はじめに
前回はFlutter Widget of the Weekの「#103 sensors_plus」、「#104 collection」、「#105 HeroMode」を紹介しました。
今回はその続きで「#106 html」、「#107 RefreshIndicator」、「#108 font_awesome_flutter」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.3.8
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#106 html
htmlとは
HTML5のパーサーで、HTMLドキュメントを作成するオブジェクトを作ることができます。
サンプルコード
import 'package:flutter/material.dart';
import 'package:html/parser.dart';
class SamplePage106 extends StatelessWidget {
const SamplePage106({
super.key,
});
String get myHtml => '<body><div id="dash"><p>Hello</p></body>';
@override
Widget build(BuildContext context) {
final document = parse(myHtml);
return Scaffold(
appBar: AppBar(
title: const Text('html'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
const Text('document.outerHtml:'),
Text(document.outerHtml),
const SizedBox(height: 20),
const Text('document.getElementById:'),
Text(document.getElementById('dash')?.innerHtml ?? ''),
const SizedBox(height: 20),
const Text('document.querySelectorAll:'),
Text(document.querySelectorAll('div > p')[0].innerHtml),
const SizedBox(height: 20),
],
),
),
),
);
}
}
結果
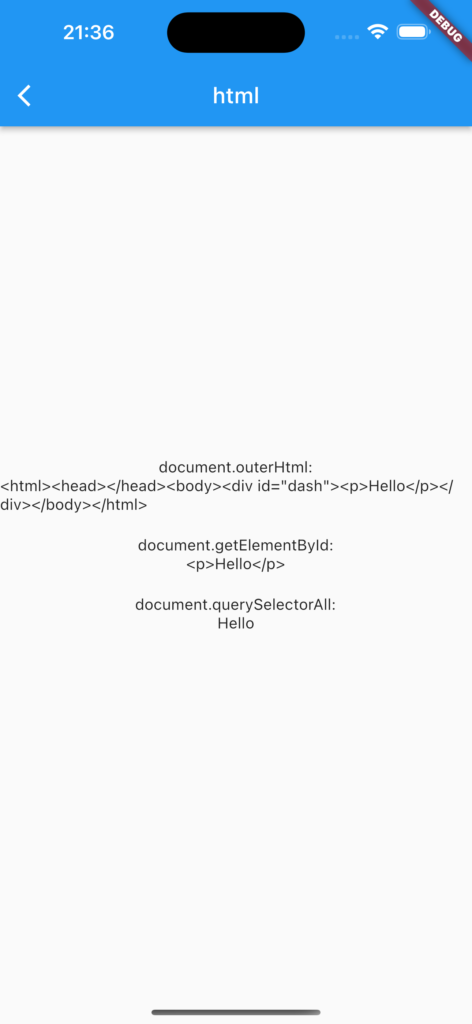
動画
公式リファレンス
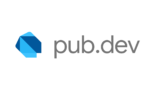
html | Dart Package
APIs for parsing and manipulating HTML content outside the browser.
#107 RefreshIndicator
RefreshIndicatorとは
リストをスワイプで更新する際に、更新中を表示することができるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage107 extends StatefulWidget {
const SamplePage107({
super.key,
});
@override
State<SamplePage107> createState() => _SamplePage107State();
}
class _SamplePage107State extends State<SamplePage107> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('RefreshIndicator'),
centerTitle: true,
),
body: SafeArea(
child: RefreshIndicator(
onRefresh: () async {
await Future<void>.delayed(const Duration(seconds: 2));
},
child: ListView.builder(
itemCount: 30,
itemBuilder: (context, index) {
return ListTile(
title: Text('index : $index'),
);
},
),
),
),
);
}
}
結果
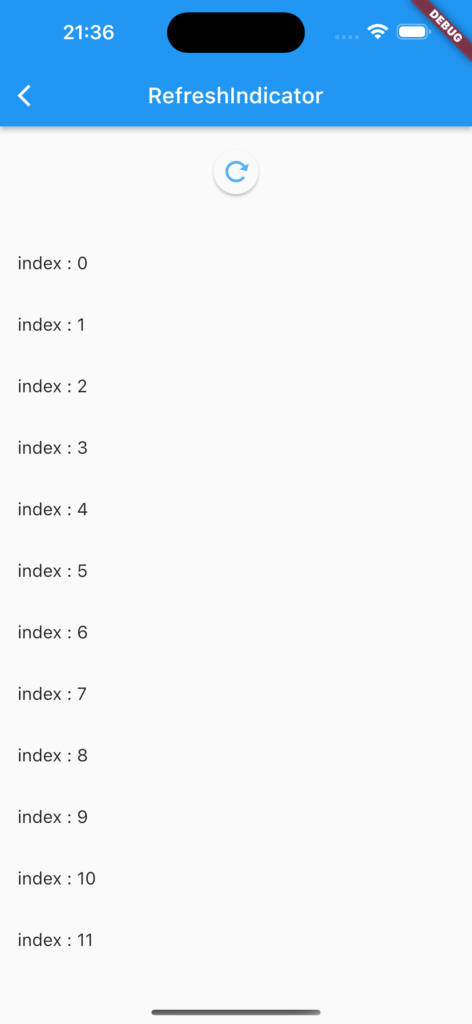
動画
公式リファレンス
RefreshIndicator class - material library - Dart API
API docs for the RefreshIndicator class from the material library, for the Dart programming language.
#108 font_awesome_flutter
font_awesome_flutterとは
優れたアイコンセットが多く入ったパッケージです。
サンプルコード
import 'package:flutter/material.dart';
import 'package:font_awesome_flutter/font_awesome_flutter.dart';
class SamplePage108 extends StatelessWidget {
const SamplePage108({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('font_awesome_flutter'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: const [
FaIcon(FontAwesomeIcons.google),
SizedBox(height: 20),
FaIcon(FontAwesomeIcons.apple),
SizedBox(height: 20),
FaIcon(FontAwesomeIcons.meta),
SizedBox(height: 20),
FaIcon(FontAwesomeIcons.amazon),
SizedBox(height: 20),
FaIcon(FontAwesomeIcons.microsoft),
],
),
),
),
);
}
}
結果
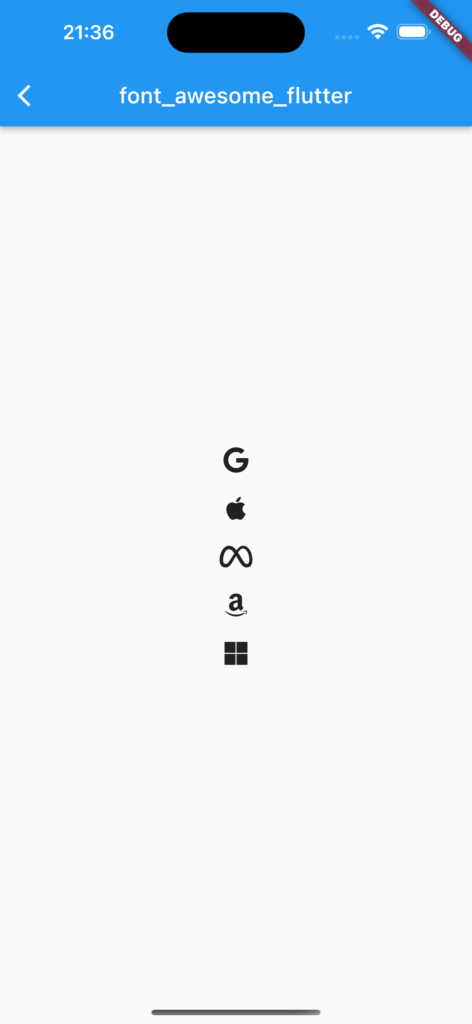
動画
公式リファレンス
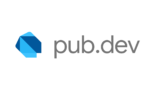
font_awesome_flutter | Flutter Package
The Font Awesome Icon pack available as Flutter Icons. Provides 2000 additional icons to use in your apps.
さいごに
このシリーズ、終りが見えない。。。
そして追いつく前にYouTubeのFlutter Widget of the Weekが終わってしまい、悲しみ。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント