はじめに
前回はFlutter Widget of the Weekの「#139 firebase_remote_config」、「#140 flame」、「#141 RawMagnifier」を紹介しました。
今回はその続きで「#142 flutter_lints」、「#143 firebase_storage」、「#144 flutter_animate」の3つです。
前回の記事はこちら
またGitHubにも公開しています。
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
Flutter Widget of the Week
環境
- Flutter 3.19.6
記事にした時点でのバージョンです。GitHubに公開しているのは常に最新の安定版(stable)を使う予定です。
#142 flutter_lints
flutter_lintsとは
いわゆる静的解析ができるパッケージです。書き方がまずいコード等を教えてくれるようになります。
さらにこのパッケージはFlutterプロジェクトを作成するとデフォルトで入っているため、自分で追加する必要がありません。
サンプルコード
- analysis_options.yaml
# The following line activates a set of recommended lints for Flutter apps,
# packages, and plugins designed to encourage good coding practices.
include: package:flutter_lints/flutter.yaml
linter:
# The lint rules applied to this project can be customized in the
サンプルコードというよりはここで設定されていますよという紹介です。
このファイルに好きなルール等も追加できます。
動画
公式リファレンス
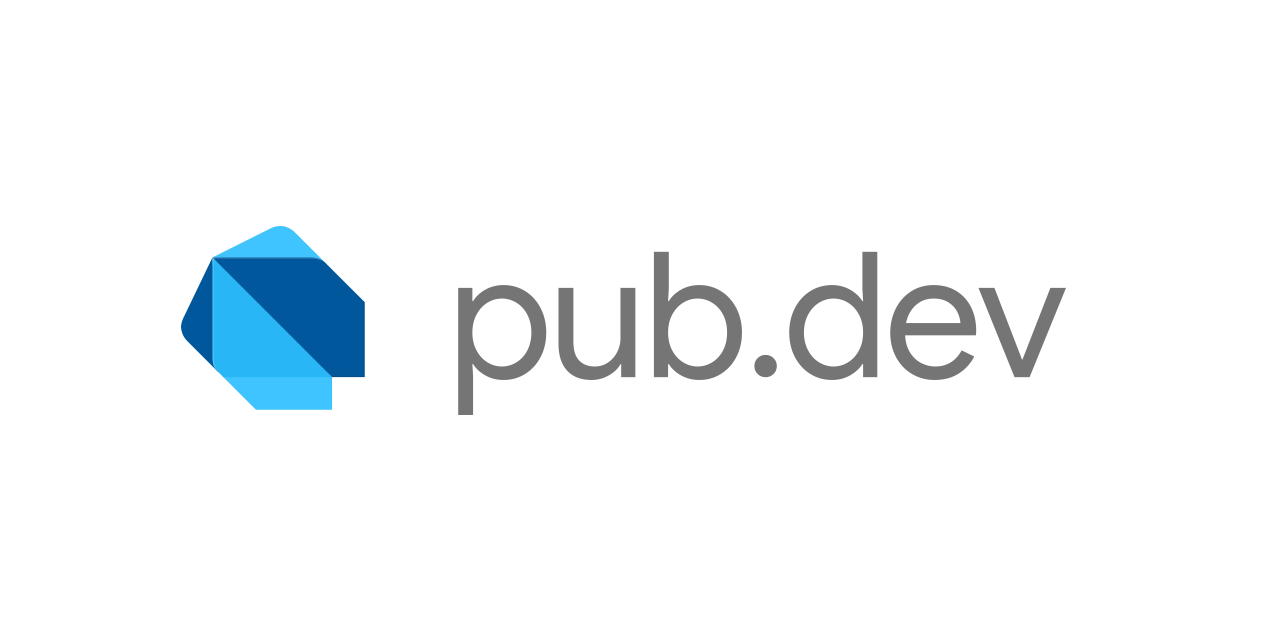
flutter_lints | Dart package
Recommended lints for Flutter apps, packages, and plugins to encourage good coding practices.
#143 firebase_storage
firebase_storageとは
firebase_storageはFirebase Cloud Storage APIを使用するためのFlutterプラグインです。
Firebase Cloud Storageはクラウドストレージのサービスで、写真等のファイルをアップロードやダウンロードが実現できます。
サンプルコード
import 'dart:io';
import 'package:firebase_storage/firebase_storage.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:path_provider/path_provider.dart';
class SamplePage143 extends StatefulWidget {
const SamplePage143({
super.key,
});
@override
State<SamplePage143> createState() => _SamplePage141State();
}
class _SamplePage141State extends State<SamplePage143> {
bool uploaded = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('firebase_storage'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
children: [
ElevatedButton(
onPressed: () async {
final storage = FirebaseStorage.instance.ref();
final images = storage.child('images');
final sampleImageRef = images.child('sample.png');
final byteData = await rootBundle.load('assets/sample.png');
final file = File(
'${(await getTemporaryDirectory()).path}/sample.png',
);
await file.create(recursive: true);
await file.writeAsBytes(
byteData.buffer.asUint8List(
byteData.offsetInBytes,
byteData.lengthInBytes,
),
);
await sampleImageRef.putFile(file);
setState(() {
uploaded = true;
});
},
child: const Text('Upload Images'),
),
const SizedBox(height: 20),
if (uploaded)
FutureBuilder(
initialData: '',
future: FirebaseStorage.instance
.ref()
.child('images')
.child('sample.png')
.getDownloadURL(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return const CircularProgressIndicator();
}
if (snapshot.hasError) {
return const Icon(Icons.error);
}
return Image.network(snapshot.data!);
},
),
],
),
),
),
);
}
}
結果
試しにassetsフォルダの画像ファイルをアップロードし、Image.networkを利用して表示してみます。
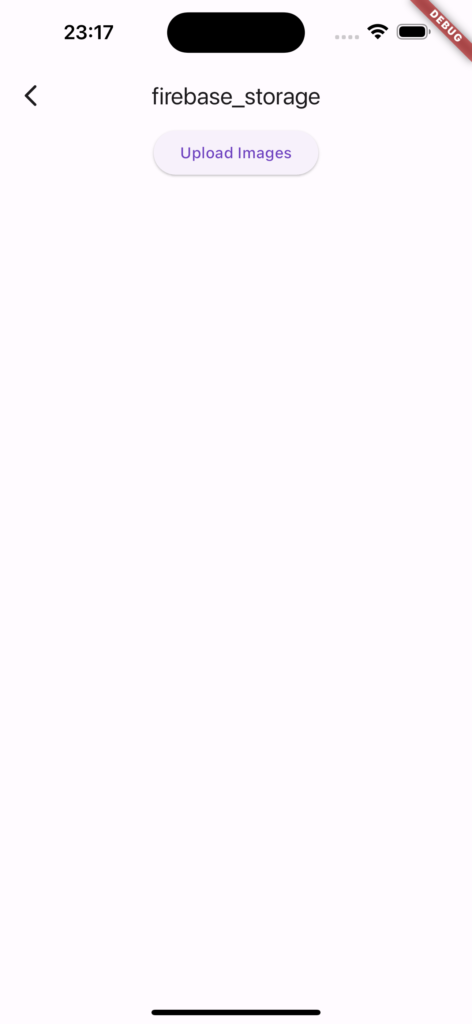
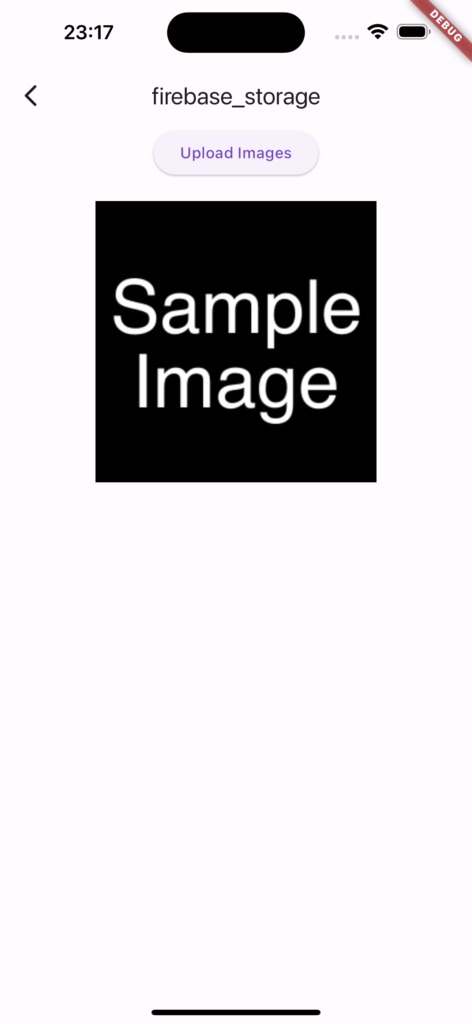
Firebase Cloud Storageを見るとちゃんとアップロードされていることが確認できます
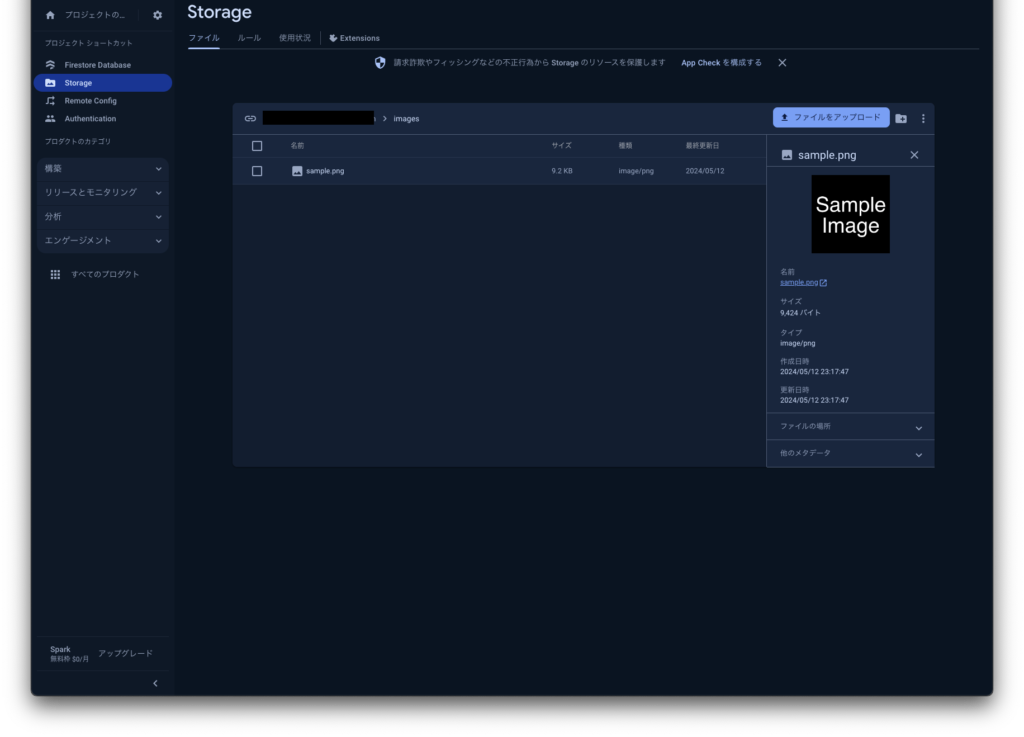
動画
公式リファレンス
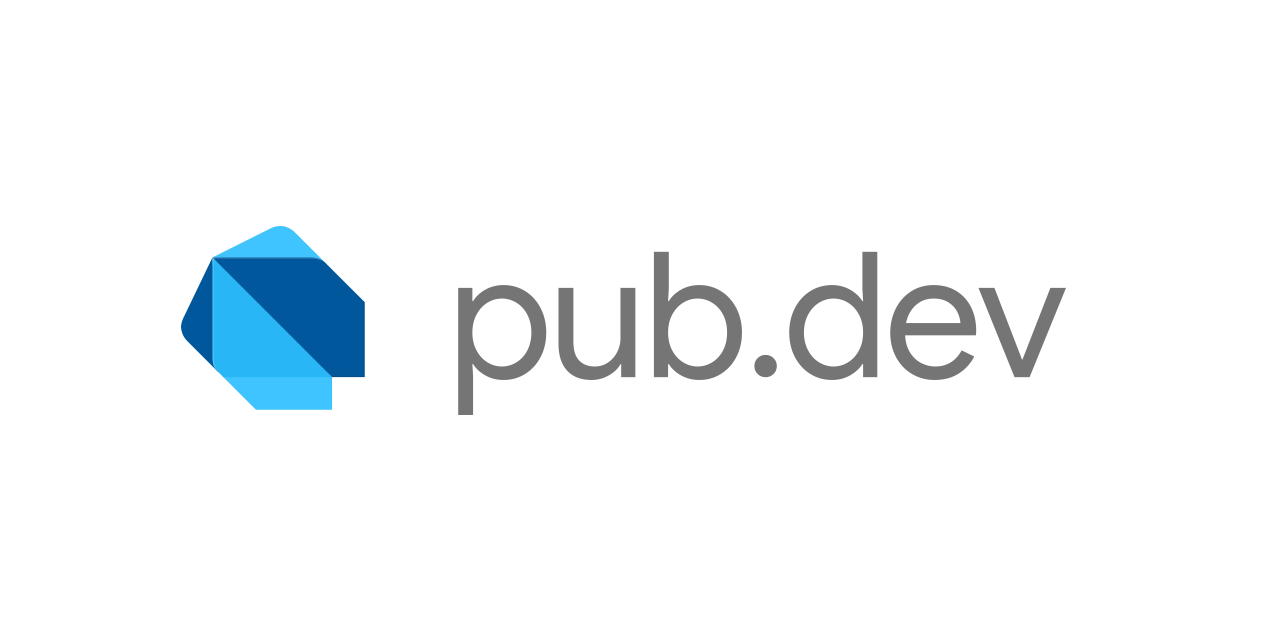
firebase_storage | Flutter package
Flutter plugin for Firebase Cloud Storage, a powerful, simple, and cost-effective object storage service for Android and...
#144 flutter_animate
flutter_animateとは
AnimationControllerなしでWidgetを簡単にアニメーションさせることができるパッケージです。
サンプルコード
import 'package:flutter/material.dart';
import 'package:flutter_animate/flutter_animate.dart';
class SamplePage144 extends StatefulWidget {
const SamplePage144({
super.key,
});
@override
State<SamplePage144> createState() => _SamplePage144State();
}
class _SamplePage144State extends State<SamplePage144> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('flutter_animate'),
centerTitle: true,
),
body: SafeArea(
child: Center(
child: Column(
children: [
const Text('Hello World!').animate().fade().scale(),
const SizedBox(height: 20),
Column(
children: [
const Text('Hello'),
const Text('World'),
const Text('Goodbye'),
].animate(interval: 400.ms).fade(duration: 300.ms),
),
const SizedBox(height: 20),
const Text('Before').animate().swap(
duration: 900.ms,
builder: (_, __) => const Text('After'),
),
],
),
),
),
);
}
}
結果
動画
公式リファレンス
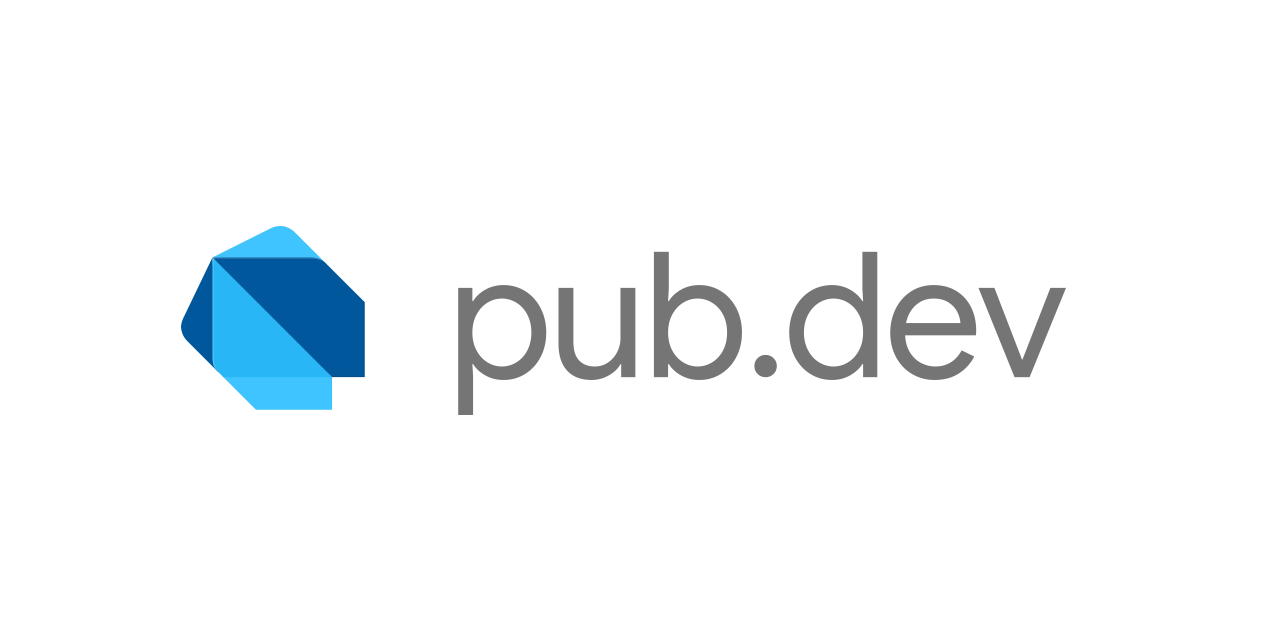
flutter_animate | Flutter package
Add beautiful animated effects & builders in Flutter, via an easy, customizable, unified API.
さいごに
サボってしまった。。。
おすすめ参考書
リンク
GitHub - nobushiueshi/flutter_widget_of_the_week
Contribute to nobushiueshi/flutter_widget_of_the_week development by creating an account on GitHub.
コメント