はじめに
前回はFlutter Widget of the Weekの「#91 device_info_plus」、「#92 ImageFiltered」、「#93 PhysicalModel」を紹介しました。
今回はその続きで「#94 animations」、「#95 flutter_slidable」、「#96 RotatedBox」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.3.4
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#94 animations
animationsとは
Flutterでは色んな方法でアニメーションを作成できます。しかし作るのに時間がかかります。
そんなの時に便利なパッケージなのがこのanimationsパッケージです。
Flutterのマテリアルデザインチームが作成したパッケージで簡単に導入できます。
サンプルコード
import 'package:animations/animations.dart';
import 'package:flutter/material.dart';
class SamplePage094 extends StatelessWidget {
const SamplePage094({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('animations'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
OpenContainer(
closedBuilder: (context, action) {
return const FlutterLogo(
size: 64,
);
},
openBuilder: (context, action) {
return const SamplePage094Second();
},
),
],
),
),
),
);
}
}
class SamplePage094Second extends StatelessWidget {
const SamplePage094Second({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Second Page'),
centerTitle: true,
),
body: const Center(
child: Text(''),
),
);
}
}
結果
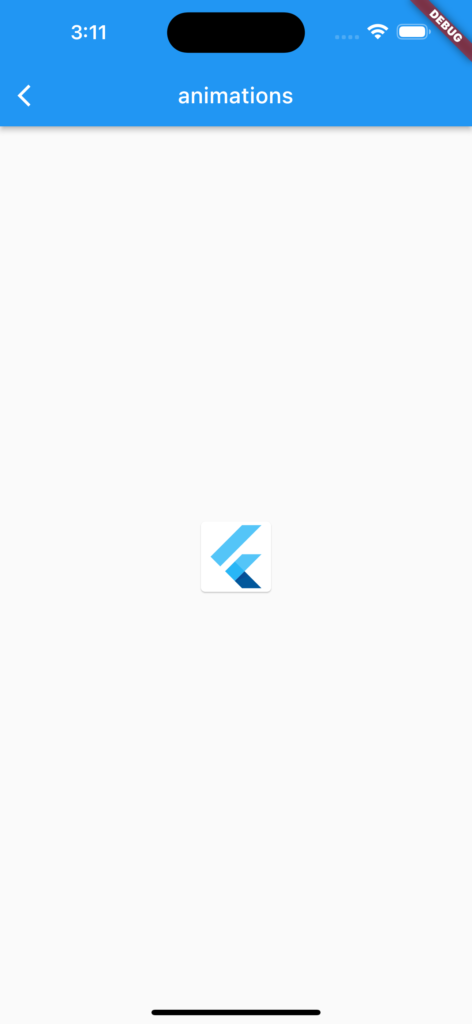
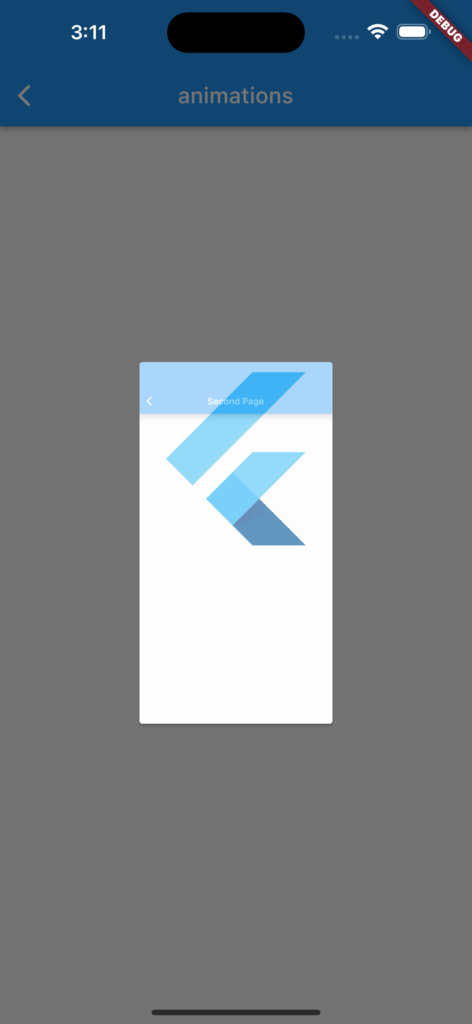
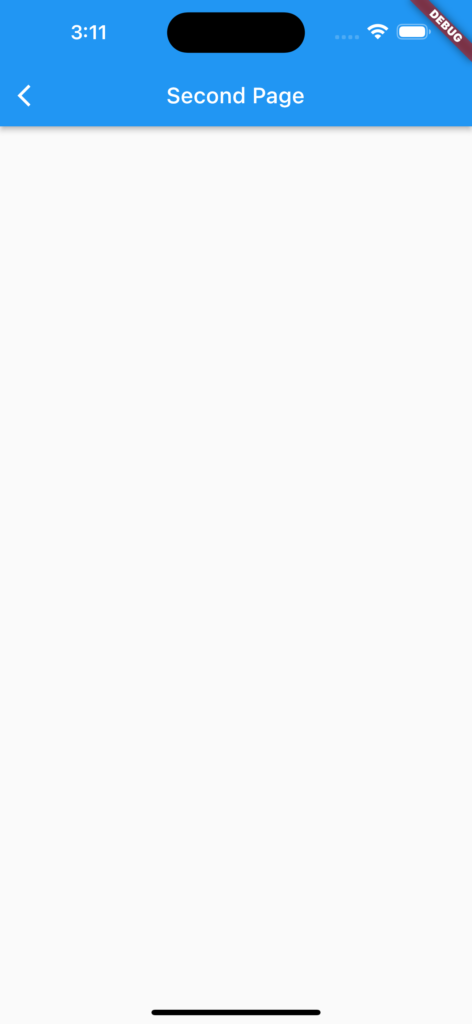
動画
公式リファレンス
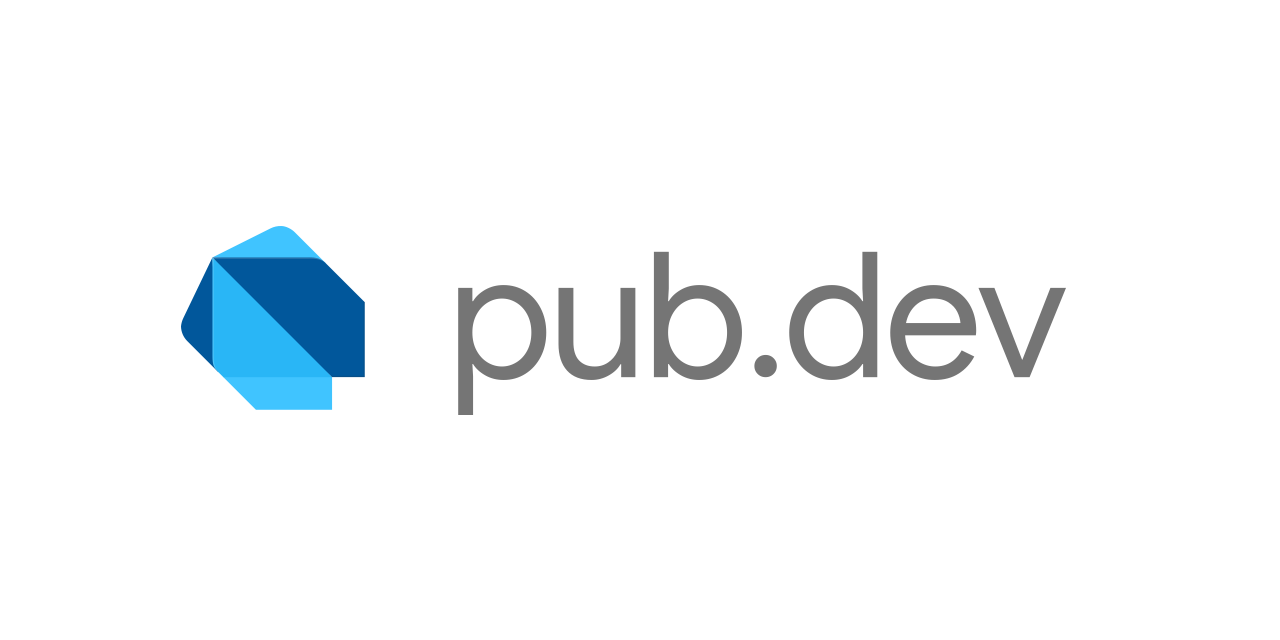
animation | Dart package
Animate HTML elements similar to jQuery's animate().
#95 flutter_slidable
flutter_slidableとは
このflutter_slidableはウィジェットをスワイプして実行可能なアクションを表示する機能が簡単に実装できるパッケージです。
サンプルコード
import 'package:flutter/material.dart';
import 'package:flutter_slidable/flutter_slidable.dart';
class SamplePage095 extends StatelessWidget {
const SamplePage095({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('flutter_slidable'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
Card(
child: Slidable(
key: const ValueKey(0),
startActionPane: ActionPane(
motion: const ScrollMotion(),
dismissible: DismissiblePane(
onDismissed: () {},
),
children: [
SlidableAction(
onPressed: (context) {},
backgroundColor: const Color(0xFFFE4A49),
foregroundColor: Colors.white,
icon: Icons.delete,
label: 'Delete',
),
SlidableAction(
onPressed: (context) {},
backgroundColor: const Color(0xFF21B7CA),
foregroundColor: Colors.white,
icon: Icons.share,
label: 'Share',
),
],
),
endActionPane: ActionPane(
motion: const ScrollMotion(),
children: [
SlidableAction(
flex: 2,
onPressed: (context) {},
backgroundColor: const Color(0xFF7BC043),
foregroundColor: Colors.white,
icon: Icons.archive,
label: 'Archive',
),
SlidableAction(
onPressed: (context) {},
backgroundColor: const Color(0xFF0392CF),
foregroundColor: Colors.white,
icon: Icons.save,
label: 'Save',
),
],
),
child: const ListTile(
title: Text('Slide me'),
),
),
),
],
),
),
),
);
}
}
結果
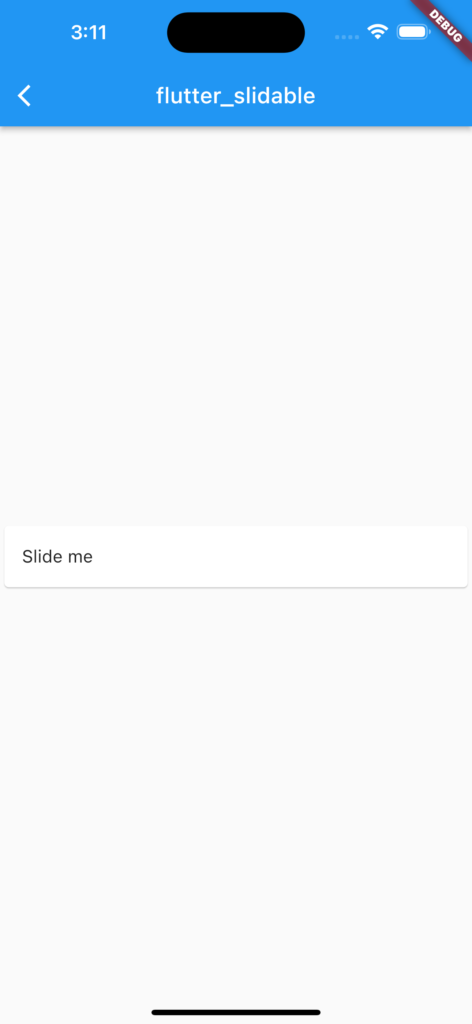
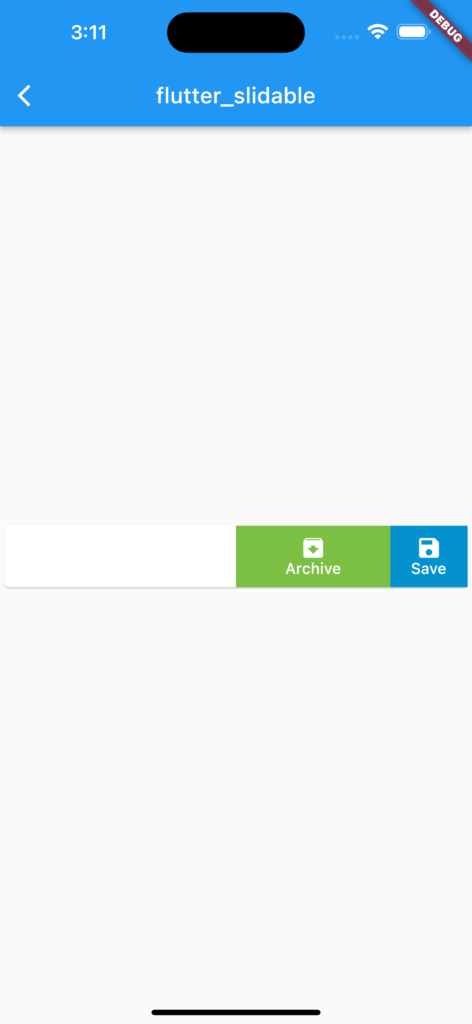
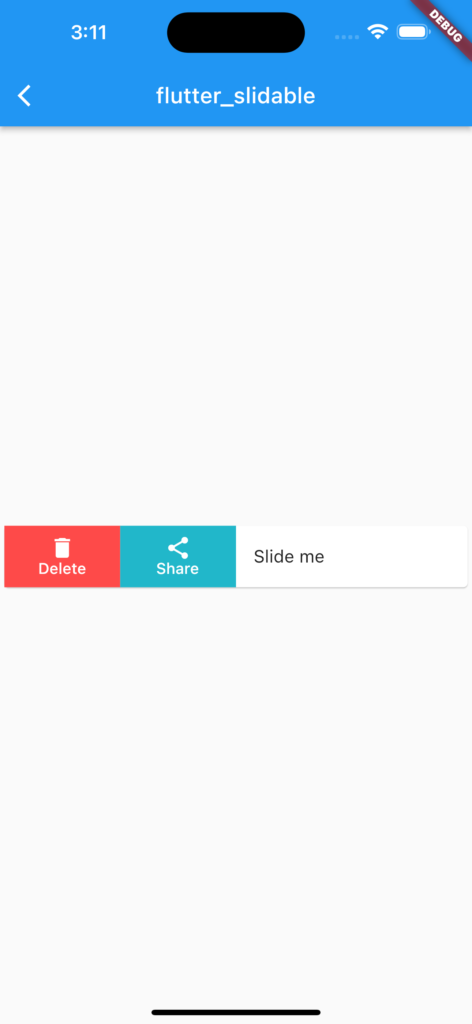
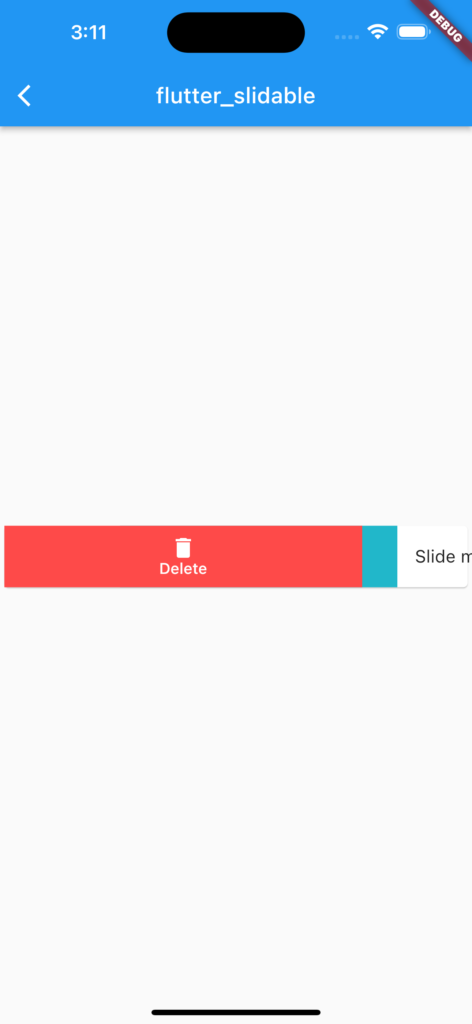
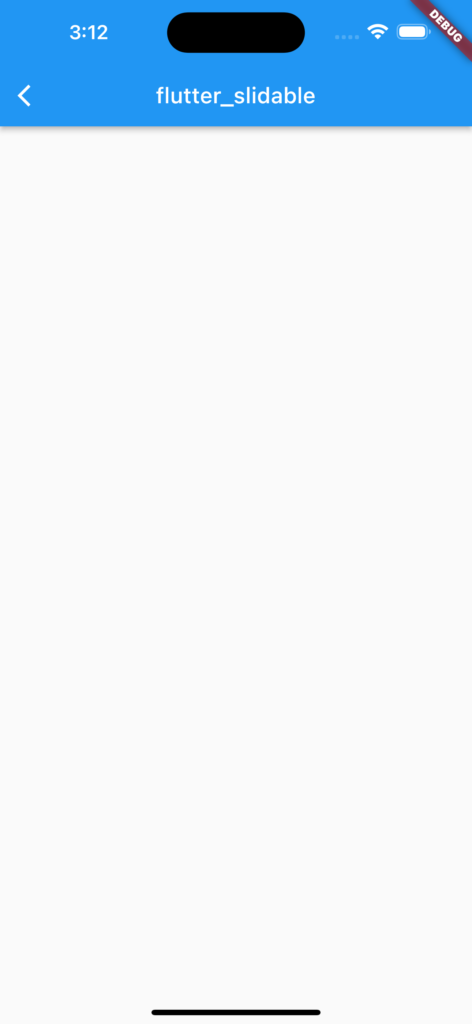
動画
公式リファレンス
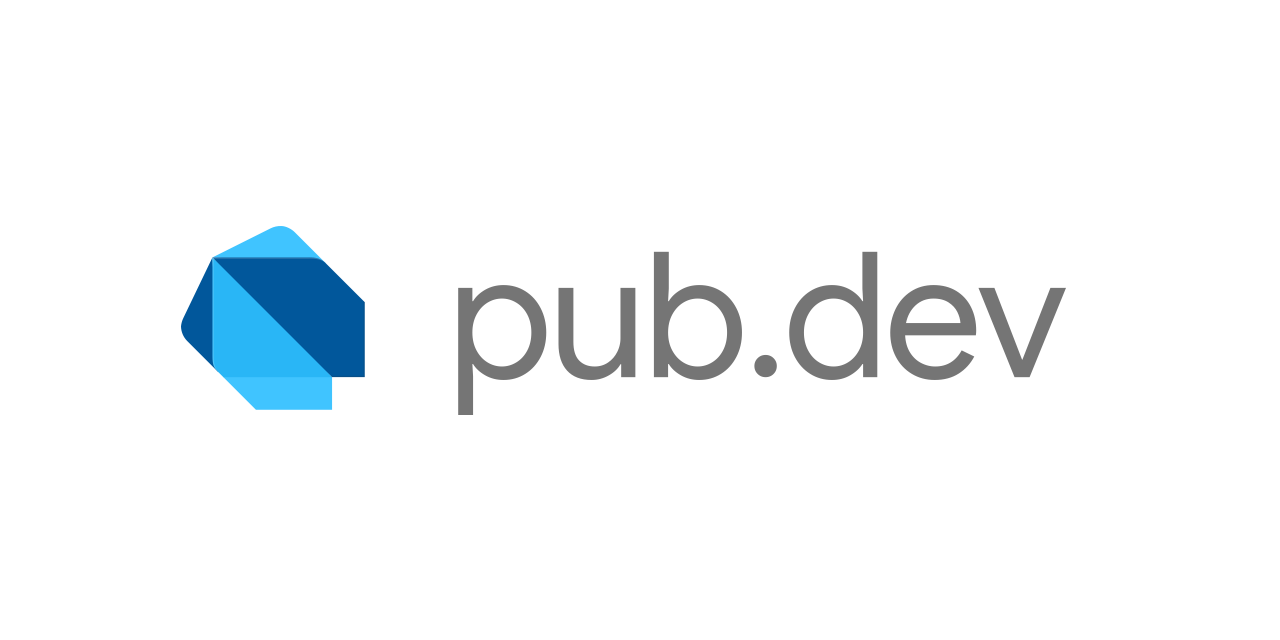
flutter_slidable | Flutter package
A Flutter implementation of slidable list item with directional slide actions that can be dismissed.
#96 RotatedBox
RotatedBoxとは
ウィジェットを回転させることができるウィジェットです。
Transform.rorateとは違いレンダリングにのみに影響を与え、レイアウトには影響を与えません。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage096 extends StatelessWidget {
const SamplePage096({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('RotatedBox'),
centerTitle: true,
),
body: SafeArea(
child: Row(
children: [
RotatedBox(
quarterTurns: 3,
child: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Container(
padding: const EdgeInsets.all(5),
margin: const EdgeInsets.all(20),
color: Colors.black,
child: Text(
'Rotation',
style: TextStyle(
color: Colors.white,
fontSize:
Theme.of(context).textTheme.headline3!.fontSize,
fontWeight: FontWeight.bold,
),
),
),
],
),
),
Expanded(
child: SingleChildScrollView(
child: Text('ABCDEFGHIJKLMNOPQRSTUVWXYZ' * 50),
),
),
RotatedBox(
quarterTurns: 3,
child: Container(
margin: const EdgeInsets.all(10),
child: Row(
children: const [
Chip(
label: Text('Dash'),
),
Chip(
label: Text('Flutter'),
),
Chip(
label: Text('Tutorial'),
),
],
),
),
)
],
),
),
);
}
}
結果
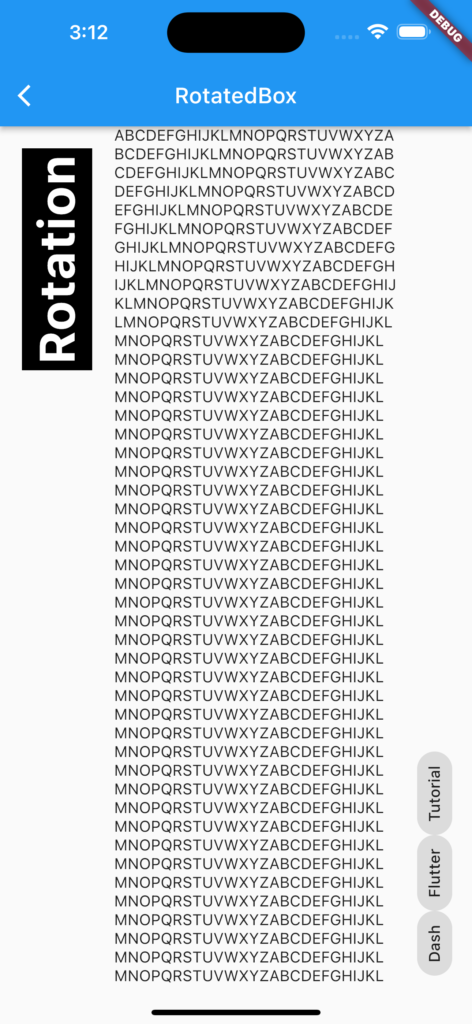
動画
公式リファレンス
RotatedBox class - widgets library - Dart API
API docs for the RotatedBox class from the widgets library, for the Dart programming language.
さいごに
このシリーズ、終りが見えない。。。
そして追いつく前にYouTubeのFlutter Widget of the Weekが終わってしまい、悲しみ。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント