はじめに
前回はFlutter Widget of the Weekの「#148 cloud_firestore」、「#149 firebase_analytics」、「#150 home_widget」を紹介しました。
今回はその続きで「#151 firebase_auth」、「#152 fl_chart」、「#153 gap」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.19.6
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#151 firebase_auth
firebase_authとは?
Firebaseで認証機能が提供されているサービスのFirebase Authenticationを扱えるようになるパッケージです。
メール・パスワード、電話番号、OAuthプロバイダを使った認証が簡単に実装できます。
また匿名認証も扱えるのでセキュリティールールによりデータを保護できます。
サンプルコード
void main() async {
WidgetsFlutterBinding.ensureInitialized();
// Firebase.
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
runApp(const MyApp());
}
import 'package:firebase_auth/firebase_auth.dart';
import 'package:flutter/material.dart';
class SamplePage151 extends StatefulWidget {
const SamplePage151({
super.key,
});
@override
State<SamplePage151> createState() => _SamplePage151State();
}
class _SamplePage151State extends State<SamplePage151> {
final emailAddress = TextEditingController(text: '');
final password = TextEditingController(text: '');
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('firebase_auth'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Form(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 20),
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
TextField(
controller: emailAddress,
decoration: const InputDecoration(
icon: Icon(Icons.mail_outlined),
label: Text('メールアドレス'),
),
),
TextField(
controller: password,
obscureText: true,
decoration: const InputDecoration(
icon: Icon(Icons.password_outlined),
label: Text('パスワード'),
),
),
const SizedBox(height: 120),
FilledButton(
onPressed: () async {
try {
await FirebaseAuth.instance
.createUserWithEmailAndPassword(
email: emailAddress.text,
password: password.text,
);
} on FirebaseAuthException catch (e) {
if (e.code == 'weak-password') {
// ignore: avoid_print
print('The password provided is too weak.');
} else if (e.code == 'email-already-in-use') {
// ignore: avoid_print
print('The account already exists for that email.');
}
} catch (e) {
// ignore: avoid_print
print(e);
}
},
child: const Text('ユーザー登録'),
),
],
),
),
),
),
),
);
}
}
結果
メールアドレスとパスワードを入力し、ユーザー登録ボタンを押すと。。。
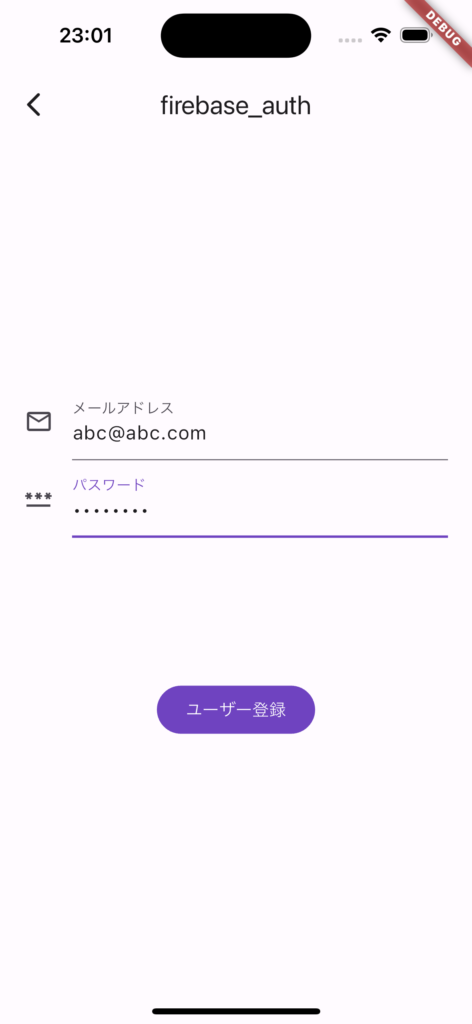
Firebase Authenticationでユーザーが無事登録されました。
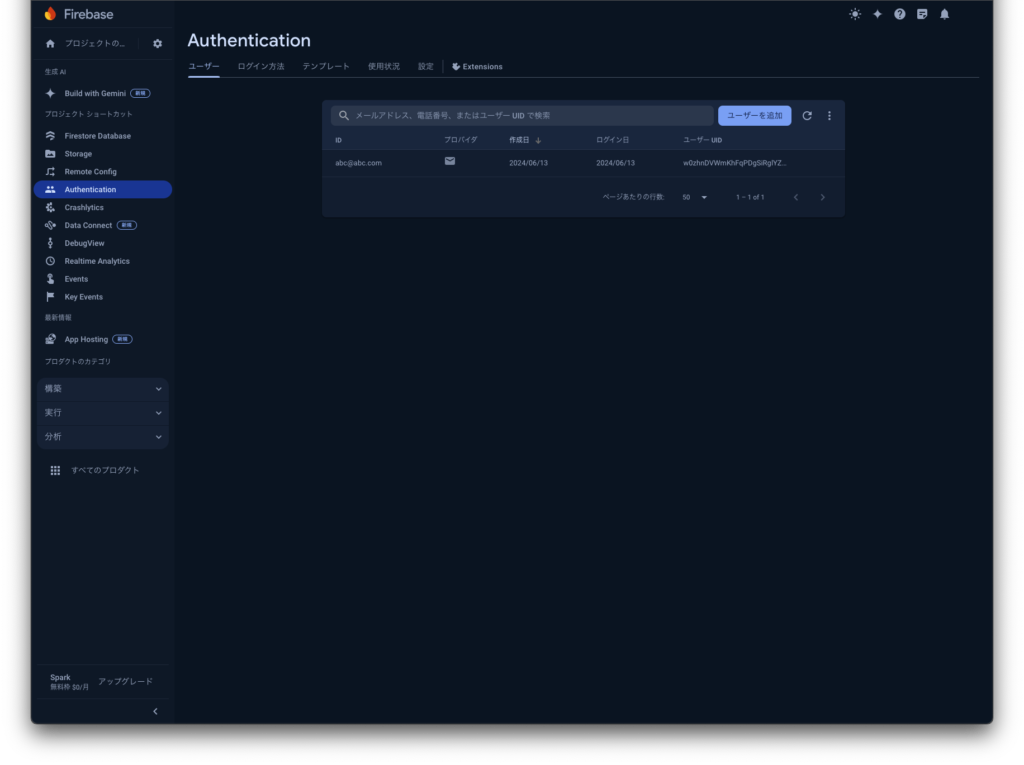
動画
公式リファレンス
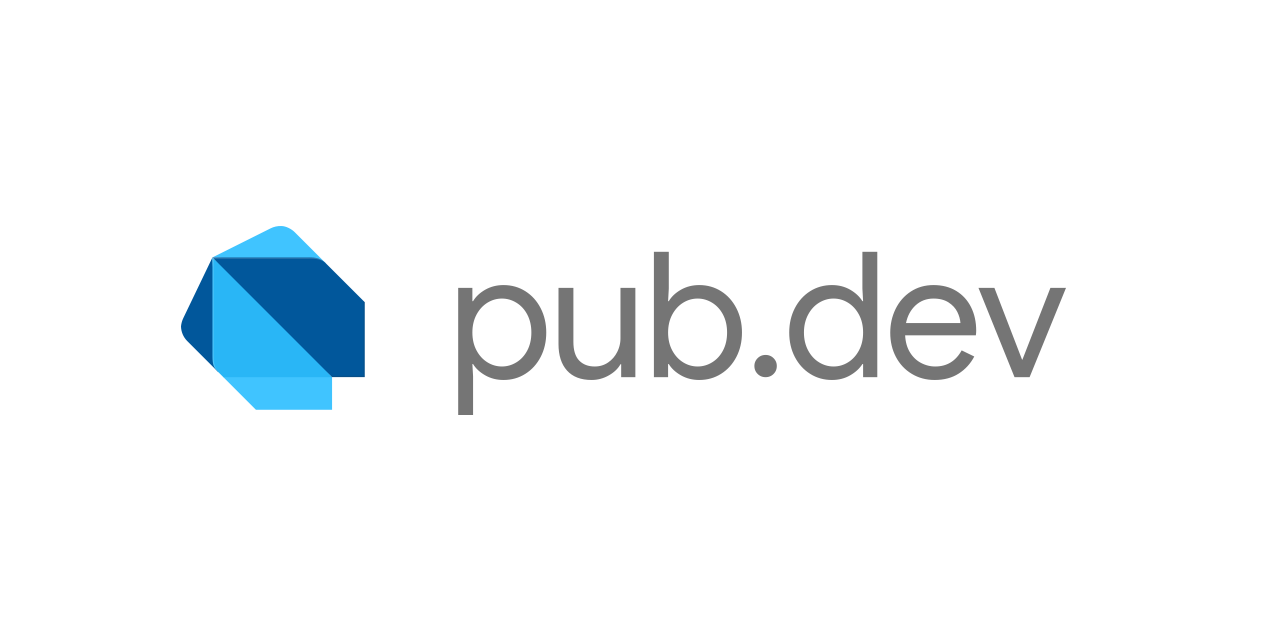
firebase_auth | Flutter package
Flutter plugin for Firebase Auth, enabling authentication using passwords, phone numbers and identity providers like Goo...
#152 fl_chart
fl_chartとは?
様々なグラフを表示できるパッケージです。
折れ線グラフ、棒グラフ、円グラフ、散布図、レーダーチャート等に対応しています。
サンプルコード
import 'package:fl_chart/fl_chart.dart';
import 'package:flutter/material.dart';
class SamplePage152 extends StatefulWidget {
const SamplePage152({
super.key,
});
@override
State<SamplePage152> createState() => _SamplePage152State();
}
class _SamplePage152State extends State<SamplePage152> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('fl_chart'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 20),
child: PieChart(
PieChartData(
sections: [
PieChartSectionData(
value: 500,
title: r'Investments: $500',
showTitle: true,
radius: 70,
color: Colors.green,
),
PieChartSectionData(
value: 1200,
title: r'Housing: $1200',
showTitle: true,
radius: 70,
color: Colors.blue,
),
PieChartSectionData(
value: 400,
title: r'Food: $400',
showTitle: true,
radius: 70,
color: Colors.pink,
),
PieChartSectionData(
value: 400,
title: r'Fun: $400',
showTitle: true,
radius: 70,
color: Colors.yellow,
),
PieChartSectionData(
value: 80,
title: r'Internet: $80',
showTitle: true,
radius: 70,
color: Colors.purple,
),
],
),
),
),
),
),
);
}
}
結果
簡単に円グラフが実装できちゃいました。
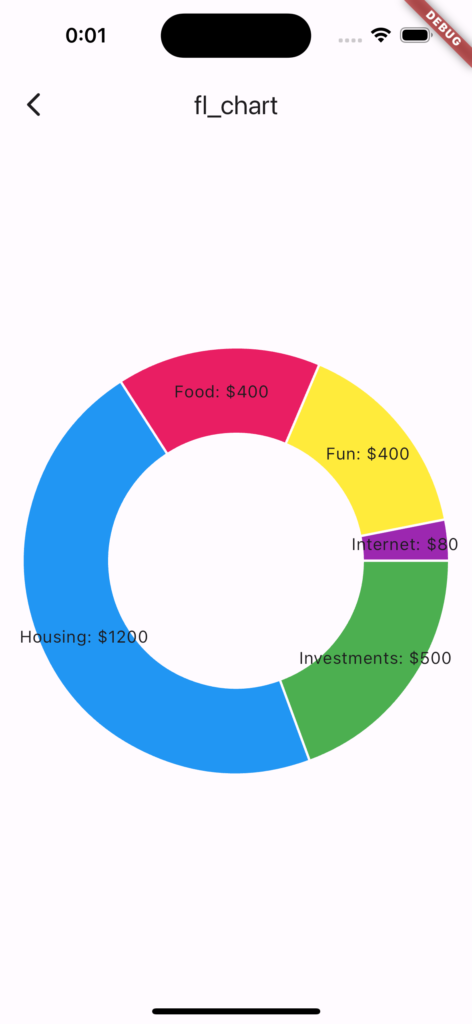
動画
公式リファレンス
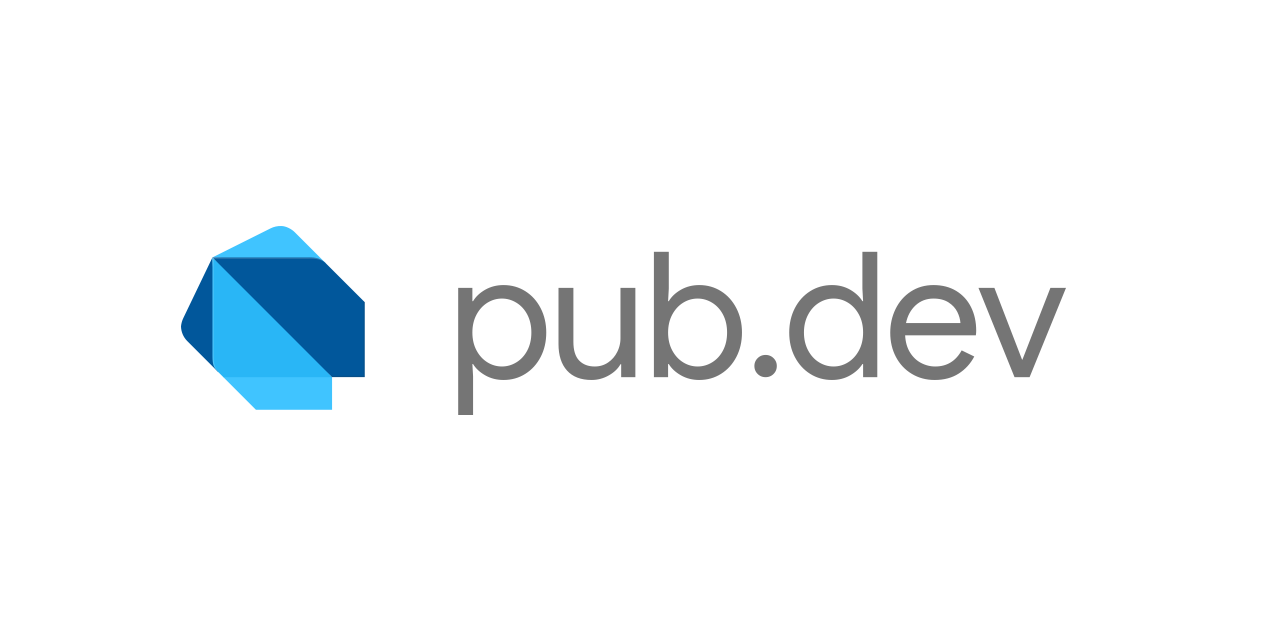
fl_chart | Flutter package
A highly customizable Flutter chart library that supports Line Chart, Bar Chart, Pie Chart, Scatter Chart, and Radar Cha...
#153 gap
gapとは?
ColumnやListView等で間隔を空けられるウィジェットを提供するパッケージです。
SizedBoxやPadding等でも可能ですが、隙間を空ける方向が指定する必要があります。
しかしこのgapには不要です。
サンプルコード
import 'package:flutter/material.dart';
import 'package:gap/gap.dart';
class SamplePage153 extends StatefulWidget {
const SamplePage153({
super.key,
});
@override
State<SamplePage153> createState() => _SamplePage153State();
}
class _SamplePage153State extends State<SamplePage153> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('gap'),
centerTitle: true,
),
body: SafeArea(
child: ListView(
children: [
Container(
height: 200,
color: Colors.red,
),
const Gap(20),
Container(
height: 200,
color: Colors.blue,
),
const Gap(20),
Container(
height: 200,
color: Colors.yellow,
),
const Gap(20),
Container(
height: 200,
color: Colors.green,
),
const Gap(20),
Container(
height: 200,
color: Colors.purple,
),
],
),
),
);
}
}
結果
Gapのほうが楽ですね。。。
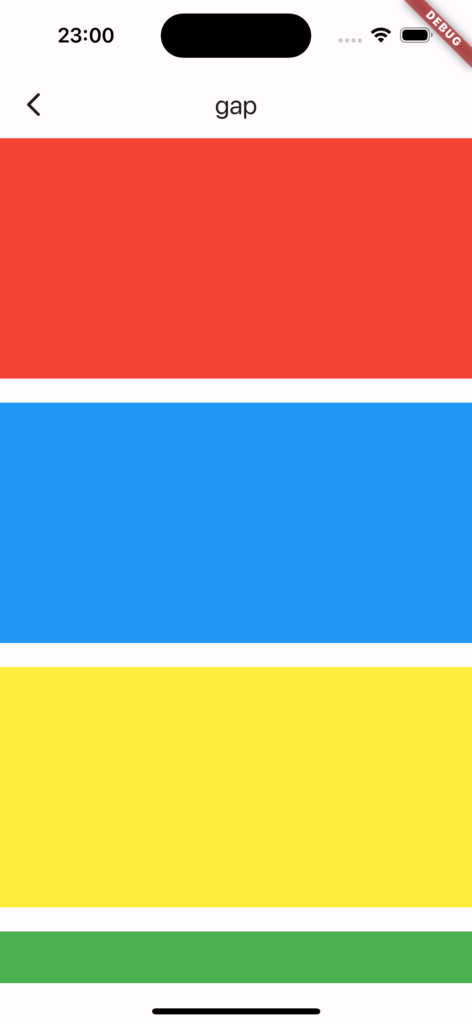
動画
公式リファレンス
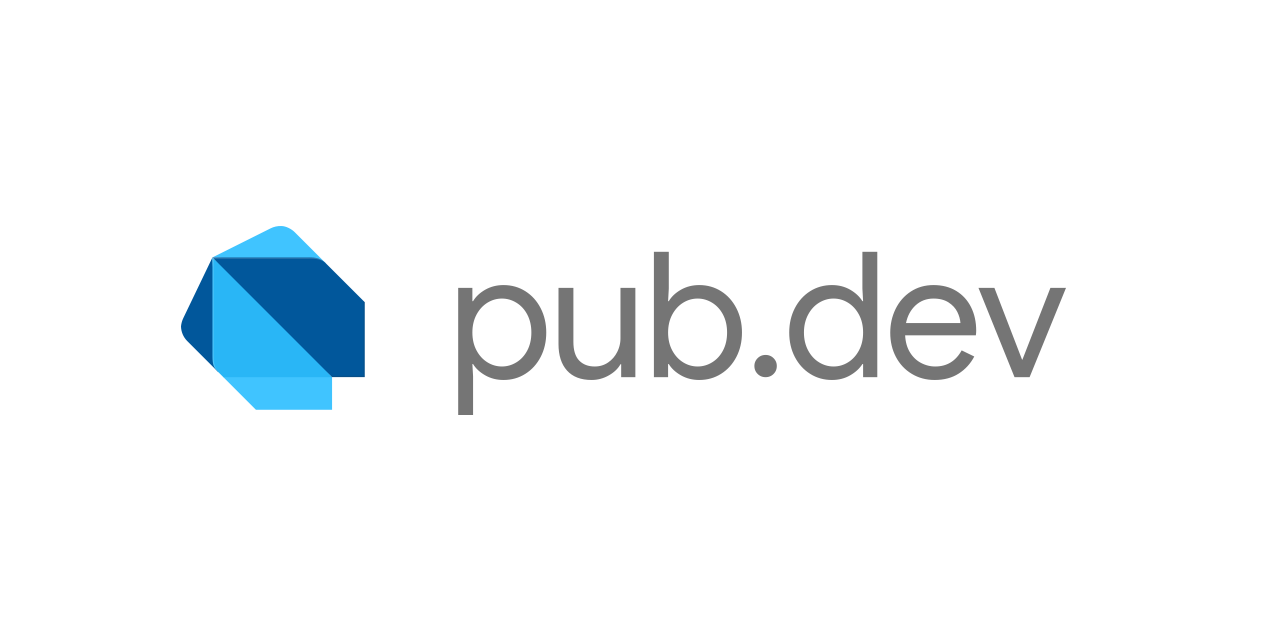
gap | Flutter package
Flutter widgets for easily adding gaps inside Flex widgets such as Columns and Rows or scrolling views.
さいごに
サボってしまった。。。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント