はじめに
前回はFlutter Widget of the Weekの「#73 ClipPath」、「#74 CircularProgressIndicator,LinearProgressIndicator」、「#75 Divider」を紹介しました。
今回はその続きで「#76 IgnorePointer」、「#77 CupertinoActivityIndicator」、「#78 ClipOval」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.3.1
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#76 IgnorePointer
IgnorePointerとは
IgnorePointerのchildに設定したウィジェットのサブツリーのイベント(タップ、スクロール等)を一括で無効化できるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage076 extends StatefulWidget {
const SamplePage076({
super.key,
});
@override
State<SamplePage076> createState() => _SamplePage076State();
}
class _SamplePage076State extends State<SamplePage076> {
bool _ignore = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('IgnorePointer'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: [
IgnorePointer(
ignoring: _ignore,
child: ElevatedButton(
onPressed: () {},
child: _ignore ? const Text('押せない') : const Text('押せる'),
),
),
const SizedBox(height: 30),
ElevatedButton(
onPressed: () {},
child: const Text('押せる'),
)
],
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_ignore = !_ignore;
});
},
child: _ignore
? const Icon(Icons.check_box_outline_blank)
: const Icon(Icons.check_box),
),
);
}
}
結果
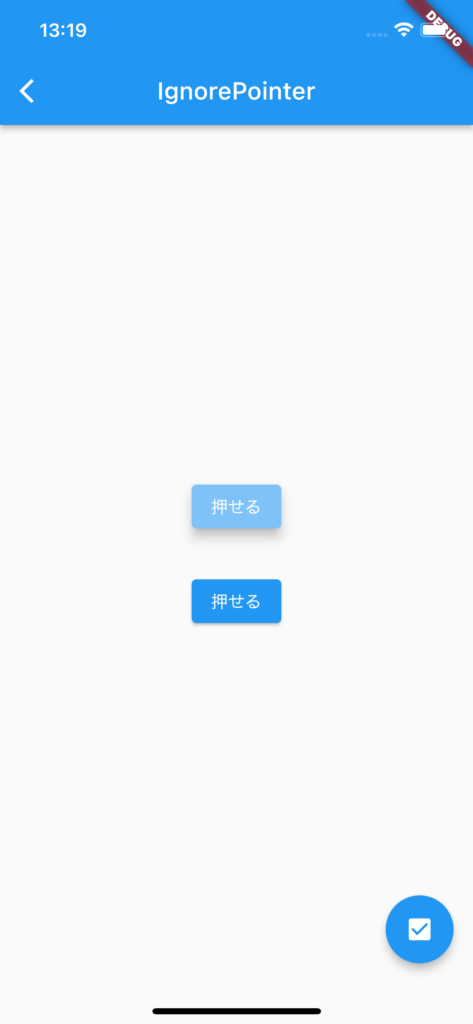
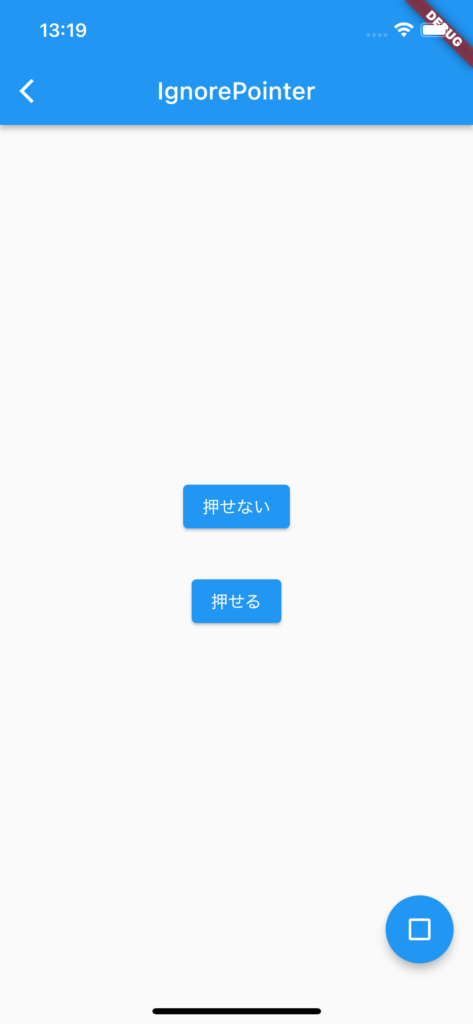
動画
公式リファレンス
IgnorePointer class - widgets library - Dart API
API docs for the IgnorePointer class from the widgets library, for the Dart programming language.
#77 CupertinoActivityIndicator
CupertinoActivityIndicatorとは
進捗状況や実行中であることを示すのにうってつけのウィジェットです。
以前紹介したCircularProgressIndicatorとは違い、iOSに寄せたデザインになっています。
サンプルコード
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class SamplePage077 extends StatefulWidget {
const SamplePage077({
super.key,
});
@override
State<SamplePage077> createState() => _SamplePage077State();
}
class _SamplePage077State extends State<SamplePage077> {
late final Future<String> _future;
@override
void initState() {
super.initState();
_future = _loading();
}
Future<String> _loading() async {
await Future<void>.delayed(
const Duration(seconds: 3),
);
return 'Complete';
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('CupertinoActivityIndicator'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: FutureBuilder<String>(
future: _future,
builder: (context, snapshot) {
if (snapshot.connectionState != ConnectionState.done) {
return const CupertinoActivityIndicator();
}
if (snapshot.hasError) {
return const Icon(
Icons.error,
color: Colors.red,
);
}
return Text(snapshot.data ?? '');
},
),
),
),
);
}
}
結果
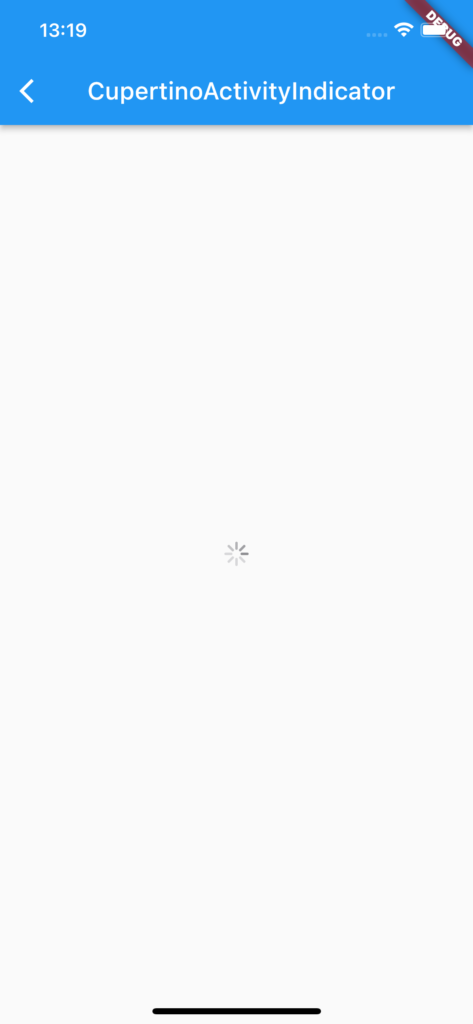
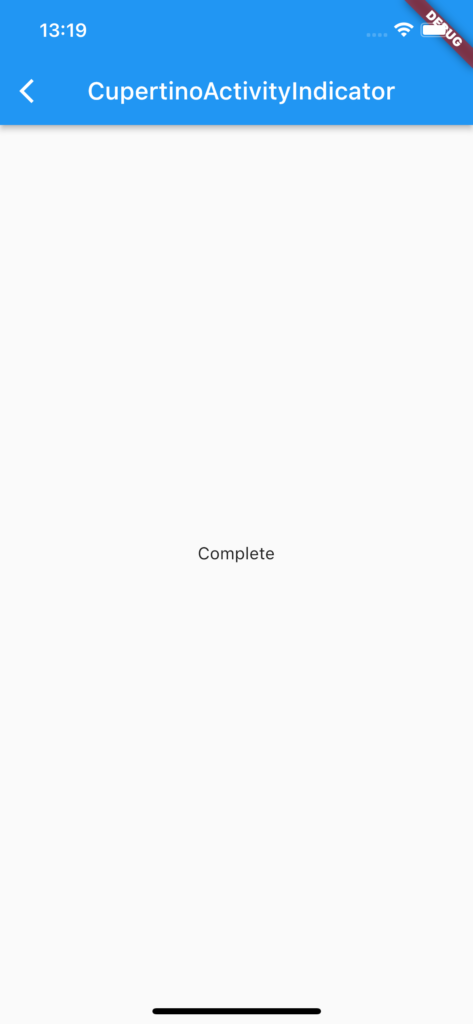
動画
公式リファレンス
CupertinoActivityIndicator class - cupertino library - Dart API
API docs for the CupertinoActivityIndicator class from the cupertino library, for the Dart programming language.
#78 ClipOval
ClipOvalとは
子ウィジェットを円形や楕円形にくり抜くことができるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage078 extends StatelessWidget {
const SamplePage078({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ClipOval'),
centerTitle: true,
),
body: ColoredBox(
color: Colors.grey,
child: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ClipOval(
child: Image.asset(
'assets/genba_neko.png',
width: 128,
height: 128,
),
),
const SizedBox(height: 30),
ClipOval(
clipper: _SamplePage078Clipper(),
child: Image.asset(
'assets/genba_neko.png',
width: 128,
height: 128,
),
),
],
),
),
),
),
);
}
}
class _SamplePage078Clipper extends CustomClipper<Rect> {
@override
Rect getClip(Size size) {
return const Rect.fromLTWH(0, 0, 100, 50);
}
@override
bool shouldReclip(covariant CustomClipper<Rect> oldClipper) {
return false;
}
}
結果
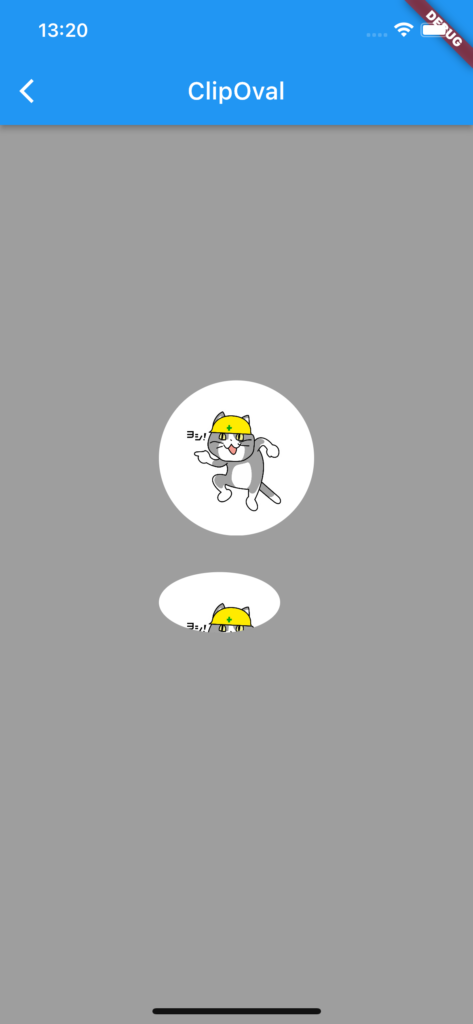
動画
公式リファレンス
ClipOval class - widgets library - Dart API
API docs for the ClipOval class from the widgets library, for the Dart programming language.
さいごに
CupertinoActivityIndicator使うことはないな。。。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント