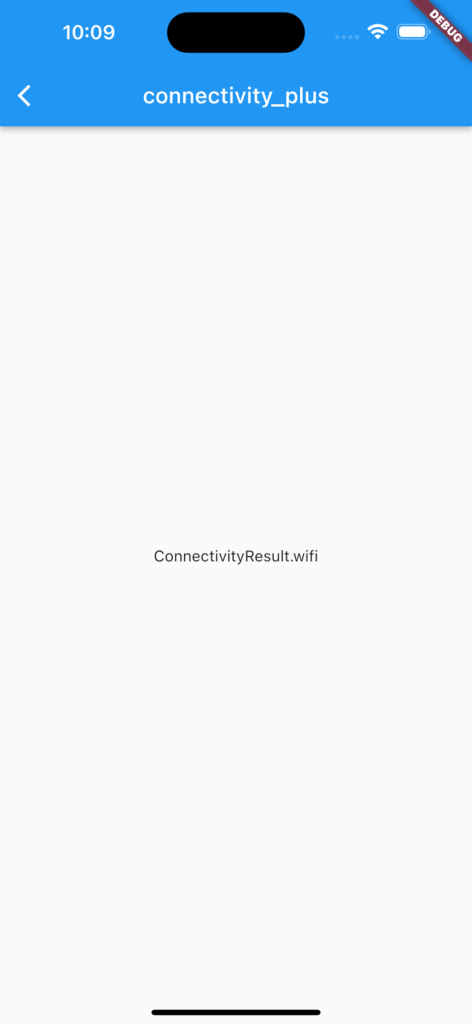
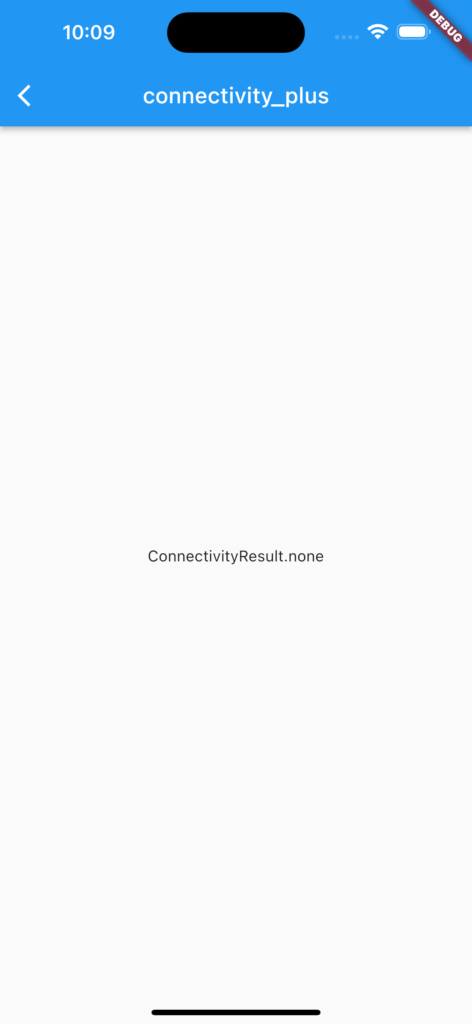
動画
公式リファレンス
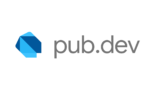
connectivity_plus | Flutter Package
Flutter plugin for discovering the state of the network (WiFi & mobile/cellular) connectivity on Android and iOS.
さいごに
このシリーズ、終りが見えない。。。
そして追いつく前にYouTubeのFlutter Widget of the Weekが終わってしまい、悲しみ。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント