はじめに
前回はFlutter Widget of the Weekの「#88 GridView」、「#89 SwitchListTile」、「#90 Location」を紹介しました。
今回はその続きで「#91 device_info_plus」、「#92 ImageFiltered」、「#93 PhysicalModel」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.3.4
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#91 device_info_plus
device_info_plusとは
OSのバージョンやモデル情報を知りたい場合に使うプラグインです。
ただし動画紹介している「device_info」は既に更新が終了されており、後継の「device_info_plus」を利用する必要があります。
サンプルコード
import 'dart:io';
import 'package:device_info_plus/device_info_plus.dart';
import 'package:flutter/material.dart';
class SamplePage091 extends StatelessWidget {
const SamplePage091({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('device_info_plus'),
centerTitle: true,
),
body: SafeArea(
child: Platform.isIOS
? FutureBuilder<IosDeviceInfo>(
future: DeviceInfoPlugin().iosInfo,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return const Center(
child: CircularProgressIndicator(),
);
}
if (snapshot.hasError) {
return const Center(
child: Icon(
Icons.error,
color: Colors.red,
),
);
}
final info = snapshot.data!;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
Text('model : ${info.model}'),
Text('systemVersion : ${info.systemVersion}'),
Text('utsname.machine : ${info.utsname.machine}'),
],
),
);
},
)
: FutureBuilder<AndroidDeviceInfo>(
future: DeviceInfoPlugin().androidInfo,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return const Center(
child: CircularProgressIndicator(),
);
}
if (snapshot.hasError) {
return const Center(
child: Icon(
Icons.error,
color: Colors.red,
),
);
}
final info = snapshot.data!;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
Text('device : ${info.device}'),
Text('systemVersion : ${info.version.release}'),
Text('manufacturer : ${info.manufacturer}'),
],
),
);
},
),
),
);
}
}
結果
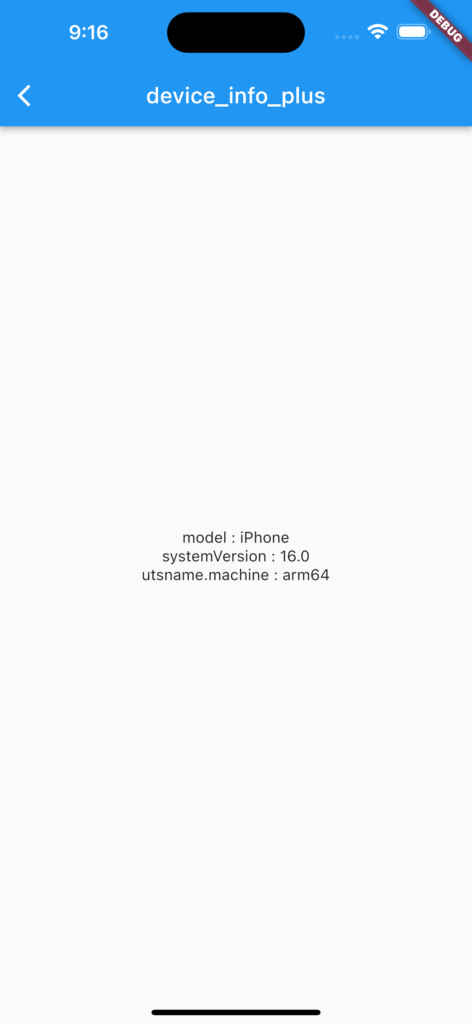
動画
公式リファレンス
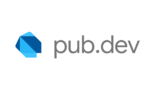
device_info_plus | Flutter Package
Flutter plugin providing detailed information about the device (make, model, etc.), and Android or iOS version the app i...
#92 ImageFiltered
ImageFilteredとは
ImageFilterは低レベルクラスで画像を処理することができます。例えばブラーやサイズ変更、移動、スキュー、回転などです。
そんなImageFilterをウィジェット簡単に扱えるのがこのImageFilteredウィジェットです。
サンプルコード
import 'dart:ui';
import 'package:flutter/material.dart';
class SamplePage092 extends StatelessWidget {
const SamplePage092({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ImageFiltered'),
centerTitle: true,
),
body: SafeArea(
child: ListView(
children: [
_SamplePage092Child(),
ImageFiltered(
imageFilter: ImageFilter.blur(
sigmaX: 2,
sigmaY: 2,
),
child: _SamplePage092Child(),
),
ImageFiltered(
imageFilter: ImageFilter.dilate(
radiusX: 0.2,
radiusY: 0.2,
),
child: _SamplePage092Child(),
),
ImageFiltered(
imageFilter: ImageFilter.erode(
radiusX: 0.2,
radiusY: 0.2,
),
child: _SamplePage092Child(),
),
ImageFiltered(
imageFilter: ImageFilter.matrix(
Matrix4.rotationZ(0.2).storage,
),
child: _SamplePage092Child(),
),
const SizedBox(height: 64),
ImageFiltered(
imageFilter: ImageFilter.compose(
inner: ImageFilter.dilate(
radiusX: 0.3,
radiusY: 0.3,
),
outer: ImageFilter.erode(
radiusX: 0.3,
radiusY: 0.3,
),
),
child: _SamplePage092Child(),
),
],
),
),
);
}
}
class _SamplePage092Child extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Image.asset(
'assets/genba_neko.png',
width: 128,
height: 128,
);
}
}
結果
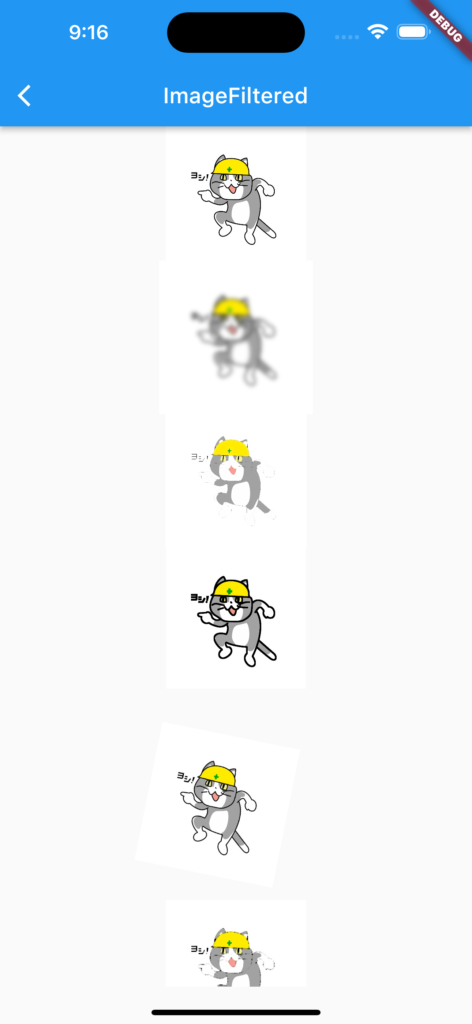
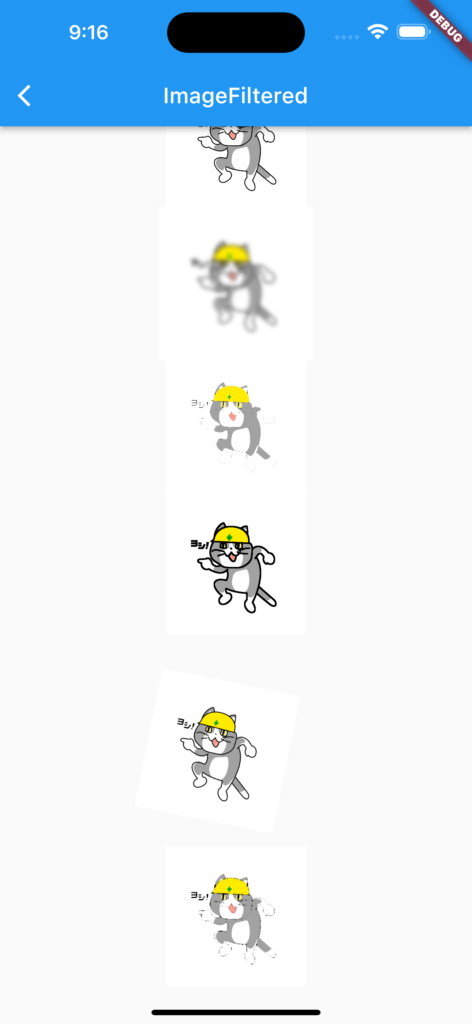
動画
公式リファレンス
ImageFiltered class - widgets library - Dart API
API docs for the ImageFiltered class from the widgets library, for the Dart programming language.
#93 PhysicalModel
PhysicalModelとは
ウィジェットをリアルに感じさせるための陰影をつけることができるウィジェットです。
またPhysicalModelは子ウィジェットではなく、下に作られるレイヤーに描写された物体に陰影が付くので子ウィジェットが透明のもの使ってしまうと見えてしまいます。
サンプルコード
import 'package:flutter/material.dart';
class SamplePage093 extends StatelessWidget {
const SamplePage093({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('PhysicalModel'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
PhysicalModel(
color: Colors.black,
elevation: 20,
child: Container(
color: Colors.blue,
width: 128,
height: 128,
),
),
const SizedBox(height: 64),
const PhysicalModel(
color: Colors.red,
shadowColor: Colors.red,
shape: BoxShape.circle,
elevation: 10,
child: FlutterLogo(
size: 128,
),
),
],
),
),
),
);
}
}
結果
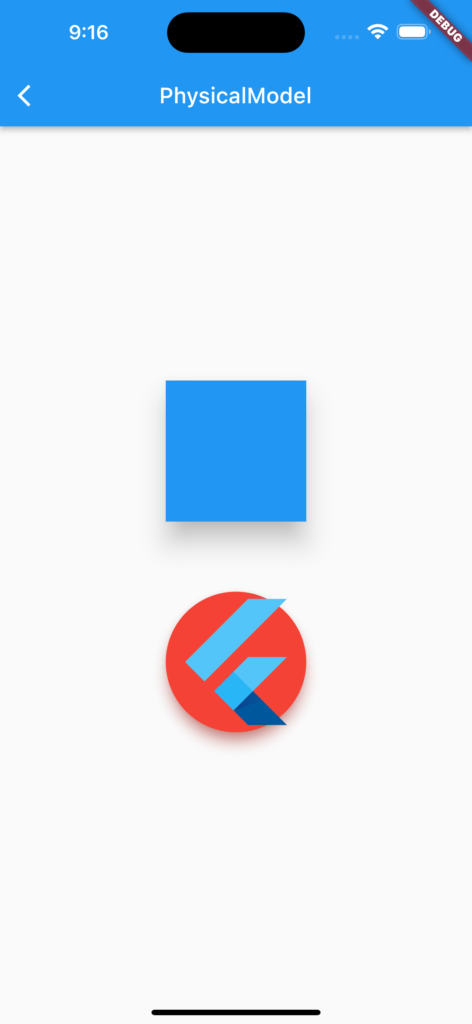
動画
公式リファレンス
PhysicalModel class - widgets library - Dart API
API docs for the PhysicalModel class from the widgets library, for the Dart programming language.
さいごに
このシリーズ、終りが見えない。。。
そして追いつく前にYouTubeのFlutter Widget of the Weekが終わってしまい、悲しみ。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント