はじめに
Unityでゲームを製作しているとMonoBehaviourを継承しているクラスのメソッドをどこからでも呼び出したいことがよくありますよ?
今回はそれを実現するMonoBehaviourをSingletonにする方法を紹介します。
環境
- Unity 2021.1.4f1
シングルトンって何?
オブジェクト指向プログラミングにおけるクラスのデザインパターンの一つです。実行時にそのクラスのインスタンスが必ず単一になるよう設計することを言います。
サンプルプログラム
基底クラスを準備
SingletonMonoBehaviour.cs
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public abstract class SingletonMonoBehaviour<T> : MonoBehaviour where T : MonoBehaviour
{
protected abstract bool dontDestroyOnLoad { get; }
private static T instance;
public static T Instance
{
get
{
if (!instance)
{
Type t = typeof(T);
instance = (T)FindObjectOfType(t);
if (!instance)
{
Debug.LogError(t + " is nothing.");
}
}
return instance;
}
}
protected virtual void Awake()
{
if (this != Instance)
{
Destroy(this);
return;
}
if (dontDestroyOnLoad)
{
DontDestroyOnLoad(this.gameObject);
}
}
}
サンプルプログラム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TestManager : SingletonMonoBehaviour<TestManager>
{
protected override bool dontDestroyOnLoad { get { return true; } }
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
public void Test()
{
Debug.Log("テストです");
}
}
これをGameObjectにアタッチして
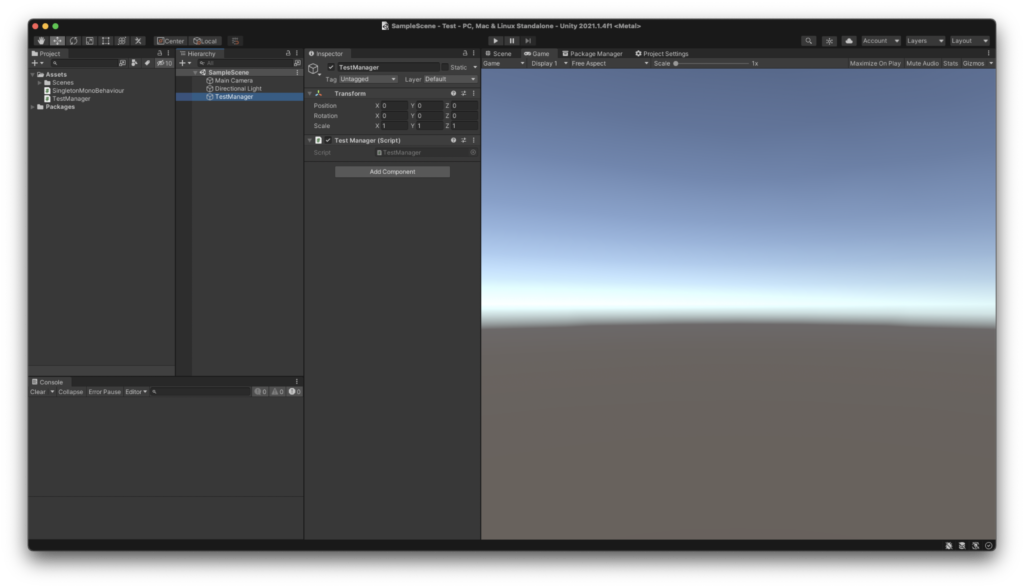
実行すると。。。
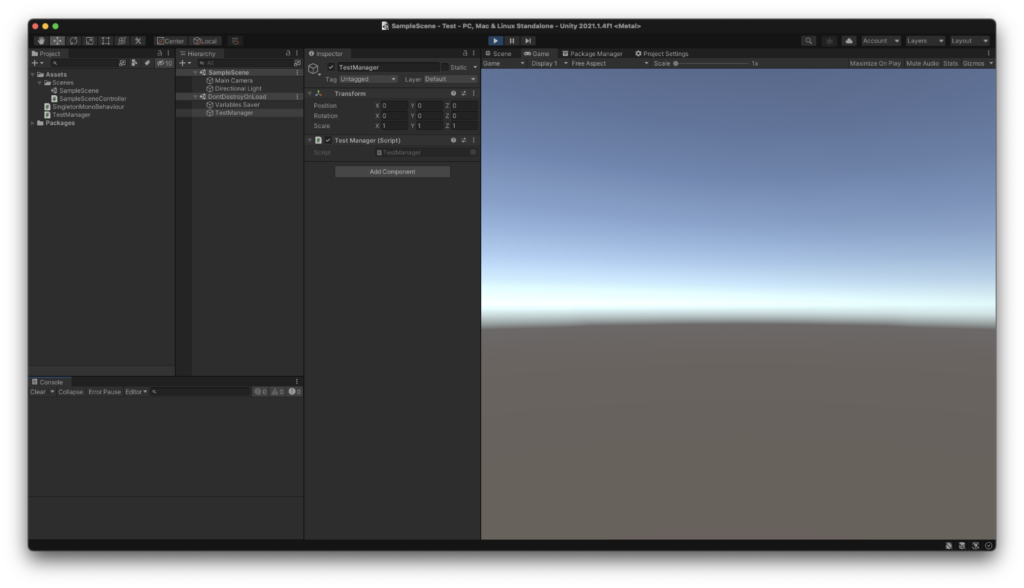
無事、DontDestroyOnLoadになりました。
これで以下のプログラムでスクリプト上でTestManagerのTest()をどこからでも呼びだせます
{
TestManager.Instance.Test();
}
さいごに
ひんぱんに使うと思うので、ぜひ覚えてください。
コメント