はじめに
UnityでUGUIのRawImageでTextureを表示する際に、Material(Shader)を使って加工する場合がよくあります。単純に表示されているモTextureをコピーする場合はRawImage.textureの値を参照すれば取得できますが、加工された状態のものをどうすれば取得できるのか調べてみました。
環境
- Unity 2021.1.1f1
サンプルコード
やり方は結構簡単でした、TextureをRenderTextureにMaterialを反映させつつ書き込み、書き込んだRenderTextureを取り出して終わりです。
SampleSceneController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class SampleSceneController : MonoBehaviour
{
[SerializeField] private Button copyButton;
[SerializeField] private RawImage inputRawImage;
[SerializeField] private RawImage outputRawImage;
// Start is called before the first frame update
void Start()
{
copyButton.onClick.AddListener(OnClickedCopyButton);
}
private void OnClickedCopyButton()
{
StartCoroutine(Copy());
}
private IEnumerator Copy()
{
Texture2D texture = inputRawImage.texture as Texture2D;
var renderTexture = new RenderTexture(texture.width, texture.height, 0);
Graphics.Blit(texture, renderTexture, inputRawImage.material);
yield return new WaitForEndOfFrame();
Texture2D outputTexture = new Texture2D(renderTexture.width, renderTexture.height, texture.format, false);
RenderTexture.active = renderTexture;
outputTexture.ReadPixels(new Rect(0, 0, renderTexture.width, renderTexture.height), 0, 0, false);
outputTexture.Apply();
yield return new WaitForEndOfFrame();
RenderTexture.active = null;
RenderTexture.DestroyImmediate(renderTexture);
outputRawImage.texture = outputTexture;
}
}
動作確認
加工するマテリアルを用意
今回は赤くするMaterialを適当に作ります。
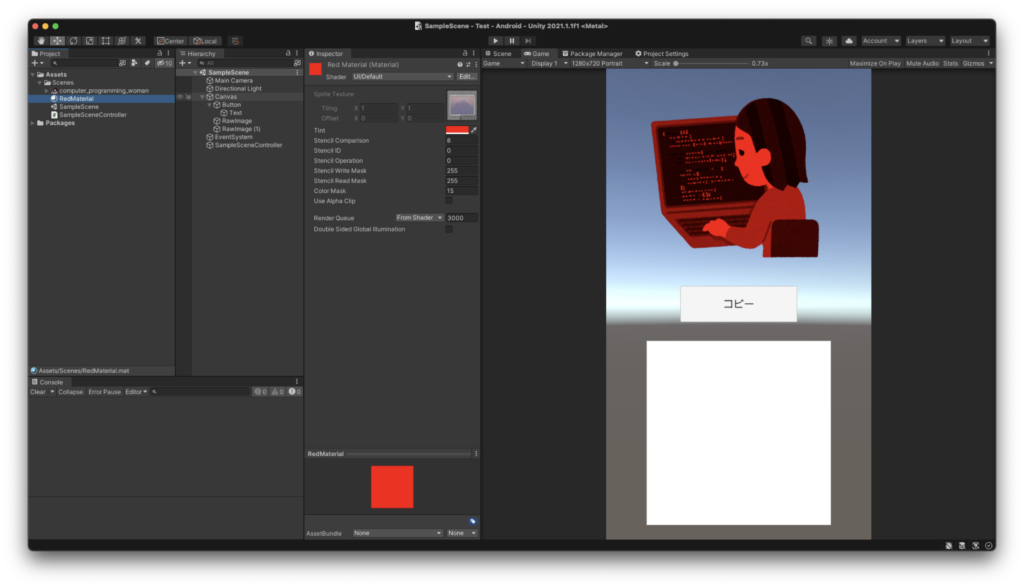
マテリアルを反映したコピー前のRawImage
通常の画像がMaterialによって赤くなっているのがわかります。
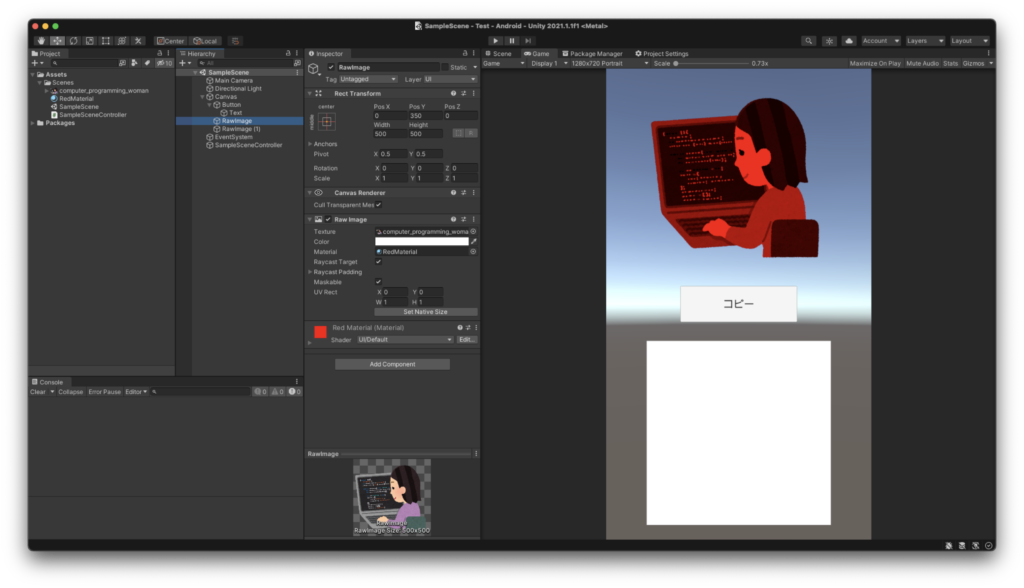
これをサンプルコードでコピーすると。。。
マテリアルなしのRawImageに反映される
RawImageにMaterialを反映したTextureが正しくコピーされました!
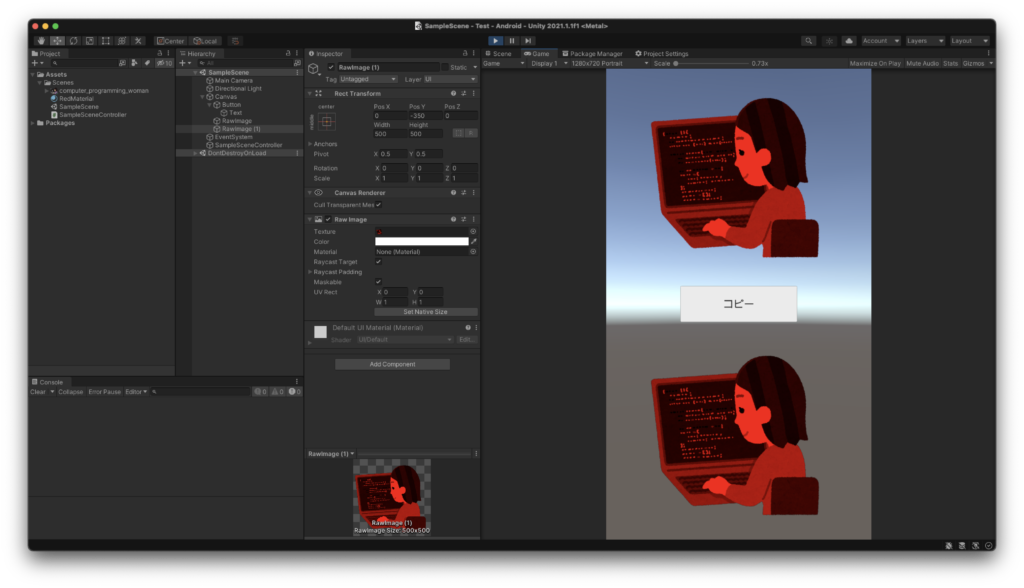
さいごに
自分はこのコードを使う場面があったのですが、みなさんが使う場面があるかは疑問ですね。。。
コメント