はじめに
前回はFlutter Widget of the Weekの「#151 firebase_auth」、「#152 fl_chart」、「#153 gap」を紹介しました。
今回はその続きで「#154 OverlayPortal」、「#155 DropdownMenu」、「#156 feedback」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.19.6
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#154 OverlayPortal
OverlayPortalとは?
既存のWidgetの上にWidgetを表示できるWidgetです。
画面上のどこにでも表示することが出来ます。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage154 extends StatefulWidget {
const SamplePage154({
super.key,
});
@override
State<SamplePage154> createState() => _SamplePage154State();
}
class _SamplePage154State extends State<SamplePage154> {
final _controller = OverlayPortalController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('OverlayPortal'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
ElevatedButton(
onPressed: _controller.toggle,
child: OverlayPortal(
controller: _controller,
overlayChildBuilder: (context) {
return const Positioned(
top: 200,
right: 200,
child: Text("I'm an overlay!"),
);
},
child: const Text('Press Me!'),
),
),
],
),
),
),
);
}
}
結果
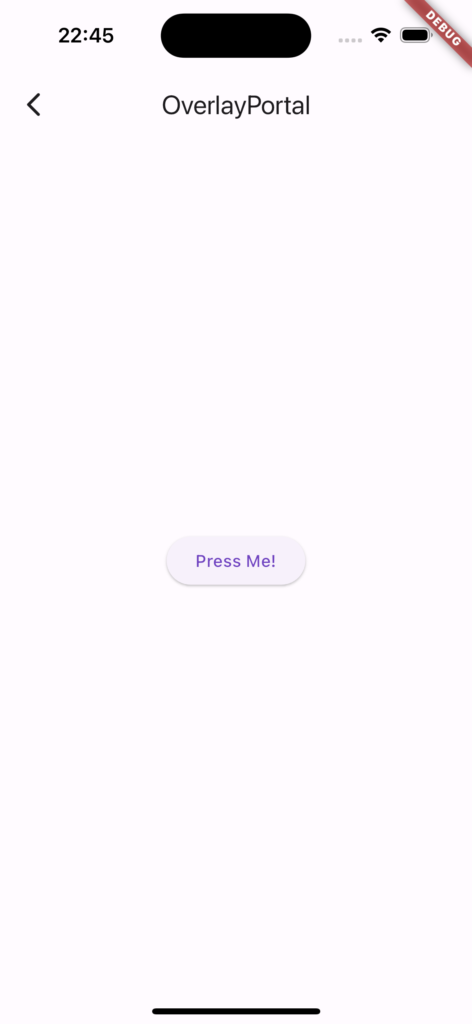
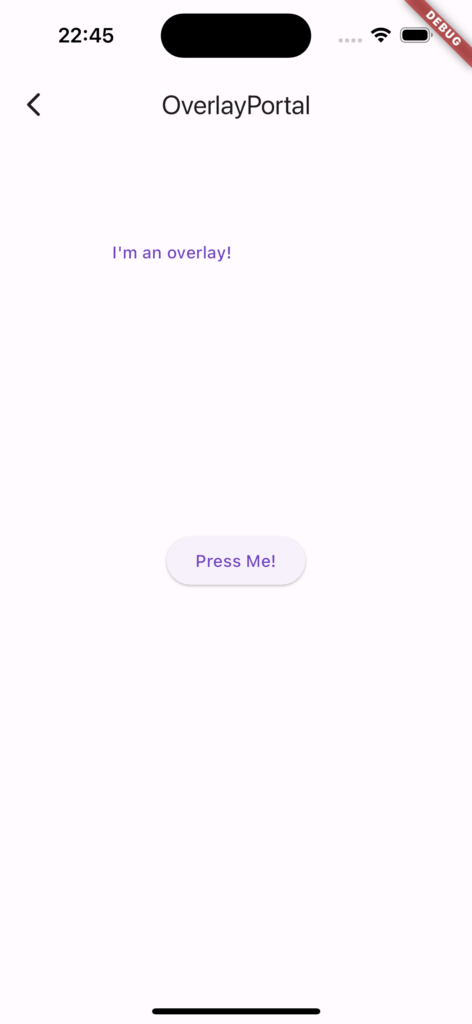
動画
公式リファレンス
OverlayPortal class - widgets library - Dart API
API docs for the OverlayPortal class from the widgets library, for the Dart programming language.
#155 DropdownMenu
DropdownMenuとは?
Widget名の通り、ドロップダウンメニューを表現できるWidgetです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage155 extends StatefulWidget {
const SamplePage155({
super.key,
});
@override
State<SamplePage155> createState() => _SamplePage155State();
}
class _SamplePage155State extends State<SamplePage155> {
var _backgroundColor = Colors.red[400];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('DropdownMenu'),
centerTitle: true,
),
backgroundColor: _backgroundColor,
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
DropdownMenu(
initialSelection: _backgroundColor,
dropdownMenuEntries: [
DropdownMenuEntry(
value: Colors.red[400],
label: 'Red',
),
DropdownMenuEntry(
value: Colors.blue[400],
label: 'Blue',
),
DropdownMenuEntry(
value: Colors.purple[400],
label: 'Purple',
),
DropdownMenuEntry(
value: Colors.green[400],
label: 'Green',
),
DropdownMenuEntry(
value: Colors.brown[400],
label: 'Brown',
),
],
onSelected: (value) {
setState(() {
_backgroundColor = value;
});
},
),
],
),
),
),
);
}
}
結果
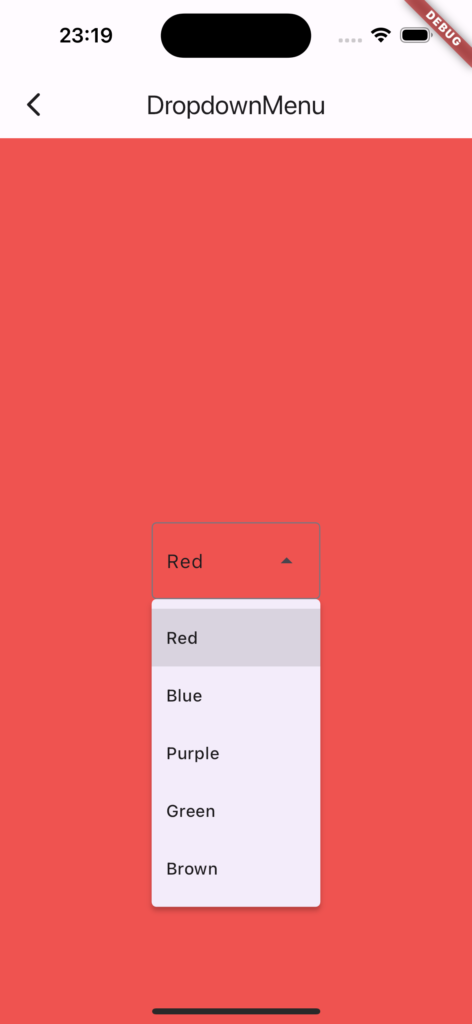
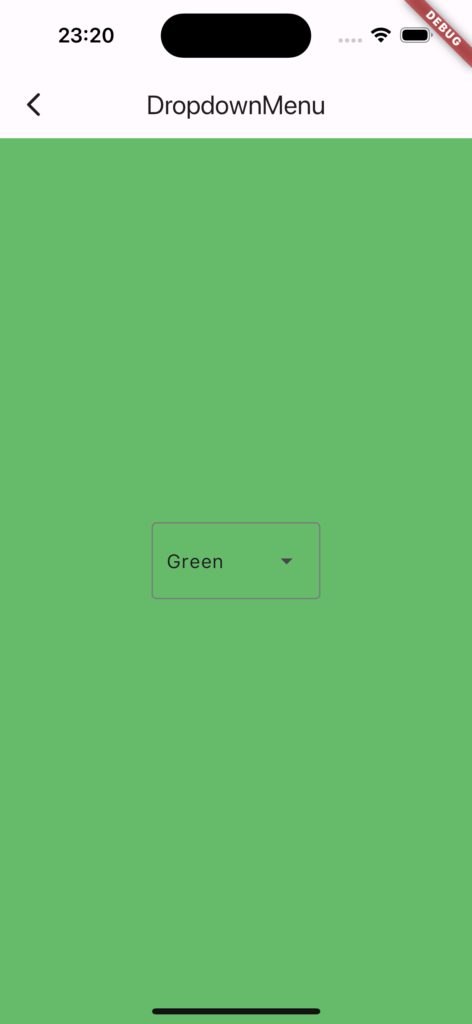
動画
公式リファレンス
DropdownMenu class - material library - Dart API
API docs for the DropdownMenu class from the material library, for the Dart programming language.
#156 feedback
feedbackとは?
ユーザーからのフィードバックを簡単に実装できてしまうパッケージです。
テキストに画像付与することができて、さらにフリーハンドで絵を書くことも出来ます。
サンプルコード
void main() async {
WidgetsFlutterBinding.ensureInitialized();
runApp(
const BetterFeedback(
child: MyApp(),
),
);
}
import 'package:feedback/feedback.dart';
import 'package:flutter/material.dart';
class SamplePage156 extends StatefulWidget {
const SamplePage156({
super.key,
});
@override
State<SamplePage156> createState() => _SamplePage156State();
}
class _SamplePage156State extends State<SamplePage156> {
int _counter = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('feedback'),
centerTitle: true,
actions: [
IconButton(
onPressed: () async {
BetterFeedback.of(context).show(
(feedback) async {
// ここでスクリーンショットやメッセージを送信する.
},
);
},
icon: const Icon(
Icons.feedback_outlined,
),
),
],
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headlineMedium,
),
],
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_counter++;
});
},
child: const Icon(Icons.add_outlined),
),
);
}
}
結果
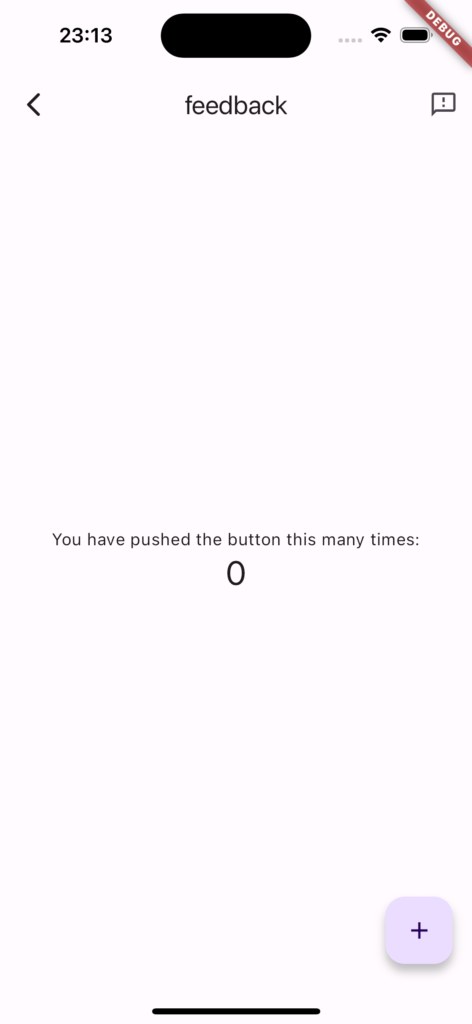
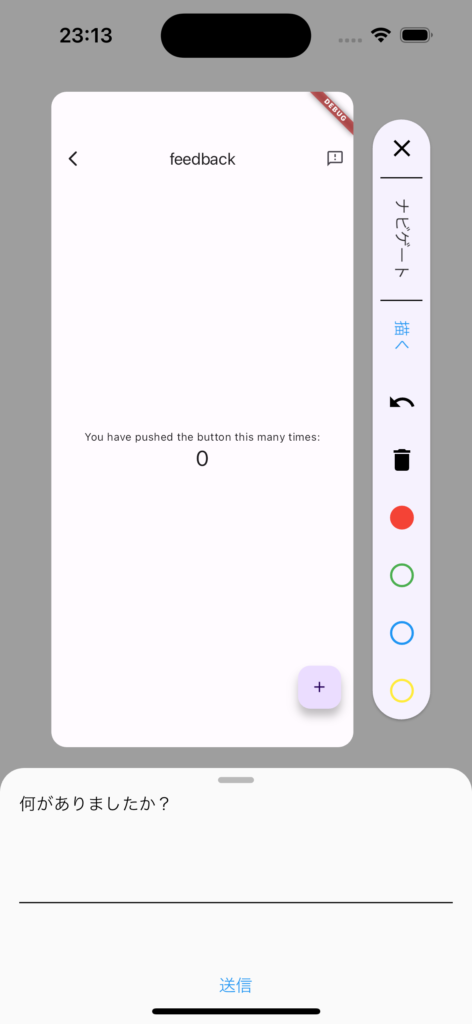
動画
公式リファレンス
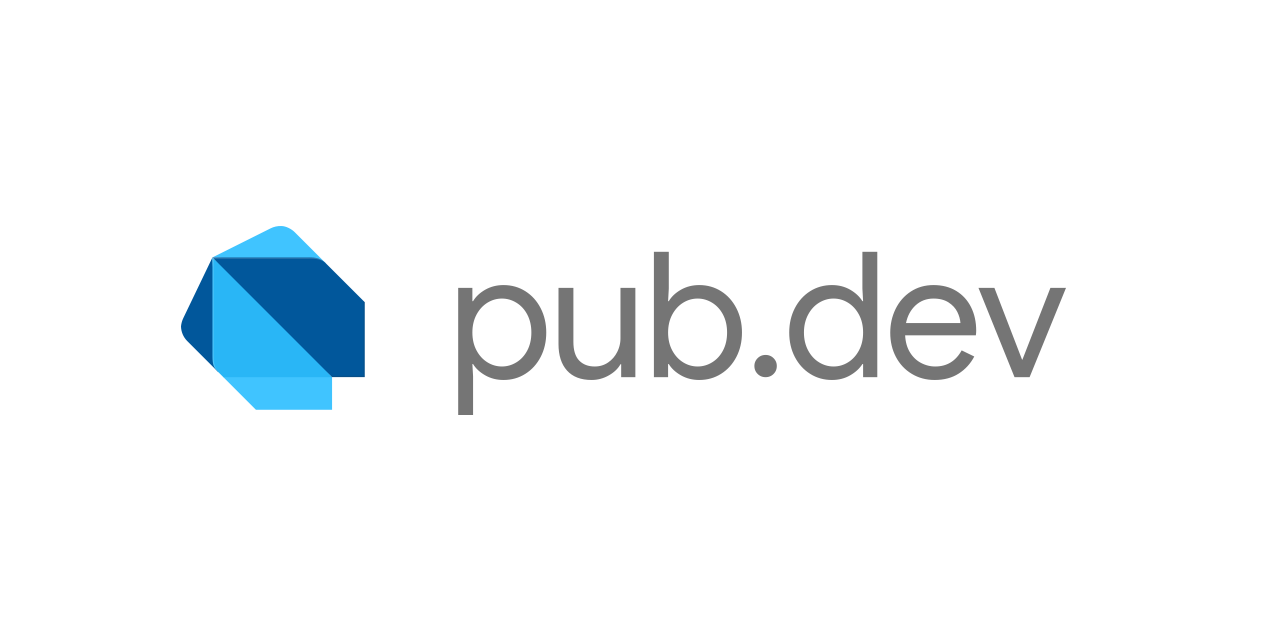
feedback | Flutter package
A Flutter package for getting better feedback. It allows the user to give interactive feedback directly in the app.
さいごに
サボってしまった。。。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント