はじめに
前回はFlutter Widget of the Weekの「#6 FutureBuilder」、「#7 FadeTransition」、「#8 FloatingActionButton」を紹介しました。
今回はその続きで「#9 PageView」、「#10 Table」の2つです。
前回の記事はこちら
Flutter Widget of the Week
環境
- Flutter 2.8.1
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#9 PageView
PageViewとは
スマートフォン特有の操作であるスワイプで切り替える画面を作りたいときに使うのがPageViewです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage009 extends StatefulWidget {
const SamplePage009({
Key? key,
}) : super(key: key);
@override
_SamplePage009State createState() => _SamplePage009State();
}
class _SamplePage009State extends State<SamplePage009> {
final _pageController = PageController(initialPage: 0);
bool _toggle = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('PageView'),
),
body: SafeArea(
child: Column(
children: [
Center(
child: Text('${_toggle ? '上下' : '左右'}スワイプ'),
),
Expanded(
child: PageView(
controller: _pageController,
scrollDirection: _toggle ? Axis.vertical : Axis.horizontal,
children: [
Container(
color: Colors.red,
child: const Center(
child: Text('Page 1'),
),
),
Container(
color: Colors.blue,
child: const Center(
child: Text('Page 2'),
),
),
Container(
color: Colors.green,
child: const Center(
child: Text('Page 3'),
),
),
],
),
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_toggle = !_toggle;
});
},
child: const Icon(Icons.loop),
),
);
}
}
結果
横スワイプで画面切り替えができ、
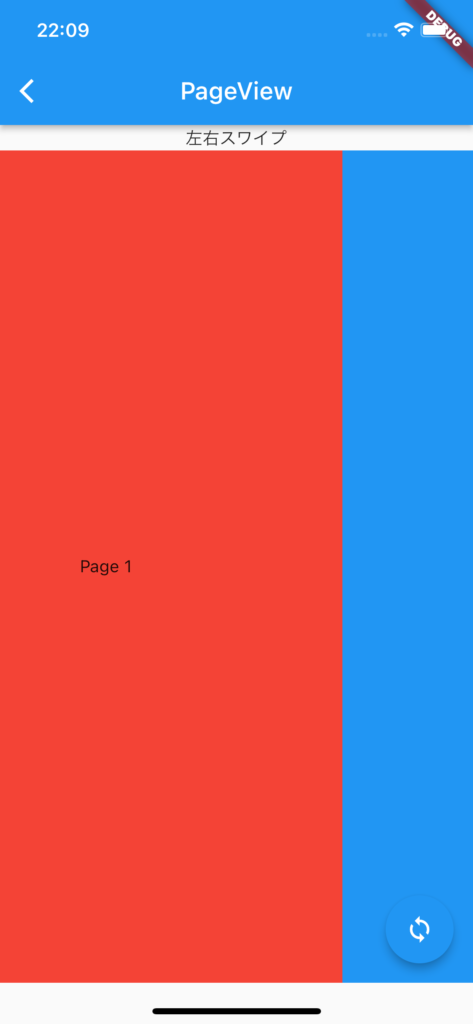
縦スワイプでも画面の切り替えができました。
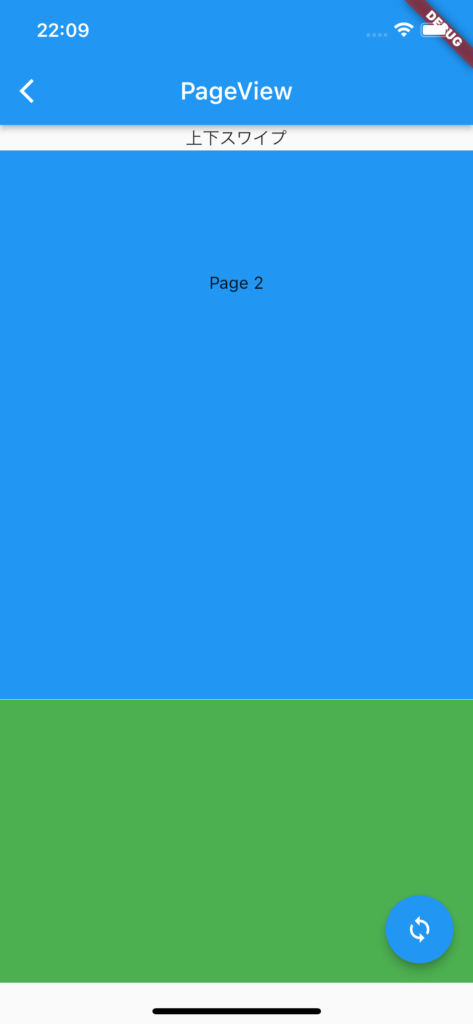
動画
公式リファレンス
PageView class - widgets library - Dart API
API docs for the PageView class from the widgets library, for the Dart programming language.
#10 Table
Tableとは
スクロールがいらないグリッド表示の画面を作るのに適しているのがTableです。
わざわざColumnやRowを組み合わせて使う必要はありません。
サンプルコード
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class SamplePage010 extends StatelessWidget {
const SamplePage010({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Table'),
),
body: SafeArea(
child: Center(
child: Table(
border: TableBorder.all(),
defaultVerticalAlignment: TableCellVerticalAlignment.middle,
children: const [
TableRow(
children: [
_SamplePage010WideWidget(),
_SamplePage010MediumWidget(),
_SamplePage010MediumWidget(),
],
),
TableRow(
children: [
_SamplePage010MediumWidget(),
_SamplePage010NarrowWidget(),
_SamplePage010MediumWidget(),
],
),
],
),
),
),
);
}
}
class _SamplePage010WideWidget extends StatelessWidget {
const _SamplePage010WideWidget();
@override
Widget build(BuildContext context) {
return Container(
width: 100,
height: 200,
color: Colors.blueAccent,
);
}
}
class _SamplePage010MediumWidget extends StatelessWidget {
const _SamplePage010MediumWidget();
@override
Widget build(BuildContext context) {
return Container(
width: 100,
height: 100,
color: Colors.lightBlueAccent,
);
}
}
class _SamplePage010NarrowWidget extends StatelessWidget {
const _SamplePage010NarrowWidget();
@override
Widget build(BuildContext context) {
return Container(
width: 100,
height: 50,
color: Colors.orangeAccent,
);
}
}
結果
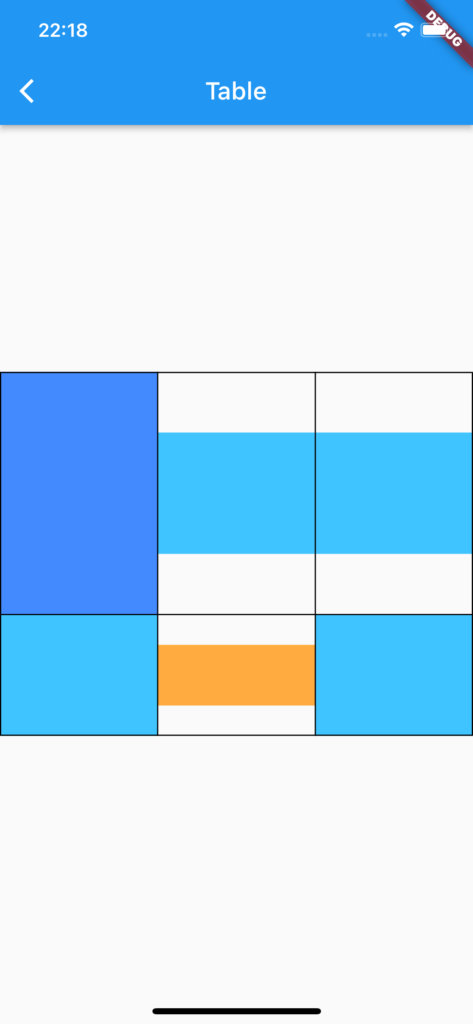
動画
公式リファレンス
Table class - widgets library - Dart API
API docs for the Table class from the widgets library, for the Dart programming language.
さいごに
Tableの使いどきがよくわからない。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント