はじめに
前回はFlutter Widget of the Weekの「#9 PageView」、「#10 Table」を紹介しました。
今回はその続きで「#11 SliverAppBar」、「#12 SliverList & SliverGrid」の2つです。
前回の記事はこちら
Flutter Widget of the Week
環境
- Flutter 2.8.1
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#11 SliverAppBar
SliverAppBarとは
アプリのヘッダーであるAppBarをスクロールで変化させたり、消したりしたい場合はSliverAppBarを使います。
SliverAppBarは主にCustomScrollViewやNestedScrollViewと共に利用します。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage011 extends StatelessWidget {
const SamplePage011({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: CustomScrollView(
slivers: <Widget>[
const SliverAppBar(
expandedHeight: 200,
floating: true,
flexibleSpace: FlexibleSpaceBar(
title: Text('SliverAppBar'),
background: FlutterLogo(
size: 200,
),
),
),
SliverFixedExtentList(
delegate: SliverChildBuilderDelegate((context, index) {
return Container(
alignment: Alignment.center,
color: Colors.blue[100 * (index % 9)],
child: Text('List Item $index'),
);
}),
itemExtent: 50,
),
],
),
);
}
}
結果
いつもと違う大きめのAppBarにできます。
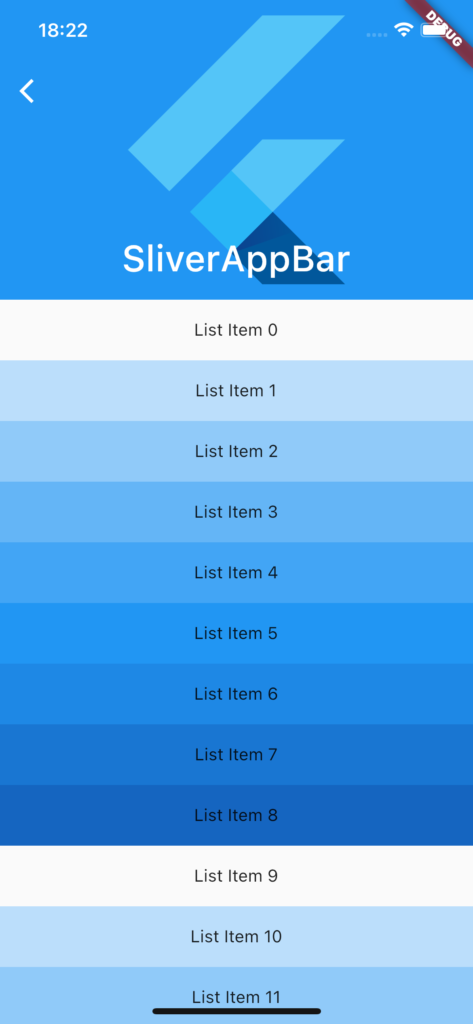
スクロールするとAppBarを完全になくすこともできます。
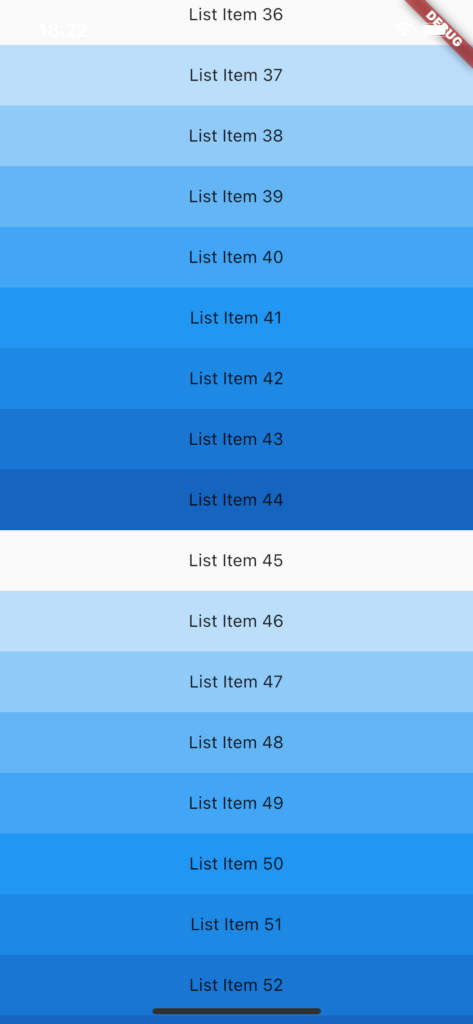
スクロールで上に戻るとAppBarが表示されます。
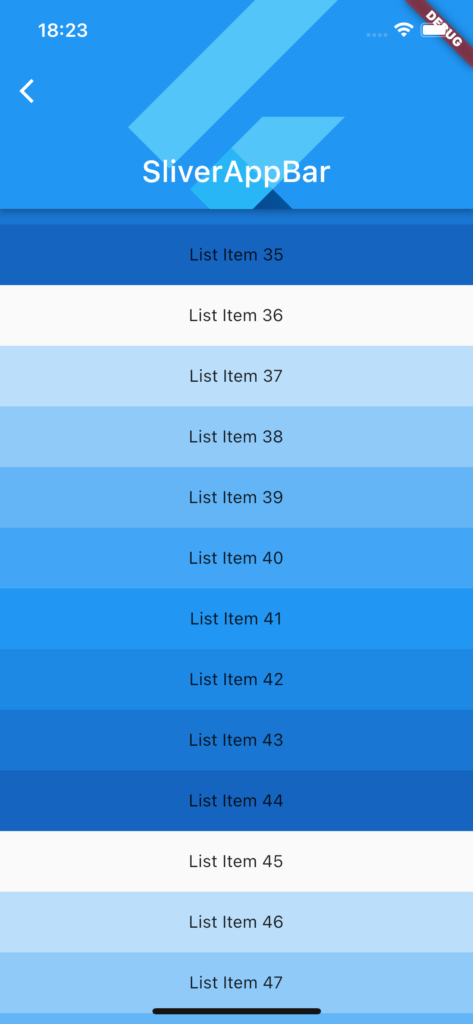
動画
公式リファレンス
SliverAppBar class - material library - Dart API
API docs for the SliverAppBar class from the material library, for the Dart programming language.
#12 SliverList & SliverGrid
SliverList & SliverGridとは
SliverList、SliverGridはCustomScrollView内でリストを表示する際に利用します。
基本的にはSliverAppBarを使う場合はSliverListやSliverGridを利用することになるかと思います。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage012 extends StatelessWidget {
const SamplePage012({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: CustomScrollView(
slivers: <Widget>[
const SliverAppBar(
expandedHeight: 200,
pinned: true,
flexibleSpace: FlexibleSpaceBar(
title: Text('SliverList & SliverGrid'),
background: FlutterLogo(
size: 200,
),
),
),
SliverList(
delegate: SliverChildBuilderDelegate((context, index) {
return Container(
alignment: Alignment.center,
color: Colors.lightBlue[100 * (index % 9)],
height: 50,
child: Text('List Item $index'),
);
}, childCount: 20),
),
SliverGrid(
delegate: SliverChildBuilderDelegate(
(context, index) {
return Container(
alignment: Alignment.center,
color: Colors.teal[100 * (index % 9)],
child: Text('Grid Item $index'),
);
},
childCount: 20,
),
gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 200,
mainAxisSpacing: 10,
crossAxisSpacing: 10,
childAspectRatio: 4,
),
),
],
),
);
}
}
結果
CustomScrollView内でSliverListを使った表現
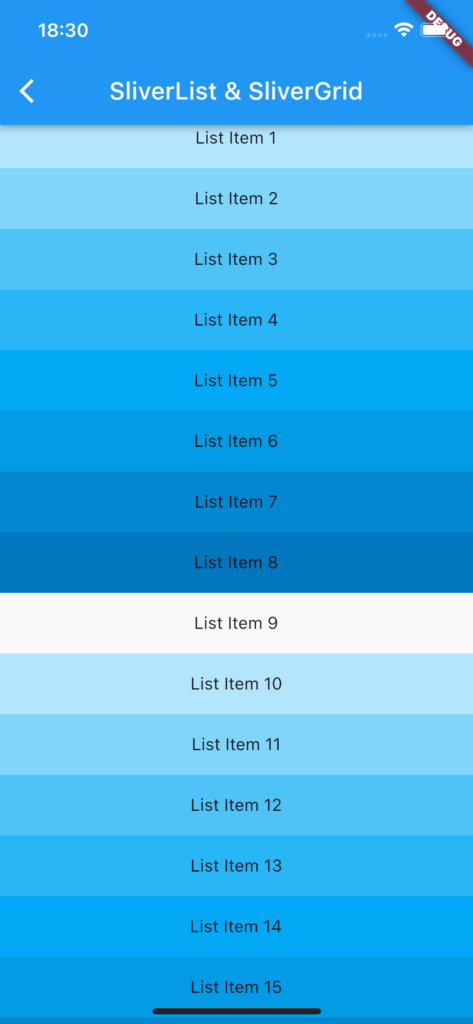
CustomScrollView内でSliverGridを使った表現
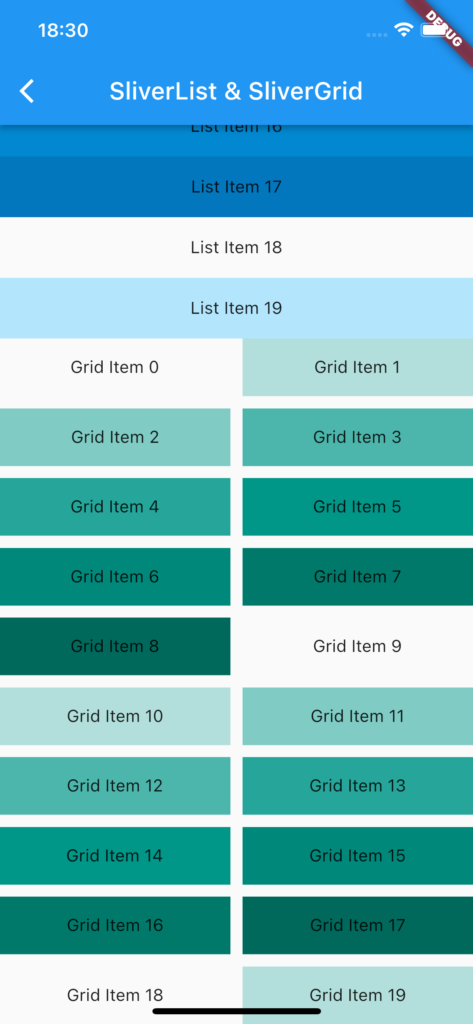
動画
公式リファレンス
SliverList class - widgets library - Dart API
API docs for the SliverList class from the widgets library, for the Dart programming language.
SliverGrid class - widgets library - Dart API
API docs for the SliverGrid class from the widgets library, for the Dart programming language.
さいごに
Sliver系はよく使うのでちゃんと覚えたいですね。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント