はじめに
前回はFlutter Widget of the Weekの「#40 Placeholder」、「#41 RichText」、「#42 ReorderableListView」を紹介しました。
今回はその続きで「#43 AnimatedSwitcher」、「#44 AnimatedPositioned」、「#45 AnimatedPadding」の3つです。
前回の記事はこちら
Flutter Widget of the Week
環境
- Flutter 3.0.2
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#43 AnimatedSwitcher
AnimatedSwitcherとは
複数のウィジェットをアニメーションを使って切り替えるためのウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage043 extends StatefulWidget {
const SamplePage043({
super.key,
});
@override
State<SamplePage043> createState() => _SamplePage043State();
}
class _SamplePage043State extends State<SamplePage043> {
int _count = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('AnimatedSwitcher'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: AnimatedSwitcher(
duration: const Duration(milliseconds: 300),
transitionBuilder: (Widget child, Animation<double> animation) {
return ScaleTransition(scale: animation, child: child);
},
child: Text(
_count.toString(),
key: ValueKey<int>(_count),
style: Theme.of(context).textTheme.headline2,
),
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_count += 1;
});
},
child: const Icon(Icons.add),
),
);
}
}
結果
カウントアップと合わせてスケーリングしながら表示が切り替わっていきます。
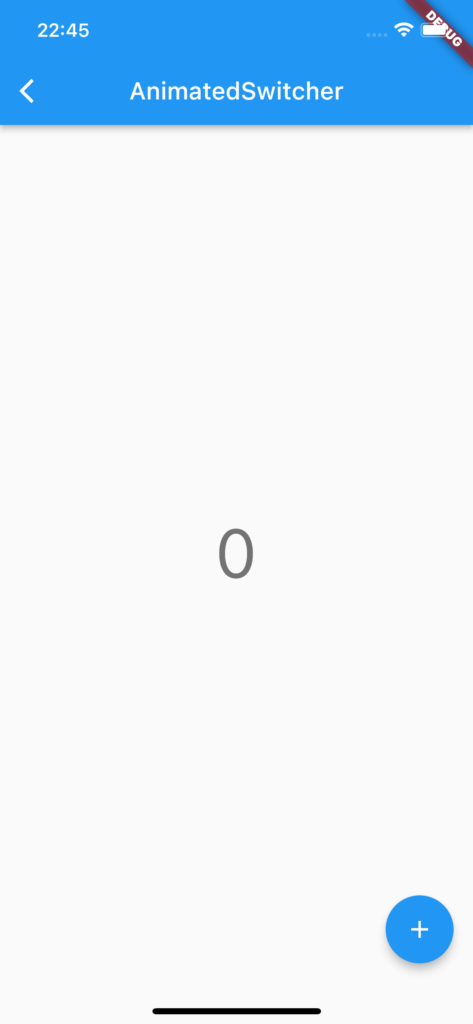
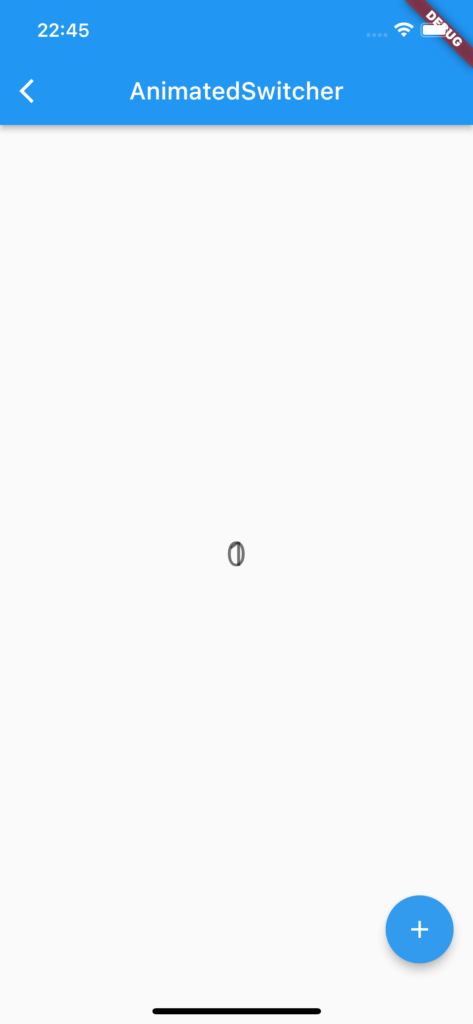
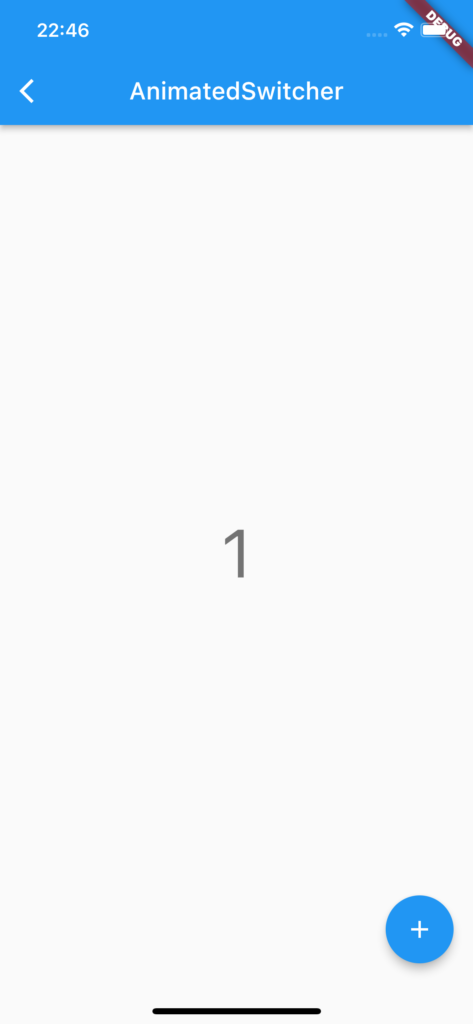
動画
公式リファレンス
AnimatedSwitcher class - widgets library - Dart API
API docs for the AnimatedSwitcher class from the widgets library, for the Dart programming language.
#44 AnimatedPositioned
AnimatedPositionedとは
Sliderのような移動するアニメーションを簡単に作れるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage044 extends StatefulWidget {
const SamplePage044({
super.key,
});
@override
State<SamplePage044> createState() => _SamplePage044State();
}
class _SamplePage044State extends State<SamplePage044> {
bool _show = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('AnimatedPositioned'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Container(
width: 300,
height: 400,
color: Colors.lightBlue,
child: Stack(
children: [
const Center(
child: Text(
'Hello!!',
style: TextStyle(
color: Colors.white,
fontSize: 30,
),
),
),
AnimatedPositioned(
duration: const Duration(milliseconds: 200),
right: 50,
bottom: _show ? 225 : 175,
child: GestureDetector(
child: Container(
width: 200,
height: 50,
color: Colors.blue[900],
child: const Icon(
Icons.touch_app,
color: Colors.white,
),
),
onTap: () {
setState(() {
_show = !_show;
});
},
),
),
],
),
),
),
),
);
}
}
結果
タップするとスライドしてHello!が表示されます。
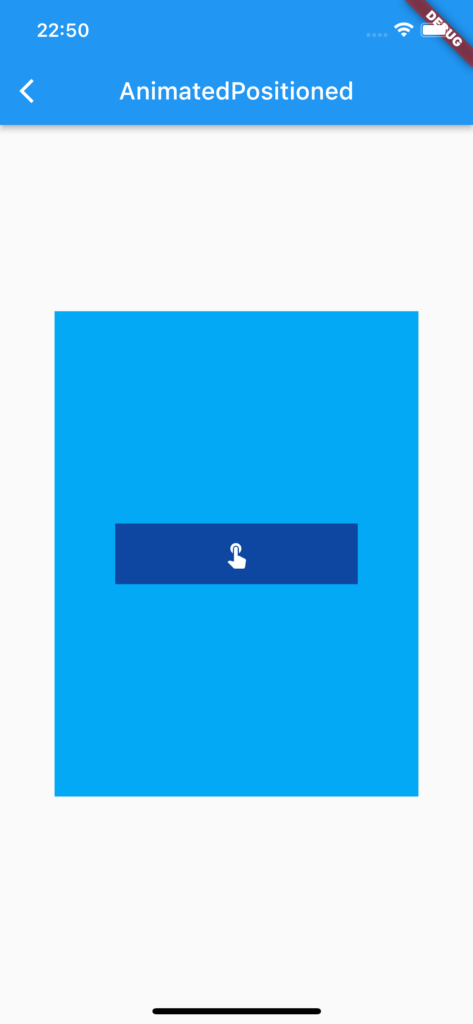
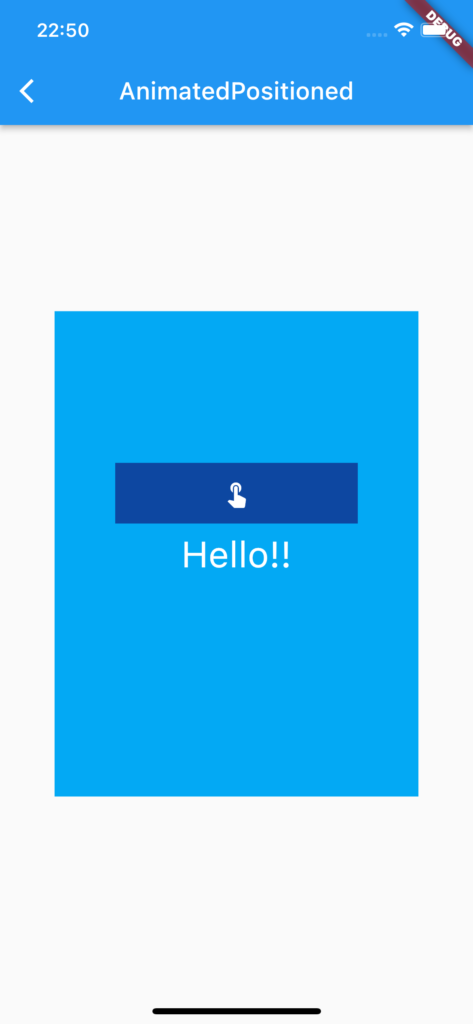
動画
公式リファレンス
AnimatedPositioned class - widgets library - Dart API
API docs for the AnimatedPositioned class from the widgets library, for the Dart programming language.
#45 AnimatedPadding
AnimatedPaddingとは
ウィジェットのPaddingを動的に変更した際にアニメーションさせることができるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage045 extends StatefulWidget {
const SamplePage045({
super.key,
});
@override
State<SamplePage045> createState() => _SamplePage045State();
}
class _SamplePage045State extends State<SamplePage045> {
double _padding = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('AnimatedPositioned'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: ColoredBox(
color: Colors.blue,
child: AnimatedPadding(
duration: const Duration(milliseconds: 300),
padding: EdgeInsets.all(_padding),
child: const Icon(
Icons.padding,
color: Colors.white,
),
),
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_padding += 5.0;
});
},
child: const Icon(Icons.add),
),
);
}
}
結果
ボタンを押すとアニメーションしながらPaddingが広がっていきます。
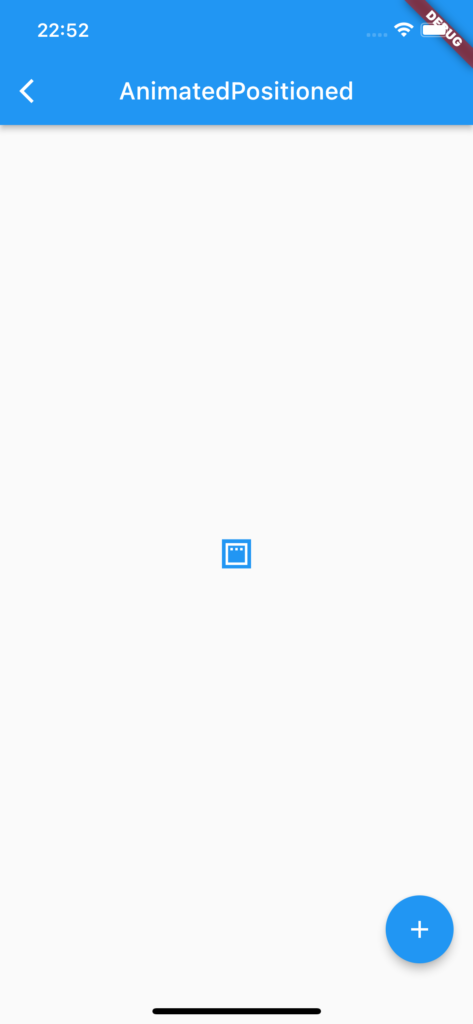
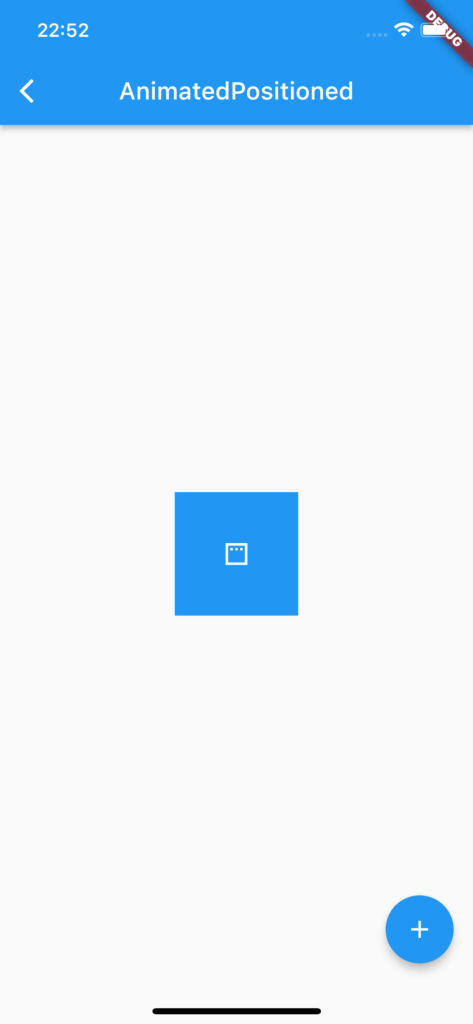
動画
公式リファレンス
AnimatedPadding class - widgets library - Dart API
API docs for the AnimatedPadding class from the widgets library, for the Dart programming language.
さいごに
今回はすべてアニメーション系のウィジェットでした。
普段アニメーション系のウィジェットは使わないので新鮮でした。
みなさんはアニメーションウィジェットは得意ですか?
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント