はじめに
前回はFlutter Widget of the Weekの「#58 AlertDialog,CupertinoAlertDialog」、「#59 AnimatedCrossFade」、「#60 DraggableScrollableSheet」を紹介しました。
今回はその続きで「#61 ColorFiltered」、「#62 ToggleButtons」、「#63 CupertinoActionSheet」の3つです。
前回の記事はこちら
Flutter Widget of the Week
環境
- Flutter 3.0.5
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#61 ColorFiltered
ColorFilteredとは
画像やウィジェット全体に色味を追加したい場合にこのウィジェットを利用します。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage061 extends StatelessWidget {
const SamplePage061({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ColorFiltered'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: const [
ColorFiltered(
colorFilter: ColorFilter.mode(
Colors.grey,
BlendMode.color,
),
child: FlutterLogo(
size: 128,
),
),
SizedBox(height: 20),
FlutterLogo(
size: 128,
),
],
),
),
),
);
}
}
結果
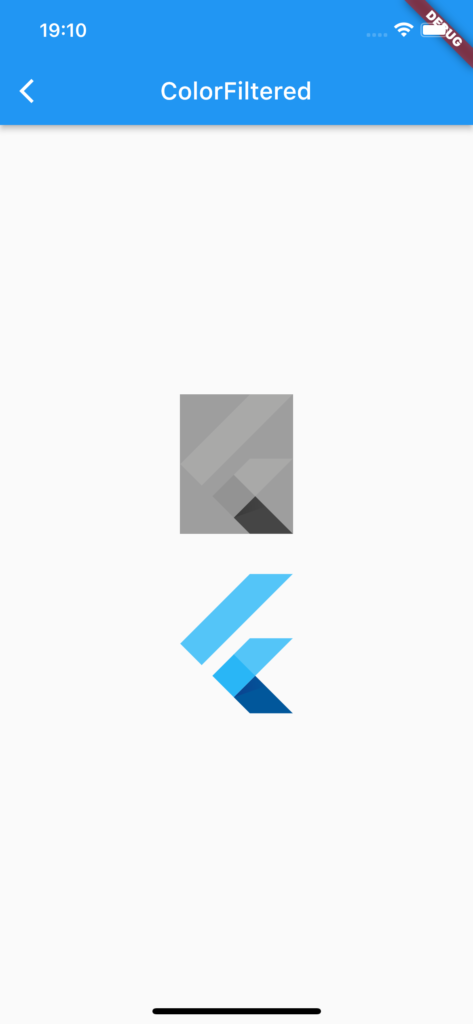
動画
公式リファレンス
ColorFiltered class - widgets library - Dart API
API docs for the ColorFiltered class from the widgets library, for the Dart programming language.
#62 ToggleButtons
ToggleButtonsとは
横並びのトグルボタンを簡単に実装できるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage062 extends StatefulWidget {
const SamplePage062({
super.key,
});
@override
State<SamplePage062> createState() => _SamplePage062State();
}
class _SamplePage062State extends State<SamplePage062> {
final _isSelected = <bool>[
false,
false,
false,
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ToggleButtons'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: ToggleButtons(
isSelected: _isSelected,
onPressed: (index) {
setState(() {
_isSelected[index] = !_isSelected[index];
});
},
children: const [
Icon(Icons.home),
Icon(Icons.drive_eta),
Icon(Icons.flutter_dash),
],
),
),
),
);
}
}
結果
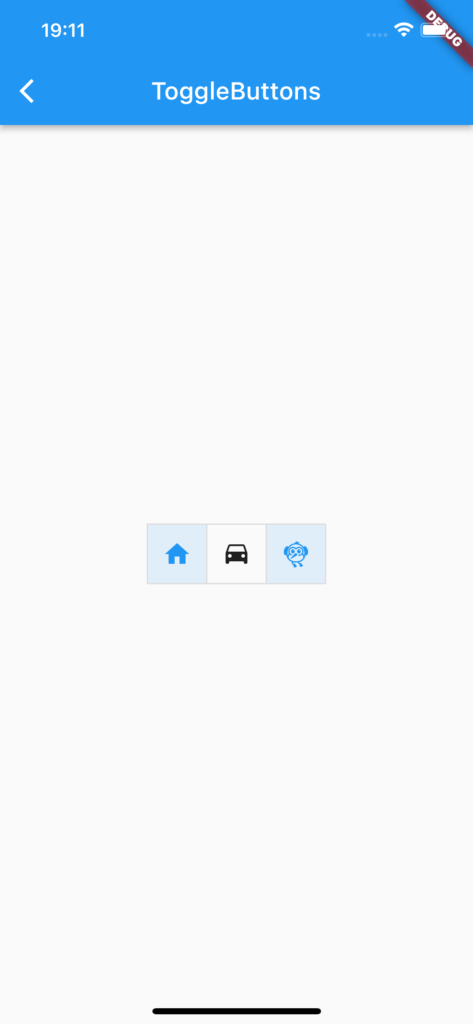
動画
公式リファレンス
ToggleButtons class - material library - Dart API
API docs for the ToggleButtons class from the material library, for the Dart programming language.
#63 CupertinoActionSheet
CupertinoActionSheetとは
iOSスタイルのActionSheetを表示したい場合に利用するウィジェットです。
サンプルコード
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class SamplePage063 extends StatefulWidget {
const SamplePage063({
super.key,
});
@override
State<SamplePage063> createState() => _SamplePage063State();
}
class _SamplePage063State extends State<SamplePage063> {
String _text = '';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('CupertinoActionSheet'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Text(_text),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
final text = await showCupertinoModalPopup<String>(
context: context,
builder: (context) {
return CupertinoActionSheet(
title: const Text('タイトル'),
message: const Text('メッセージ'),
actions: [
CupertinoActionSheetAction(
onPressed: () {
Navigator.of(context).pop('Hello');
},
isDefaultAction: true,
child: const Text('Hello'),
),
CupertinoActionSheetAction(
onPressed: () {
Navigator.of(context).pop('Goodbye');
},
child: const Text('Goodbye'),
)
],
cancelButton: CupertinoActionSheetAction(
onPressed: () {
Navigator.of(context).pop();
},
isDestructiveAction: true,
child: const Text('キャンセル'),
),
);
},
);
if (text == null) {
return;
}
setState(() {
_text = text;
});
},
child: const Icon(Icons.open_in_new),
),
);
}
}
結果
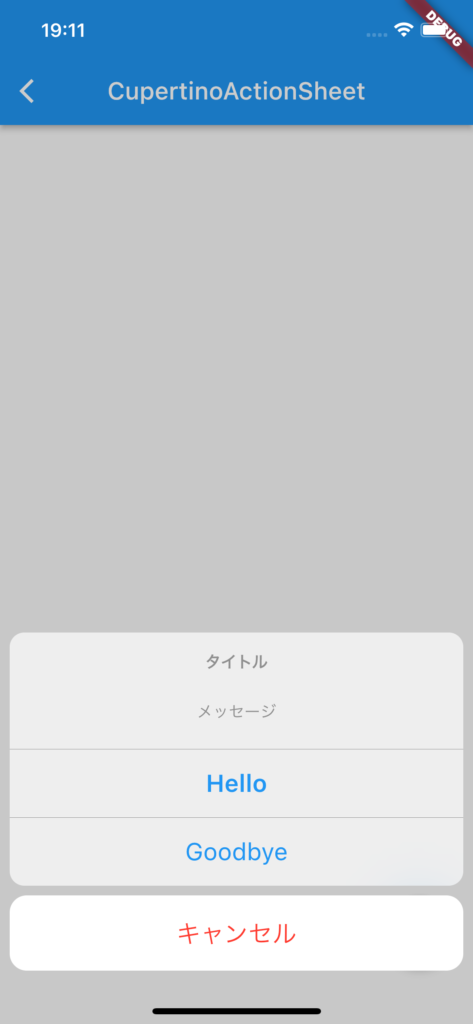
動画
公式リファレンス
CupertinoActionSheet class - cupertino library - Dart API
API docs for the CupertinoActionSheet class from the cupertino library, for the Dart programming language.
さいごに
今回のウィジェットはすべて個人開発でも使えそうですね。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント