はじめに
前回はFlutter Widget of the Weekの「#49 Stack」、「#50 AnimatedOpacity」、「#51 FractionallySizedBox」を紹介しました。
今回はその続きで「#52 ListView」、「#53 ListTile」、「#54 Container」の3つです。
前回の記事はこちら
Flutter Widget of the Week
環境
- Flutter 3.0.5
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#52 ListView
ListViewとは
スクロール可能なリストで項目を一覧表示したい場合に利用するウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage052 extends StatefulWidget {
const SamplePage052({
super.key,
});
@override
State<SamplePage052> createState() => _SamplePage052State();
}
class _SamplePage052State extends State<SamplePage052> {
bool _isVertical = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ListView'),
centerTitle: true,
),
body: SafeArea(
child: ListView.builder(
scrollDirection: _isVertical ? Axis.vertical : Axis.horizontal,
itemCount: 20,
itemBuilder: (context, index) {
return Container(
margin: const EdgeInsets.all(5),
padding: const EdgeInsets.all(20),
color: Colors.orange,
child: Center(
child: Text(
index.toString(),
style: const TextStyle(
color: Colors.white,
),
),
),
);
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_isVertical = !_isVertical;
});
},
child: const Icon(Icons.refresh),
),
);
}
}
結果
FloatingActionButtonでスクロールの方向を変化させています。
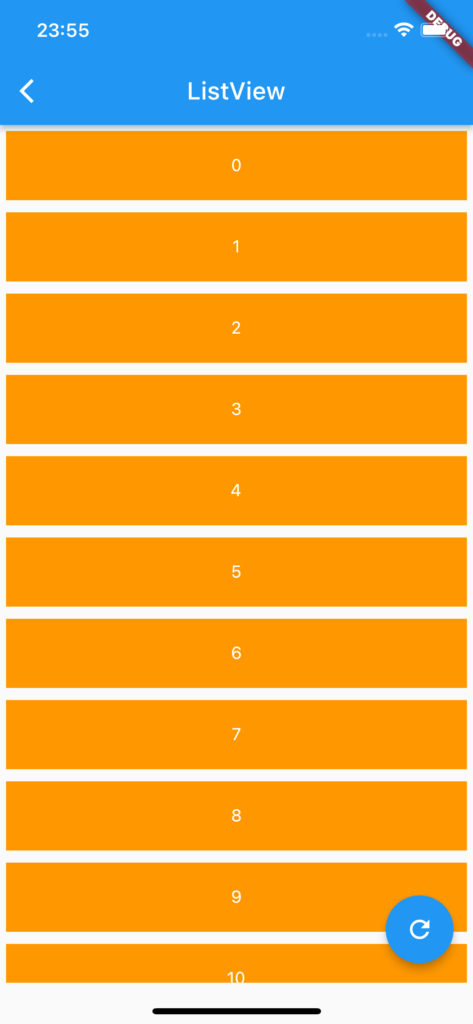
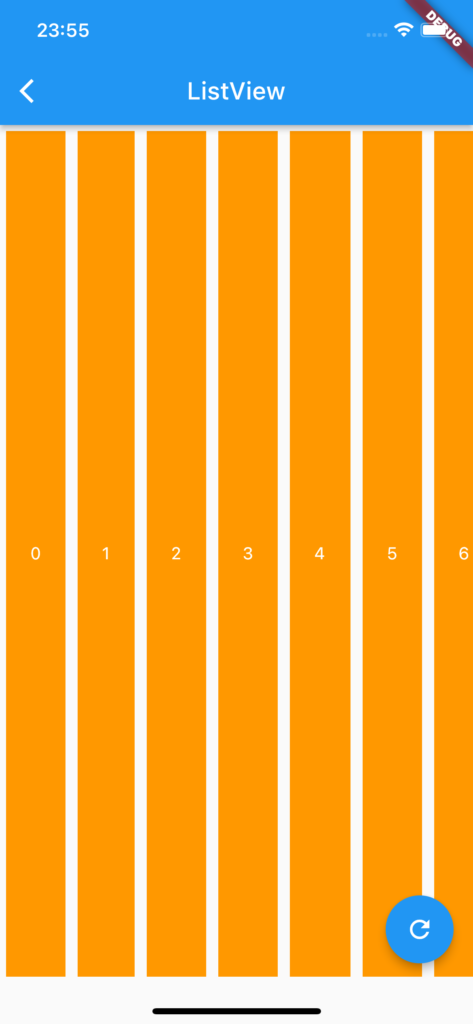
動画
公式レファレンス
ListView class - widgets library - Dart API
API docs for the ListView class from the widgets library, for the Dart programming language.
#53 ListTile
ListTileとは
マテリアルデザインの仕様にあわせたリストの項目を使いたい場合に利用するウィジェットです。
多くの場合上記のListViewと組み合わせて使うことが多いです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage053 extends StatelessWidget {
const SamplePage053({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ListTile'),
centerTitle: true,
),
body: SafeArea(
child: ListView.builder(
itemCount: 20,
itemBuilder: (context, index) {
return ListTile(
leading: const CircleAvatar(
child: Icon(Icons.person),
),
title: const Text('Widget of the week'),
subtitle: Text('#$index ...'),
trailing: const Icon(Icons.drag_handle),
);
},
),
),
);
}
}
結果
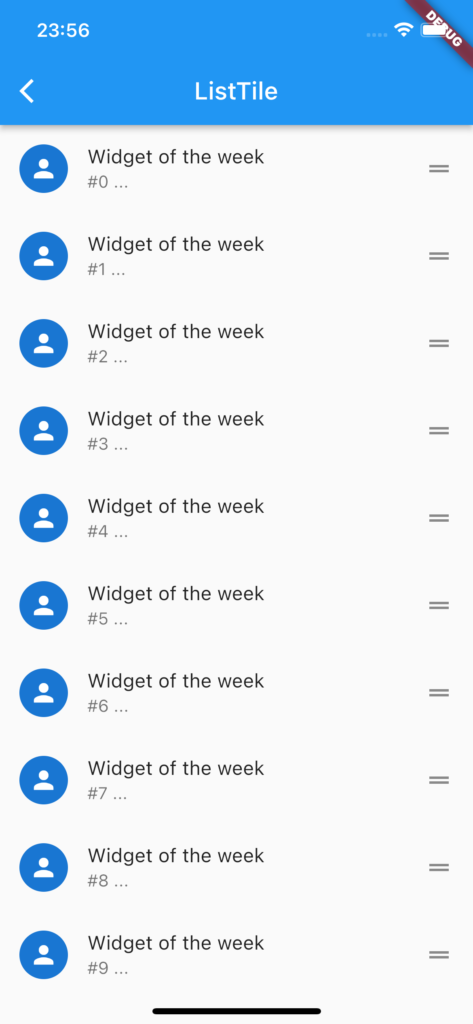
動画
公式レファレンス
ListTile class - material library - Dart API
API docs for the ListTile class from the material library, for the Dart programming language.
#54 Container
Containerとは
このウィジェットは子ウィジェットの構成や装飾、配置を調整するのに幅広く使えるウィジェットです。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage054 extends StatelessWidget {
const SamplePage054({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Container'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Container(
decoration: const BoxDecoration(
shape: BoxShape.circle,
color: Colors.blue,
),
margin: const EdgeInsets.all(25),
padding: const EdgeInsets.all(40),
child: const Text('Less boring'),
),
),
),
);
}
}
結果
背景を円形にしたウィジェット
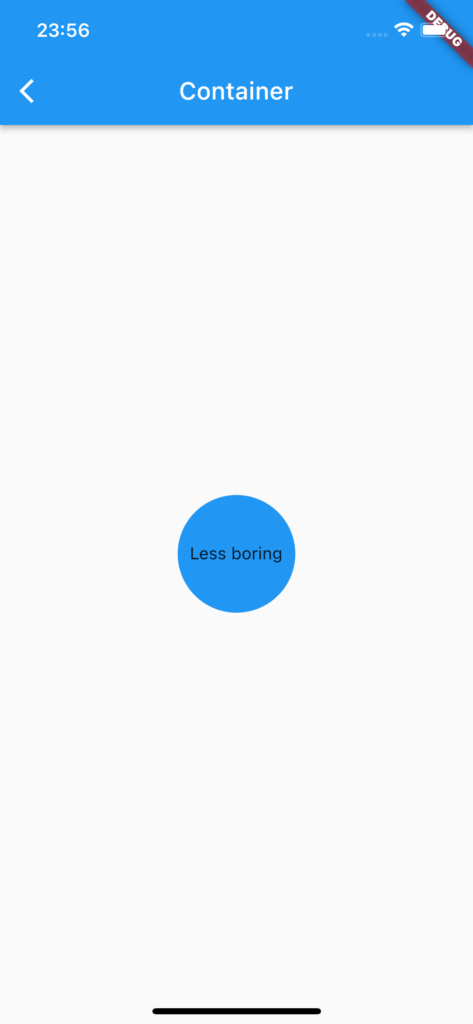
動画
公式レファレンス
Container class - widgets library - Dart API
API docs for the Container class from the widgets library, for the Dart programming language.
さいごに
ListView、ListTile、Containerは絶対使うので確実に覚えておきましょう。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント